

Python Conditional Assignment
When you want to assign a value to a variable based on some condition, like if the condition is true then assign a value to the variable, else assign some other value to the variable, then you can use the conditional assignment operator.
In this tutorial, we will look at different ways to assign values to a variable based on some condition.
1. Using Ternary Operator
The ternary operator is very special operator in Python, it is used to assign a value to a variable based on some condition.
It goes like this:
Here, the value of variable will be value_if_true if the condition is true, else it will be value_if_false .
Let's see a code snippet to understand it better.
You can see we have conditionally assigned a value to variable c based on the condition a > b .
2. Using if-else statement
if-else statements are the core part of any programming language, they are used to execute a block of code based on some condition.
Using an if-else statement, we can assign a value to a variable based on the condition we provide.
Here is an example of replacing the above code snippet with the if-else statement.
3. Using Logical Short Circuit Evaluation
Logical short circuit evaluation is another way using which you can assign a value to a variable conditionally.
The format of logical short circuit evaluation is:
It looks similar to ternary operator, but it is not. Here the condition and value_if_true performs logical AND operation, if both are true then the value of variable will be value_if_true , or else it will be value_if_false .
Let's see an example:
But if we make condition True but value_if_true False (or 0 or None), then the value of variable will be value_if_false .
So, you can see that the value of c is 20 even though the condition a < b is True .
So, you should be careful while using logical short circuit evaluation.
While working with lists , we often need to check if a list is empty or not, and if it is empty then we need to assign some default value to it.
Let's see how we can do it using conditional assignment.
Here, we have assigned a default value to my_list if it is empty.
Assign a value to a variable conditionally based on the presence of an element in a list.
Now you know 3 different ways to assign a value to a variable conditionally. Any of these methods can be used to assign a value when there is a condition.
The cleanest and fastest way to conditional value assignment is the ternary operator .
if-else statement is recommended to use when you have to execute a block of code based on some condition.
Happy coding! š
Python One Line Conditional Assignment
Problem : How to perform one-line if conditional assignments in Python?
Example : Say, you start with the following code.
You want to set the value of x to 42 if boo is True , and do nothing otherwise.
Let’s dive into the different ways to accomplish this in Python. We start with an overview:
Exercise : Run the code. Are all outputs the same?
Next, you’ll dive into each of those methods and boost your one-liner superpower !
Method 1: Ternary Operator
The most basic ternary operator x if c else y returns expression x if the Boolean expression c evaluates to True . Otherwise, if the expression c evaluates to False , the ternary operator returns the alternative expression y .
Operand | Description |
---|---|
<OnTrue> | The return expression of the operator in case the condition evaluates to |
<Condition> | The condition that determines whether to return the <On True> or the <On False> branch. |
<OnFalse> | The return expression of the operator in case the condition evaluates to |
Let’s go back to our example problem! You want to set the value of x to 42 if boo is True , and do nothing otherwise. Here’s how to do this in a single line:
While using the ternary operator works, you may wonder whether it’s possible to avoid the ...else x part for clarity of the code? In the next method, you’ll learn how!
If you need to improve your understanding of the ternary operator, watch the following video:
You can also read the related article:
- Python One Line Ternary
Method 2: Single-Line If Statement
Like in the previous method, you want to set the value of x to 42 if boo is True , and do nothing otherwise. But you don’t want to have a redundant else branch. How to do this in Python?
The solution to skip the else part of the ternary operator is surprisingly simple— use a standard if statement without else branch and write it into a single line of code :
To learn more about what you can pack into a single line, watch my tutorial video “If-Then-Else in One Line Python” :
Method 3: Ternary Tuple Syntax Hack
A shorthand form of the ternary operator is the following tuple syntax .
Syntax : You can use the tuple syntax (x, y)[c] consisting of a tuple (x, y) and a condition c enclosed in a square bracket. Here’s a more intuitive way to represent this tuple syntax.
In fact, the order of the <OnFalse> and <OnTrue> operands is just flipped when compared to the basic ternary operator. First, you have the branch that’s returned if the condition does NOT hold. Second, you run the branch that’s returned if the condition holds.
Clever! The condition boo holds so the return value passed into the x variable is the <OnTrue> branch 42 .
Don’t worry if this confuses you—you’re not alone. You can clarify the tuple syntax once and for all by studying my detailed blog article.
Related Article : Python Ternary — Tuple Syntax Hack
Python One-Liners Book: Master the Single Line First!
Python programmers will improve their computer science skills with these useful one-liners.
Python One-Liners will teach you how to read and write “one-liners”: concise statements of useful functionality packed into a single line of code. You’ll learn how to systematically unpack and understand any line of Python code, and write eloquent, powerfully compressed Python like an expert.
The book’s five chapters cover (1) tips and tricks, (2) regular expressions, (3) machine learning, (4) core data science topics, and (5) useful algorithms.
Detailed explanations of one-liners introduce key computer science concepts and boost your coding and analytical skills . You’ll learn about advanced Python features such as list comprehension , slicing , lambda functions , regular expressions , map and reduce functions, and slice assignments .
You’ll also learn how to:
- Leverage data structures to solve real-world problems , like using Boolean indexing to find cities with above-average pollution
- Use NumPy basics such as array , shape , axis , type , broadcasting , advanced indexing , slicing , sorting , searching , aggregating , and statistics
- Calculate basic statistics of multidimensional data arrays and the K-Means algorithms for unsupervised learning
- Create more advanced regular expressions using grouping and named groups , negative lookaheads , escaped characters , whitespaces, character sets (and negative characters sets ), and greedy/nongreedy operators
- Understand a wide range of computer science topics , including anagrams , palindromes , supersets , permutations , factorials , prime numbers , Fibonacci numbers, obfuscation , searching , and algorithmic sorting
By the end of the book, you’ll know how to write Python at its most refined , and create concise, beautiful pieces of “Python art” in merely a single line.
Get your Python One-Liners on Amazon!!
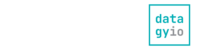
- Learn Python
- Python Lists
- Python Dictionaries
- Python Strings
- Python Functions
- Learn Pandas & NumPy
- Pandas Tutorials
- Numpy Tutorials
- Learn Data Visualization
- Python Seaborn
- Python Matplotlib
Set Pandas Conditional Column Based on Values of Another Column
- August 9, 2021 February 22, 2022
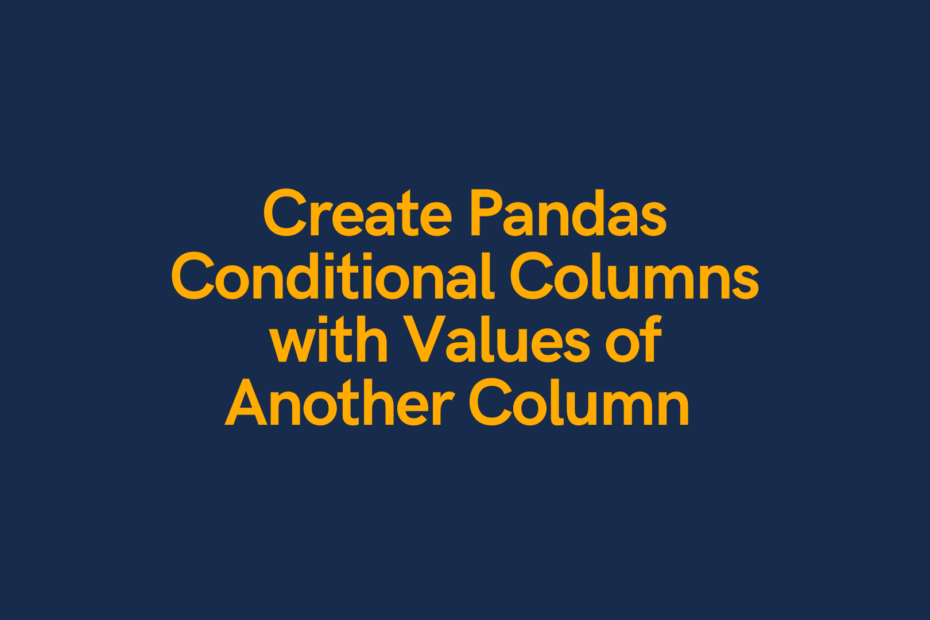
There are many times when you may need to set a Pandas column value based on the condition of another column. In this post, you’ll learn all the different ways in which you can create Pandas conditional columns.
Table of Contents
Video Tutorial
If you prefer to follow along with a video tutorial, check out my video below:
Loading a Sample Dataframe
Let’s begin by loading a sample Pandas dataframe that we can use throughout this tutorial.
We’ll begin by import pandas and loading a dataframe using the .from_dict() method:
This returns the following dataframe:
Using Pandas loc to Set Pandas Conditional Column
Pandas loc is incredibly powerful! If you need a refresher on loc (or iloc), check out my tutorial here . Pandas’ loc creates a boolean mask, based on a condition. Sometimes, that condition can just be selecting rows and columns, but it can also be used to filter dataframes. These filtered dataframes can then have values applied to them.
Let’s explore the syntax a little bit:
With the syntax above, we filter the dataframe using .loc and then assign a value to any row in the column (or columns) where the condition is met.
Let’s try this out by assigning the string ‘Under 30’ to anyone with an age less than 30, and ‘Over 30’ to anyone 30 or older.
Let's take a look at what we did here:
- We assigned the string 'Over 30' to every record in the dataframe. To learn more about this, check out my post here or creating new columns.
- We then use .loc to create a boolean mask on the Age column to filter down to rows where the age is less than 30. When this condition is met, the Age Category column is assigned the new value 'Under 30'
But what happens when you have multiple conditions? You could, of course, use .loc multiple times, but this is difficult to read and fairly unpleasant to write. Let's see how we can accomplish this using numpy's .select() method.
Using Numpy Select to Set Values using Multiple Conditions
Similar to the method above to use .loc to create a conditional column in Pandas, we can use the numpy .select() method.
Let's begin by importing numpy and we'll give it the conventional alias np :
Now, say we wanted to apply a number of different age groups, as below:
- <20 years old,
- 20-39 years old,
- 40-59 years old,
- 60+ years old
In order to do this, we'll create a list of conditions and corresponding values to fill:
Running this returns the following dataframe:
Let's break down what happens here:
- We first define a list of conditions in which the criteria are specified. Recall that lists are ordered meaning that they should be in the order in which you would like the corresponding values to appear.
- We then define a list of values to use , which corresponds to the values you'd like applied in your new column.
Something to consider here is that this can be a bit counterintuitive to write. You can similarly define a function to apply different values. We'll cover this off in the section of using the Pandas .apply() method below .
One of the key benefits is that using numpy as is very fast, especially when compared to using the .apply() method.
Using Pandas Map to Set Values in Another Column
The Pandas .map() method is very helpful when you're applying labels to another column. In order to use this method, you define a dictionary to apply to the column.
For our sample dataframe, let's imagine that we have offices in America, Canada, and France. We want to map the cities to their corresponding countries and apply and "Other" value for any other city.
When we print this out, we get the following dataframe returned:
What we can see here, is that there is a NaN value associated with any City that doesn't have a corresponding country. If we want to apply "Other" to any missing values, we can chain the .fillna() method:
Using Pandas Apply to Apply a function to a column
Finally, you can apply built-in or custom functions to a dataframe using the Pandas .apply() method.
Let's take a look at both applying built-in functions such as len() and even applying custom functions.
Applying Python Built-in Functions to a Column
We can easily apply a built-in function using the .apply() method. Let's see how we can use the len() function to count how long a string of a given column.
Take note of a few things here:
- We apply the .apply() method to a particular column,
- We omit the parentheses "()"
Using Third-Party Packages in Pandas Apply
Similarly, you can use functions from using packages. Let's use numpy to apply the .sqrt() method to find the scare root of a person's age.
Using Custom Functions with Pandas Apply
Something that makes the .apply() method extremely powerful is the ability to define and apply your own functions.
Let's revisit how we could use an if-else statement to create age categories as in our earlier example:
In this post, you learned a number of ways in which you can apply values to a dataframe column to create a Pandas conditional column, including using .loc , .np.select() , Pandas .map() and Pandas .apply() . Each of these methods has a different use case that we explored throughout this post.
Learn more about Pandas methods covered here by checking out their official documentation:
- Pandas Apply
- Numpy Select
Nik Piepenbreier
Nik is the author of datagy.io and has over a decade of experience working with data analytics, data science, and Python. He specializes in teaching developers how to use Python for data science using hands-on tutorials. View Author posts
2 thoughts on “Set Pandas Conditional Column Based on Values of Another Column”
Thank you so much! Brilliantly explained!!!
Thanks Aisha!
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
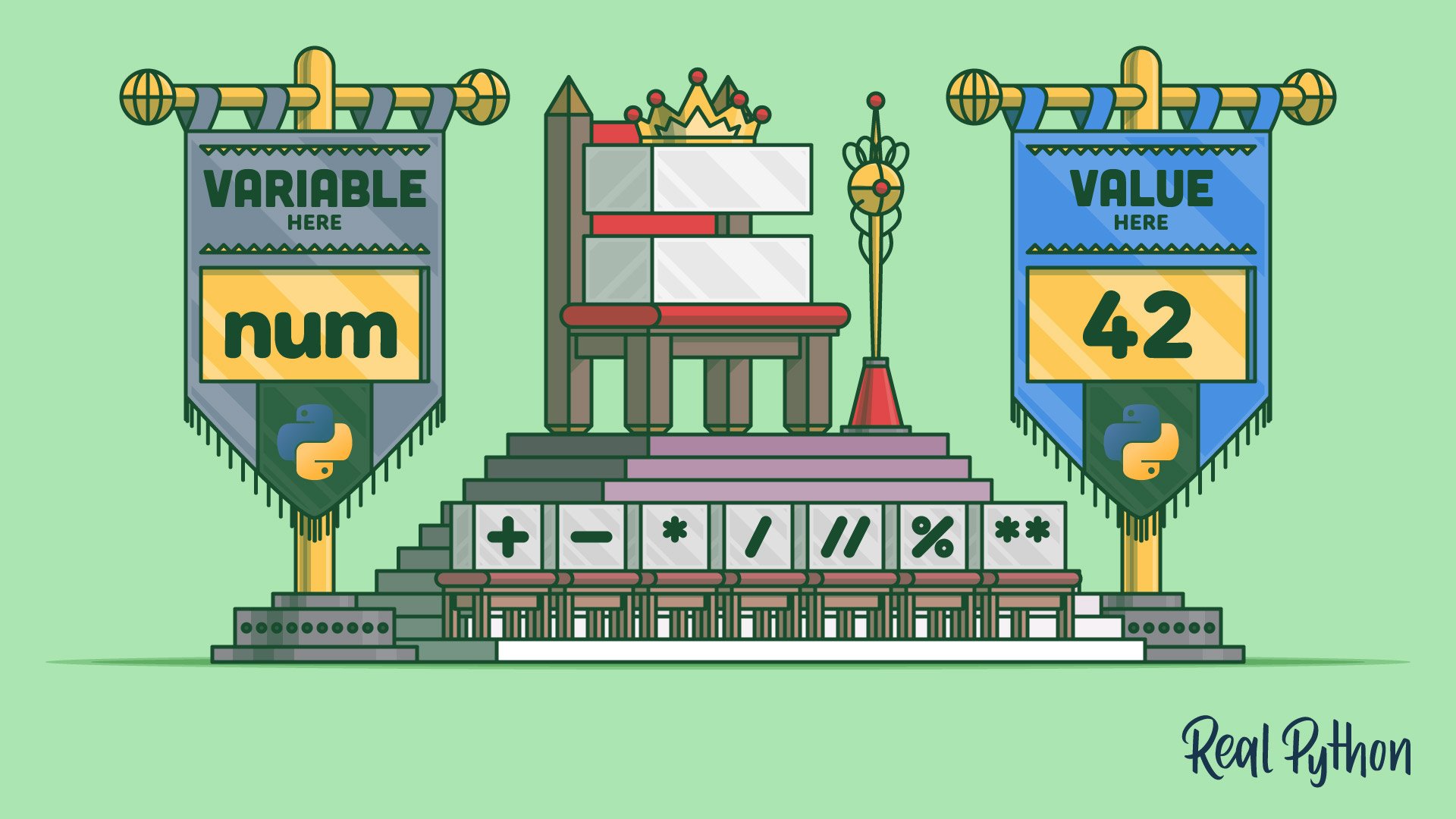
Python's Assignment Operator: Write Robust Assignments
Table of Contents
The Assignment Statement Syntax
The assignment operator, assignments and variables, other assignment syntax, initializing and updating variables, making multiple variables refer to the same object, updating lists through indices and slices, adding and updating dictionary keys, doing parallel assignments, unpacking iterables, providing default argument values, augmented mathematical assignment operators, augmented assignments for concatenation and repetition, augmented bitwise assignment operators, annotated assignment statements, assignment expressions with the walrus operator, managed attribute assignments, define or call a function, work with classes, import modules and objects, use a decorator, access the control variable in a for loop or a comprehension, use the as keyword, access the _ special variable in an interactive session, built-in objects, named constants.
Python’s assignment operators allow you to define assignment statements . This type of statement lets you create, initialize, and update variables throughout your code. Variables are a fundamental cornerstone in every piece of code, and assignment statements give you complete control over variable creation and mutation.
Learning about the Python assignment operator and its use for writing assignment statements will arm you with powerful tools for writing better and more robust Python code.
In this tutorial, you’ll:
- Use Python’s assignment operator to write assignment statements
- Take advantage of augmented assignments in Python
- Explore assignment variants, like assignment expressions and managed attributes
- Become aware of illegal and dangerous assignments in Python
You’ll dive deep into Python’s assignment statements. To get the most out of this tutorial, you should be comfortable with several basic topics, including variables , built-in data types , comprehensions , functions , and Python keywords . Before diving into some of the later sections, you should also be familiar with intermediate topics, such as object-oriented programming , constants , imports , type hints , properties , descriptors , and decorators .
Free Source Code: Click here to download the free assignment operator source code that you’ll use to write assignment statements that allow you to create, initialize, and update variables in your code.
Assignment Statements and the Assignment Operator
One of the most powerful programming language features is the ability to create, access, and mutate variables . In Python, a variable is a name that refers to a concrete value or object, allowing you to reuse that value or object throughout your code.
To create a new variable or to update the value of an existing one in Python, you’ll use an assignment statement . This statement has the following three components:
- A left operand, which must be a variable
- The assignment operator ( = )
- A right operand, which can be a concrete value , an object , or an expression
Here’s how an assignment statement will generally look in Python:
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal —or an expression that evaluates to a value.
To execute an assignment statement like the above, Python runs the following steps:
- Evaluate the right-hand expression to produce a concrete value or object . This value will live at a specific memory address in your computer.
- Store the object’s memory address in the left-hand variable . This step creates a new variable if the current one doesn’t already exist or updates the value of an existing variable.
The second step shows that variables work differently in Python than in other programming languages. In Python, variables aren’t containers for objects. Python variables point to a value or object through its memory address. They store memory addresses rather than objects.
This behavior difference directly impacts how data moves around in Python, which is always by reference . In most cases, this difference is irrelevant in your day-to-day coding, but it’s still good to know.
The central component of an assignment statement is the assignment operator . This operator is represented by the = symbol, which separates two operands:
- A value or an expression that evaluates to a concrete value
Operators are special symbols that perform mathematical , logical , and bitwise operations in a programming language. The objects (or object) on which an operator operates are called operands .
Unary operators, like the not Boolean operator, operate on a single object or operand, while binary operators act on two. That means the assignment operator is a binary operator.
Note: Like C , Python uses == for equality comparisons and = for assignments. Unlike C, Python doesn’t allow you to accidentally use the assignment operator ( = ) in an equality comparison.
Equality is a symmetrical relationship, and assignment is not. For example, the expression a == 42 is equivalent to 42 == a . In contrast, the statement a = 42 is correct and legal, while 42 = a isn’t allowed. You’ll learn more about illegal assignments later on.
The right-hand operand in an assignment statement can be any Python object, such as a number , list , string , dictionary , or even a user-defined object. It can also be an expression. In the end, expressions always evaluate to concrete objects, which is their return value.
Here are a few examples of assignments in Python:
The first two sample assignments in this code snippet use concrete values, also known as literals , to create and initialize number and greeting . The third example assigns the result of a math expression to the total variable, while the last example uses a Boolean expression.
Note: You can use the built-in id() function to inspect the memory address stored in a given variable.
Here’s a short example of how this function works:
The number in your output represents the memory address stored in number . Through this address, Python can access the content of number , which is the integer 42 in this example.
If you run this code on your computer, then you’ll get a different memory address because this value varies from execution to execution and computer to computer.
Unlike expressions, assignment statements don’t have a return value because their purpose is to make the association between the variable and its value. That’s why the Python interpreter doesn’t issue any output in the above examples.
Now that you know the basics of how to write an assignment statement, it’s time to tackle why you would want to use one.
The assignment statement is the explicit way for you to associate a name with an object in Python. You can use this statement for two main purposes:
- Creating and initializing new variables
- Updating the values of existing variables
When you use a variable name as the left operand in an assignment statement for the first time, you’re creating a new variable. At the same time, you’re initializing the variable to point to the value of the right operand.
On the other hand, when you use an existing variable in a new assignment, you’re updating or mutating the variable’s value. Strictly speaking, every new assignment will make the variable refer to a new value and stop referring to the old one. Python will garbage-collect all the values that are no longer referenced by any existing variable.
Assignment statements not only assign a value to a variable but also determine the data type of the variable at hand. This additional behavior is another important detail to consider in this kind of statement.
Because Python is a dynamically typed language, successive assignments to a given variable can change the variable’s data type. Changing the data type of a variable during a program’s execution is considered bad practice and highly discouraged. It can lead to subtle bugs that can be difficult to track down.
Unlike in math equations, in Python assignments, the left operand must be a variable rather than an expression or a value. For example, the following construct is illegal, and Python flags it as invalid syntax:
In this example, you have expressions on both sides of the = sign, and this isn’t allowed in Python code. The error message suggests that you may be confusing the equality operator with the assignment one, but that’s not the case. You’re really running an invalid assignment.
To correct this construct and convert it into a valid assignment, you’ll have to do something like the following:
In this code snippet, you first import the sqrt() function from the math module. Then you isolate the hypotenuse variable in the original equation by using the sqrt() function. Now your code works correctly.
Now you know what kind of syntax is invalid. But don’t get the idea that assignment statements are rigid and inflexible. In fact, they offer lots of room for customization, as you’ll learn next.
Python’s assignment statements are pretty flexible and versatile. You can write them in several ways, depending on your specific needs and preferences. Here’s a quick summary of the main ways to write assignments in Python:
Up to this point, you’ve mostly learned about the base assignment syntax in the above code snippet. In the following sections, you’ll learn about multiple, parallel, and augmented assignments. You’ll also learn about assignments with iterable unpacking.
Read on to see the assignment statements in action!
Assignment Statements in Action
You’ll find and use assignment statements everywhere in your Python code. They’re a fundamental part of the language, providing an explicit way to create, initialize, and mutate variables.
You can use assignment statements with plain names, like number or counter . You can also use assignments in more complicated scenarios, such as with:
- Qualified attribute names , like user.name
- Indices and slices of mutable sequences, like a_list[i] and a_list[i:j]
- Dictionary keys , like a_dict[key]
This list isn’t exhaustive. However, it gives you some idea of how flexible these statements are. You can even assign multiple values to an equal number of variables in a single line, commonly known as parallel assignment . Additionally, you can simultaneously assign the values in an iterable to a comma-separated group of variables in what’s known as an iterable unpacking operation.
In the following sections, you’ll dive deeper into all these topics and a few other exciting things that you can do with assignment statements in Python.
The most elementary use case of an assignment statement is to create a new variable and initialize it using a particular value or expression:
All these statements create new variables, assigning them initial values or expressions. For an initial value, you should always use the most sensible and least surprising value that you can think of. For example, initializing a counter to something different from 0 may be confusing and unexpected because counters almost always start having counted no objects.
Updating a variable’s current value or state is another common use case of assignment statements. In Python, assigning a new value to an existing variable doesn’t modify the variable’s current value. Instead, it causes the variable to refer to a different value. The previous value will be garbage-collected if no other variable refers to it.
Consider the following examples:
These examples run two consecutive assignments on the same variable. The first one assigns the string "Hello, World!" to a new variable named greeting .
The second assignment updates the value of greeting by reassigning it the "Hi, Pythonistas!" string. In this example, the original value of greeting —the "Hello, World!" string— is lost and garbage-collected. From this point on, you can’t access the old "Hello, World!" string.
Even though running multiple assignments on the same variable during a program’s execution is common practice, you should use this feature with caution. Changing the value of a variable can make your code difficult to read, understand, and debug. To comprehend the code fully, you’ll have to remember all the places where the variable was changed and the sequential order of those changes.
Because assignments also define the data type of their target variables, it’s also possible for your code to accidentally change the type of a given variable at runtime. A change like this can lead to breaking errors, like AttributeError exceptions. Remember that strings don’t have the same methods and attributes as lists or dictionaries, for example.
In Python, you can make several variables reference the same object in a multiple-assignment line. This can be useful when you want to initialize several similar variables using the same initial value:
In this example, you chain two assignment operators in a single line. This way, your two variables refer to the same initial value of 0 . Note how both variables hold the same memory address, so they point to the same instance of 0 .
When it comes to integer variables, Python exhibits a curious behavior. It provides a numeric interval where multiple assignments behave the same as independent assignments. Consider the following examples:
To create n and m , you use independent assignments. Therefore, they should point to different instances of the number 42 . However, both variables hold the same object, which you confirm by comparing their corresponding memory addresses.
Now check what happens when you use a greater initial value:
Now n and m hold different memory addresses, which means they point to different instances of the integer number 300 . In contrast, when you use multiple assignments, both variables refer to the same object. This tiny difference can save you small bits of memory if you frequently initialize integer variables in your code.
The implicit behavior of making independent assignments point to the same integer number is actually an optimization called interning . It consists of globally caching the most commonly used integer values in day-to-day programming.
Under the hood, Python defines a numeric interval in which interning takes place. That’s the interning interval for integer numbers. You can determine this interval using a small script like the following:
This script helps you determine the interning interval by comparing integer numbers from -10 to 500 . If you run the script from your command line, then you’ll get an output like the following:
This output means that if you use a single number between -5 and 256 to initialize several variables in independent statements, then all these variables will point to the same object, which will help you save small bits of memory in your code.
In contrast, if you use a number that falls outside of the interning interval, then your variables will point to different objects instead. Each of these objects will occupy a different memory spot.
You can use the assignment operator to mutate the value stored at a given index in a Python list. The operator also works with list slices . The syntax to write these types of assignment statements is the following:
In the first construct, expression can return any Python object, including another list. In the second construct, expression must return a series of values as a list, tuple, or any other sequence. You’ll get a TypeError if expression returns a single value.
Note: When creating slice objects, you can use up to three arguments. These arguments are start , stop , and step . They define the number that starts the slice, the number at which the slicing must stop retrieving values, and the step between values.
Here’s an example of updating an individual value in a list:
In this example, you update the value at index 2 using an assignment statement. The original number at that index was 7 , and after the assignment, the number is 3 .
Note: Using indices and the assignment operator to update a value in a tuple or a character in a string isn’t possible because tuples and strings are immutable data types in Python.
Their immutability means that you can’t change their items in place :
You can’t use the assignment operator to change individual items in tuples or strings. These data types are immutable and don’t support item assignments.
It’s important to note that you can’t add new values to a list by using indices that don’t exist in the target list:
In this example, you try to add a new value to the end of numbers by using an index that doesn’t exist. This assignment isn’t allowed because there’s no way to guarantee that new indices will be consecutive. If you ever want to add a single value to the end of a list, then use the .append() method.
If you want to update several consecutive values in a list, then you can use slicing and an assignment statement:
In the first example, you update the letters between indices 1 and 3 without including the letter at 3 . The second example updates the letters from index 3 until the end of the list. Note that this slicing appends a new value to the list because the target slice is shorter than the assigned values.
Also note that the new values were provided through a tuple, which means that this type of assignment allows you to use other types of sequences to update your target list.
The third example updates a single value using a slice where both indices are equal. In this example, the assignment inserts a new item into your target list.
In the final example, you use a step of 2 to replace alternating letters with their lowercase counterparts. This slicing starts at index 1 and runs through the whole list, stepping by two items each time.
Updating the value of an existing key or adding new key-value pairs to a dictionary is another common use case of assignment statements. To do these operations, you can use the following syntax:
The first construct helps you update the current value of an existing key, while the second construct allows you to add a new key-value pair to the dictionary.
For example, to update an existing key, you can do something like this:
In this example, you update the current inventory of oranges in your store using an assignment. The left operand is the existing dictionary key, and the right operand is the desired new value.
While you can’t add new values to a list by assignment, dictionaries do allow you to add new key-value pairs using the assignment operator. In the example below, you add a lemon key to inventory :
In this example, you successfully add a new key-value pair to your inventory with 100 units. This addition is possible because dictionaries don’t have consecutive indices but unique keys, which are safe to add by assignment.
The assignment statement does more than assign the result of a single expression to a single variable. It can also cope nicely with assigning multiple values to multiple variables simultaneously in what’s known as a parallel assignment .
Here’s the general syntax for parallel assignments in Python:
Note that the left side of the statement can be either a tuple or a list of variables. Remember that to create a tuple, you just need a series of comma-separated elements. In this case, these elements must be variables.
The right side of the statement must be a sequence or iterable of values or expressions. In any case, the number of elements in the right operand must match the number of variables on the left. Otherwise, you’ll get a ValueError exception.
In the following example, you compute the two solutions of a quadratic equation using a parallel assignment:
In this example, you first import sqrt() from the math module. Then you initialize the equation’s coefficients in a parallel assignment.
The equation’s solution is computed in another parallel assignment. The left operand contains a tuple of two variables, x1 and x2 . The right operand consists of a tuple of expressions that compute the solutions for the equation. Note how each result is assigned to each variable by position.
A classical use case of parallel assignment is to swap values between variables:
The highlighted line does the magic and swaps the values of previous_value and next_value at the same time. Note that in a programming language that doesn’t support this kind of assignment, you’d have to use a temporary variable to produce the same effect:
In this example, instead of using parallel assignment to swap values between variables, you use a new variable to temporarily store the value of previous_value to avoid losing its reference.
For a concrete example of when you’d need to swap values between variables, say you’re learning how to implement the bubble sort algorithm , and you come up with the following function:
In the highlighted line, you use a parallel assignment to swap values in place if the current value is less than the next value in the input list. To dive deeper into the bubble sort algorithm and into sorting algorithms in general, check out Sorting Algorithms in Python .
You can use assignment statements for iterable unpacking in Python. Unpacking an iterable means assigning its values to a series of variables one by one. The iterable must be the right operand in the assignment, while the variables must be the left operand.
Like in parallel assignments, the variables must come as a tuple or list. The number of variables must match the number of values in the iterable. Alternatively, you can use the unpacking operator ( * ) to grab several values in a variable if the number of variables doesn’t match the iterable length.
Here’s the general syntax for iterable unpacking in Python:
Iterable unpacking is a powerful feature that you can use all around your code. It can help you write more readable and concise code. For example, you may find yourself doing something like this:
Whenever you do something like this in your code, go ahead and replace it with a more readable iterable unpacking using a single and elegant assignment, like in the following code snippet:
The numbers list on the right side contains four values. The assignment operator unpacks these values into the four variables on the left side of the statement. The values in numbers get assigned to variables in the same order that they appear in the iterable. The assignment is done by position.
Note: Because Python sets are also iterables, you can use them in an iterable unpacking operation. However, it won’t be clear which value goes to which variable because sets are unordered data structures.
The above example shows the most common form of iterable unpacking in Python. The main condition for the example to work is that the number of variables matches the number of values in the iterable.
What if you don’t know the iterable length upfront? Will the unpacking work? It’ll work if you use the * operator to pack several values into one of your target variables.
For example, say that you want to unpack the first and second values in numbers into two different variables. Additionally, you would like to pack the rest of the values in a single variable conveniently called rest . In this case, you can use the unpacking operator like in the following code:
In this example, first and second hold the first and second values in numbers , respectively. These values are assigned by position. The * operator packs all the remaining values in the input iterable into rest .
The unpacking operator ( * ) can appear at any position in your series of target variables. However, you can only use one instance of the operator:
The iterable unpacking operator works in any position in your list of variables. Note that you can only use one unpacking operator per assignment. Using more than one unpacking operator isn’t allowed and raises a SyntaxError .
Dropping away unwanted values from the iterable is a common use case for the iterable unpacking operator. Consider the following example:
In Python, if you want to signal that a variable won’t be used, then you use an underscore ( _ ) as the variable’s name. In this example, useful holds the only value that you need to use from the input iterable. The _ variable is a placeholder that guarantees that the unpacking works correctly. You won’t use the values that end up in this disposable variable.
Note: In the example above, if your target iterable is a sequence data type, such as a list or tuple, then it’s best to access its last item directly.
To do this, you can use the -1 index:
Using -1 gives you access to the last item of any sequence data type. In contrast, if you’re dealing with iterators , then you won’t be able to use indices. That’s when the *_ syntax comes to your rescue.
The pattern used in the above example comes in handy when you have a function that returns multiple values, and you only need a few of these values in your code. The os.walk() function may provide a good example of this situation.
This function allows you to iterate over the content of a directory recursively. The function returns a generator object that yields three-item tuples. Each tuple contains the following items:
- The path to the current directory as a string
- The names of all the immediate subdirectories as a list of strings
- The names of all the files in the current directory as a list of strings
Now say that you want to iterate over your home directory and list only the files. You can do something like this:
This code will issue a long output depending on the current content of your home directory. Note that you need to provide a string with the path to your user folder for the example to work. The _ placeholder variable will hold the unwanted data.
In contrast, the filenames variable will hold the list of files in the current directory, which is the data that you need. The code will print the list of filenames. Go ahead and give it a try!
The assignment operator also comes in handy when you need to provide default argument values in your functions and methods. Default argument values allow you to define functions that take arguments with sensible defaults. These defaults allow you to call the function with specific values or to simply rely on the defaults.
As an example, consider the following function:
This function takes one argument, called name . This argument has a sensible default value that’ll be used when you call the function without arguments. To provide this sensible default value, you use an assignment.
Note: According to PEP 8 , the style guide for Python code, you shouldn’t use spaces around the assignment operator when providing default argument values in function definitions.
Here’s how the function works:
If you don’t provide a name during the call to greet() , then the function uses the default value provided in the definition. If you provide a name, then the function uses it instead of the default one.
Up to this point, you’ve learned a lot about the Python assignment operator and how to use it for writing different types of assignment statements. In the following sections, you’ll dive into a great feature of assignment statements in Python. You’ll learn about augmented assignments .
Augmented Assignment Operators in Python
Python supports what are known as augmented assignments . An augmented assignment combines the assignment operator with another operator to make the statement more concise. Most Python math and bitwise operators have an augmented assignment variation that looks something like this:
Note that $ isn’t a valid Python operator. In this example, it’s a placeholder for a generic operator. This statement works as follows:
- Evaluate expression to produce a value.
- Run the operation defined by the operator that prefixes the = sign, using the previous value of variable and the return value of expression as operands.
- Assign the resulting value back to variable .
In practice, an augmented assignment like the above is equivalent to the following statement:
As you can conclude, augmented assignments are syntactic sugar . They provide a shorthand notation for a specific and popular kind of assignment.
For example, say that you need to define a counter variable to count some stuff in your code. You can use the += operator to increment counter by 1 using the following code:
In this example, the += operator, known as augmented addition , adds 1 to the previous value in counter each time you run the statement counter += 1 .
It’s important to note that unlike regular assignments, augmented assignments don’t create new variables. They only allow you to update existing variables. If you use an augmented assignment with an undefined variable, then you get a NameError :
Python evaluates the right side of the statement before assigning the resulting value back to the target variable. In this specific example, when Python tries to compute x + 1 , it finds that x isn’t defined.
Great! You now know that an augmented assignment consists of combining the assignment operator with another operator, like a math or bitwise operator. To continue this discussion, you’ll learn which math operators have an augmented variation in Python.
An equation like x = x + b doesn’t make sense in math. But in programming, a statement like x = x + b is perfectly valid and can be extremely useful. It adds b to x and reassigns the result back to x .
As you already learned, Python provides an operator to shorten x = x + b . Yes, the += operator allows you to write x += b instead. Python also offers augmented assignment operators for most math operators. Here’s a summary:
Operator | Description | Example | Equivalent |
---|---|---|---|
Adds the right operand to the left operand and stores the result in the left operand | |||
Subtracts the right operand from the left operand and stores the result in the left operand | |||
Multiplies the right operand with the left operand and stores the result in the left operand | |||
Divides the left operand by the right operand and stores the result in the left operand | |||
Performs of the left operand by the right operand and stores the result in the left operand | |||
Finds the remainder of dividing the left operand by the right operand and stores the result in the left operand | |||
Raises the left operand to the power of the right operand and stores the result in the left operand |
The Example column provides generic examples of how to use the operators in actual code. Note that x must be previously defined for the operators to work correctly. On the other hand, y can be either a concrete value or an expression that returns a value.
Note: The matrix multiplication operator ( @ ) doesn’t support augmented assignments yet.
Consider the following example of matrix multiplication using NumPy arrays:
Note that the exception traceback indicates that the operation isn’t supported yet.
To illustrate how augmented assignment operators work, say that you need to create a function that takes an iterable of numeric values and returns their sum. You can write this function like in the code below:
In this function, you first initialize total to 0 . In each iteration, the loop adds a new number to total using the augmented addition operator ( += ). When the loop terminates, total holds the sum of all the input numbers. Variables like total are known as accumulators . The += operator is typically used to update accumulators.
Note: Computing the sum of a series of numeric values is a common operation in programming. Python provides the built-in sum() function for this specific computation.
Another interesting example of using an augmented assignment is when you need to implement a countdown while loop to reverse an iterable. In this case, you can use the -= operator:
In this example, custom_reversed() is a generator function because it uses yield . Calling the function creates an iterator that yields items from the input iterable in reverse order. To decrement the control variable, index , you use an augmented subtraction statement that subtracts 1 from the variable in every iteration.
Note: Similar to summing the values in an iterable, reversing an iterable is also a common requirement. Python provides the built-in reversed() function for this specific computation, so you don’t have to implement your own. The above example only intends to show the -= operator in action.
Finally, counters are a special type of accumulators that allow you to count objects. Here’s an example of a letter counter:
To create this counter, you use a Python dictionary. The keys store the letters. The values store the counts. Again, to increment the counter, you use an augmented addition.
Counters are so common in programming that Python provides a tool specially designed to facilitate the task of counting. Check out Python’s Counter: The Pythonic Way to Count Objects for a complete guide on how to use this tool.
The += and *= augmented assignment operators also work with sequences , such as lists, tuples, and strings. The += operator performs augmented concatenations , while the *= operator performs augmented repetition .
These operators behave differently with mutable and immutable data types:
Operator | Description | Example |
---|---|---|
Runs an augmented concatenation operation on the target sequence. Mutable sequences are updated in place. If the sequence is immutable, then a new sequence is created and assigned back to the target name. | ||
Adds to itself times. Mutable sequences are updated in place. If the sequence is immutable, then a new sequence is created and assigned back to the target name. |
Note that the augmented concatenation operator operates on two sequences, while the augmented repetition operator works on a sequence and an integer number.
Consider the following examples and pay attention to the result of calling the id() function:
Mutable sequences like lists support the += augmented assignment operator through the .__iadd__() method, which performs an in-place addition. This method mutates the underlying list, appending new values to its end.
Note: If the left operand is mutable, then x += y may not be completely equivalent to x = x + y . For example, if you do list_1 = list_1 + list_2 instead of list_1 += list_2 above, then you’ll create a new list instead of mutating the existing one. This may be important if other variables refer to the same list.
Immutable sequences, such as tuples and strings, don’t provide an .__iadd__() method. Therefore, augmented concatenations fall back to the .__add__() method, which doesn’t modify the sequence in place but returns a new sequence.
There’s another difference between mutable and immutable sequences when you use them in an augmented concatenation. Consider the following examples:
With mutable sequences, the data to be concatenated can come as a list, tuple, string, or any other iterable. In contrast, with immutable sequences, the data can only come as objects of the same type. You can concatenate tuples to tuples and strings to strings, for example.
Again, the augmented repetition operator works with a sequence on the left side of the operator and an integer on the right side. This integer value represents the number of repetitions to get in the resulting sequence:
When the *= operator operates on a mutable sequence, it falls back to the .__imul__() method, which performs the operation in place, modifying the underlying sequence. In contrast, if *= operates on an immutable sequence, then .__mul__() is called, returning a new sequence of the same type.
Note: Values of n less than 0 are treated as 0 , which returns an empty sequence of the same data type as the target sequence on the left side of the *= operand.
Note that a_list[0] is a_list[3] returns True . This is because the *= operator doesn’t make a copy of the repeated data. It only reflects the data. This behavior can be a source of issues when you use the operator with mutable values.
For example, say that you want to create a list of lists to represent a matrix, and you need to initialize the list with n empty lists, like in the following code:
In this example, you use the *= operator to populate matrix with three empty lists. Now check out what happens when you try to populate the first sublist in matrix :
The appended values are reflected in the three sublists. This happens because the *= operator doesn’t make copies of the data that you want to repeat. It only reflects the data. Therefore, every sublist in matrix points to the same object and memory address.
If you ever need to initialize a list with a bunch of empty sublists, then use a list comprehension :
This time, when you populate the first sublist of matrix , your changes aren’t propagated to the other sublists. This is because all the sublists are different objects that live in different memory addresses.
Bitwise operators also have their augmented versions. The logic behind them is similar to that of the math operators. The following table summarizes the augmented bitwise operators that Python provides:
Operator | Operation | Example | Equivalent |
---|---|---|---|
Augmented bitwise AND ( ) | |||
Augmented bitwise OR ( ) | |||
Augmented bitwise XOR ( ) | |||
Augmented bitwise right shift | |||
Augmented bitwise left shift |
The augmented bitwise assignment operators perform the intended operation by taking the current value of the left operand as a starting point for the computation. Consider the following example, which uses the & and &= operators:
Programmers who work with high-level languages like Python rarely use bitwise operations in day-to-day coding. However, these types of operations can be useful in some situations.
For example, say that you’re implementing a Unix-style permission system for your users to access a given resource. In this case, you can use the characters "r" for reading, "w" for writing, and "x" for execution permissions, respectively. However, using bit-based permissions could be more memory efficient:
You can assign permissions to your users with the OR bitwise operator or the augmented OR bitwise operator. Finally, you can use the bitwise AND operator to check if a user has a certain permission, as you did in the final two examples.
You’ve learned a lot about augmented assignment operators and statements in this and the previous sections. These operators apply to math, concatenation, repetition, and bitwise operations. Now you’re ready to look at other assignment variants that you can use in your code or find in other developers’ code.
Other Assignment Variants
So far, you’ve learned that Python’s assignment statements and the assignment operator are present in many different scenarios and use cases. Those use cases include variable creation and initialization, parallel assignments, iterable unpacking, augmented assignments, and more.
In the following sections, you’ll learn about a few variants of assignment statements that can be useful in your future coding. You can also find these assignment variants in other developers’ code. So, you should be aware of them and know how they work in practice.
In short, you’ll learn about:
- Annotated assignment statements with type hints
- Assignment expressions with the walrus operator
- Managed attribute assignments with properties and descriptors
- Implicit assignments in Python
These topics will take you through several interesting and useful examples that showcase the power of Python’s assignment statements.
PEP 526 introduced a dedicated syntax for variable annotation back in Python 3.6 . The syntax consists of the variable name followed by a colon ( : ) and the variable type:
Even though these statements declare three variables with their corresponding data types, the variables aren’t actually created or initialized. So, for example, you can’t use any of these variables in an augmented assignment statement:
If you try to use one of the previously declared variables in an augmented assignment, then you get a NameError because the annotation syntax doesn’t define the variable. To actually define it, you need to use an assignment.
The good news is that you can use the variable annotation syntax in an assignment statement with the = operator:
The first statement in this example is what you can call an annotated assignment statement in Python. You may ask yourself why you should use type annotations in this type of assignment if everybody can see that counter holds an integer number. You’re right. In this example, the variable type is unambiguous.
However, imagine what would happen if you found a variable initialization like the following:
What would be the data type of each user in users ? If the initialization of users is far away from the definition of the User class, then there’s no quick way to answer this question. To clarify this ambiguity, you can provide the appropriate type hint for users :
Now you’re clearly communicating that users will hold a list of User instances. Using type hints in assignment statements that initialize variables to empty collection data types—such as lists, tuples, or dictionaries—allows you to provide more context about how your code works. This practice will make your code more explicit and less error-prone.
Up to this point, you’ve learned that regular assignment statements with the = operator don’t have a return value. They just create or update variables. Therefore, you can’t use a regular assignment to assign a value to a variable within the context of an expression.
Python 3.8 changed this by introducing a new type of assignment statement through PEP 572 . This new statement is known as an assignment expression or named expression .
Note: Expressions are a special type of statement in Python. Their distinguishing characteristic is that expressions always have a return value, which isn’t the case with all types of statements.
Unlike regular assignments, assignment expressions have a return value, which is why they’re called expressions in the first place. This return value is automatically assigned to a variable. To write an assignment expression, you must use the walrus operator ( := ), which was named for its resemblance to the eyes and tusks of a walrus lying on its side.
The general syntax of an assignment statement is as follows:
This expression looks like a regular assignment. However, instead of using the assignment operator ( = ), it uses the walrus operator ( := ). For the expression to work correctly, the enclosing parentheses are required in most use cases. However, there are certain situations in which these parentheses are superfluous. Either way, they won’t hurt you.
Assignment expressions come in handy when you want to reuse the result of an expression or part of an expression without using a dedicated assignment to grab this value beforehand.
Note: Assignment expressions with the walrus operator have several practical use cases. They also have a few restrictions. For example, they’re illegal in certain contexts, such as lambda functions, parallel assignments, and augmented assignments.
For a deep dive into this special type of assignment, check out The Walrus Operator: Python’s Assignment Expressions .
A particularly handy use case for assignment expressions is when you need to grab the result of an expression used in the context of a conditional statement. For example, say that you need to write a function to compute the mean of a sample of numeric values. Without the walrus operator, you could do something like this:
In this example, the sample size ( n ) is a value that you need to reuse in two different computations. First, you need to check whether the sample has data points or not. Then you need to use the sample size to compute the mean. To be able to reuse n , you wrote a dedicated assignment statement at the beginning of your function to grab the sample size.
You can avoid this extra step by combining it with the first use of the target value, len(sample) , using an assignment expression like the following:
The assignment expression introduced in the conditional computes the sample size and assigns it to n . This way, you guarantee that you have a reference to the sample size to use in further computations.
Because the assignment expression returns the sample size anyway, the conditional can check whether that size equals 0 or not and then take a certain course of action depending on the result of this check. The return statement computes the sample’s mean and sends the result back to the function caller.
Python provides a few tools that allow you to fine-tune the operations behind the assignment of attributes. The attributes that run implicit operations on assignments are commonly referred to as managed attributes .
Properties are the most commonly used tool for providing managed attributes in your classes. However, you can also use descriptors and, in some cases, the .__setitem__() special method.
To understand what fine-tuning the operation behind an assignment means, say that you need a Point class that only allows numeric values for its coordinates, x and y . To write this class, you must set up a validation mechanism to reject non-numeric values. You can use properties to attach the validation functionality on top of x and y .
Here’s how you can write your class:
In Point , you use properties for the .x and .y coordinates. Each property has a getter and a setter method . The getter method returns the attribute at hand. The setter method runs the input validation using a try … except block and the built-in float() function. Then the method assigns the result to the actual attribute.
Here’s how your class works in practice:
When you use a property-based attribute as the left operand in an assignment statement, Python automatically calls the property’s setter method, running any computation from it.
Because both .x and .y are properties, the input validation runs whenever you assign a value to either attribute. In the first example, the input values are valid numbers and the validation passes. In the final example, "one" isn’t a valid numeric value, so the validation fails.
If you look at your Point class, you’ll note that it follows a repetitive pattern, with the getter and setter methods looking quite similar. To avoid this repetition, you can use a descriptor instead of a property.
A descriptor is a class that implements the descriptor protocol , which consists of four special methods :
- .__get__() runs when you access the attribute represented by the descriptor.
- .__set__() runs when you use the attribute in an assignment statement.
- .__delete__() runs when you use the attribute in a del statement.
- .__set_name__() sets the attribute’s name, creating a name-aware attribute.
Here’s how your code may look if you use a descriptor to represent the coordinates of your Point class:
You’ve removed repetitive code by defining Coordinate as a descriptor that manages the input validation in a single place. Go ahead and run the following code to try out the new implementation of Point :
Great! The class works as expected. Thanks to the Coordinate descriptor, you now have a more concise and non-repetitive version of your original code.
Another way to fine-tune the operations behind an assignment statement is to provide a custom implementation of .__setitem__() in your class. You’ll use this method in classes representing mutable data collections, such as custom list-like or dictionary-like classes.
As an example, say that you need to create a dictionary-like class that stores its keys in lowercase letters:
In this example, you create a dictionary-like class by subclassing UserDict from collections . Your class implements a .__setitem__() method, which takes key and value as arguments. The method uses str.lower() to convert key into lowercase letters before storing it in the underlying dictionary.
Python implicitly calls .__setitem__() every time you use a key as the left operand in an assignment statement. This behavior allows you to tweak how you process the assignment of keys in your custom dictionary.
Implicit Assignments in Python
Python implicitly runs assignments in many different contexts. In most cases, these implicit assignments are part of the language syntax. In other cases, they support specific behaviors.
Whenever you complete an action in the following list, Python runs an implicit assignment for you:
- Define or call a function
- Define or instantiate a class
- Use the current instance , self
- Import modules and objects
- Use a decorator
- Use the control variable in a for loop or a comprehension
- Use the as qualifier in with statements , imports, and try … except blocks
- Access the _ special variable in an interactive session
Behind the scenes, Python performs an assignment in every one of the above situations. In the following subsections, you’ll take a tour of all these situations.
When you define a function, the def keyword implicitly assigns a function object to your function’s name. Here’s an example:
From this point on, the name greet refers to a function object that lives at a given memory address in your computer. You can call the function using its name and a pair of parentheses with appropriate arguments. This way, you can reuse greet() wherever you need it.
If you call your greet() function with fellow as an argument, then Python implicitly assigns the input argument value to the name parameter on the function’s definition. The parameter will hold a reference to the input arguments.
When you define a class with the class keyword, you’re assigning a specific name to a class object . You can later use this name to create instances of that class. Consider the following example:
In this example, the name User holds a reference to a class object, which was defined in __main__.User . Like with a function, when you call the class’s constructor with the appropriate arguments to create an instance, Python assigns the arguments to the parameters defined in the class initializer .
Another example of implicit assignments is the current instance of a class, which in Python is called self by convention. This name implicitly gets a reference to the current object whenever you instantiate a class. Thanks to this implicit assignment, you can access .name and .job from within the class without getting a NameError in your code.
Import statements are another variant of implicit assignments in Python. Through an import statement, you assign a name to a module object, class, function, or any other imported object. This name is then created in your current namespace so that you can access it later in your code:
In this example, you import the sys module object from the standard library and assign it to the sys name, which is now available in your namespace, as you can conclude from the second call to the built-in dir() function.
You also run an implicit assignment when you use a decorator in your code. The decorator syntax is just a shortcut for a formal assignment like the following:
Here, you call decorator() with a function object as an argument. This call will typically add functionality on top of the existing function, func() , and return a function object, which is then reassigned to the func name.
The decorator syntax is syntactic sugar for replacing the previous assignment, which you can now write as follows:
Even though this new code looks pretty different from the above assignment, the code implicitly runs the same steps.
Another situation in which Python automatically runs an implicit assignment is when you use a for loop or a comprehension. In both cases, you can have one or more control variables that you then use in the loop or comprehension body:
The memory address of control_variable changes on each iteration of the loop. This is because Python internally reassigns a new value from the loop iterable to the loop control variable on each cycle.
The same behavior appears in comprehensions:
In the end, comprehensions work like for loops but use a more concise syntax. This comprehension creates a new list of strings that mimic the output from the previous example.
The as keyword in with statements, except clauses, and import statements is another example of an implicit assignment in Python. This time, the assignment isn’t completely implicit because the as keyword provides an explicit way to define the target variable.
In a with statement, the target variable that follows the as keyword will hold a reference to the context manager that you’re working with. As an example, say that you have a hello.txt file with the following content:
You want to open this file and print each of its lines on your screen. In this case, you can use the with statement to open the file using the built-in open() function.
In the example below, you accomplish this. You also add some calls to print() that display information about the target variable defined by the as keyword:
This with statement uses the open() function to open hello.txt . The open() function is a context manager that returns a text file object represented by an io.TextIOWrapper instance.
Since you’ve defined a hello target variable with the as keyword, now that variable holds a reference to the file object itself. You confirm this by printing the object and its memory address. Finally, the for loop iterates over the lines and prints this content to the screen.
When it comes to using the as keyword in the context of an except clause, the target variable will contain an exception object if any exception occurs:
In this example, you run a division that raises a ZeroDivisionError . The as keyword assigns the raised exception to error . Note that when you print the exception object, you get only the message because exceptions have a custom .__str__() method that supports this behavior.
There’s a final detail to remember when using the as specifier in a try … except block like the one in the above example. Once you leave the except block, the target variable goes out of scope , and you can’t use it anymore.
Finally, Python’s import statements also support the as keyword. In this context, you can use as to import objects with a different name:
In these examples, you use the as keyword to import the numpy package with the np name and pandas with the name pd . If you call dir() , then you’ll realize that np and pd are now in your namespace. However, the numpy and pandas names are not.
Using the as keyword in your imports comes in handy when you want to use shorter names for your objects or when you need to use different objects that originally had the same name in your code. It’s also useful when you want to make your imported names non-public using a leading underscore, like in import sys as _sys .
The final implicit assignment that you’ll learn about in this tutorial only occurs when you’re using Python in an interactive session. Every time you run a statement that returns a value, the interpreter stores the result in a special variable denoted by a single underscore character ( _ ).
You can access this special variable as you’d access any other variable:
These examples cover several situations in which Python internally uses the _ variable. The first two examples evaluate expressions. Expressions always have a return value, which is automatically assigned to the _ variable every time.
When it comes to function calls, note that if your function returns a fruitful value, then _ will hold it. In contrast, if your function returns None , then the _ variable will remain untouched.
The next example consists of a regular assignment statement. As you already know, regular assignments don’t return any value, so the _ variable isn’t updated after these statements run. Finally, note that accessing a variable in an interactive session returns the value stored in the target variable. This value is then assigned to the _ variable.
Note that since _ is a regular variable, you can use it in other expressions:
In this example, you first create a list of values. Then you call len() to get the number of values in the list. Python automatically stores this value in the _ variable. Finally, you use _ to compute the mean of your list of values.
Now that you’ve learned about some of the implicit assignments that Python runs under the hood, it’s time to dig into a final assignment-related topic. In the following few sections, you’ll learn about some illegal and dangerous assignments that you should be aware of and avoid in your code.
Illegal and Dangerous Assignments in Python
In Python, you’ll find a few situations in which using assignments is either forbidden or dangerous. You must be aware of these special situations and try to avoid them in your code.
In the following sections, you’ll learn when using assignment statements isn’t allowed in Python. You’ll also learn about some situations in which using assignments should be avoided if you want to keep your code consistent and robust.
You can’t use Python keywords as variable names in assignment statements. This kind of assignment is explicitly forbidden. If you try to use a keyword as a variable name in an assignment, then you get a SyntaxError :
Whenever you try to use a keyword as the left operand in an assignment statement, you get a SyntaxError . Keywords are an intrinsic part of the language and can’t be overridden.
If you ever feel the need to name one of your variables using a Python keyword, then you can append an underscore to the name of your variable:
In this example, you’re using the desired name for your variables. Because you added a final underscore to the names, Python doesn’t recognize them as keywords, so it doesn’t raise an error.
Note: Even though adding an underscore at the end of a name is an officially recommended practice , it can be confusing sometimes. Therefore, try to find an alternative name or use a synonym whenever you find yourself using this convention.
For example, you can write something like this:
In this example, using the name booking_class for your variable is way clearer and more descriptive than using class_ .
You’ll also find that you can use only a few keywords as part of the right operand in an assignment statement. Those keywords will generally define simple statements that return a value or object. These include lambda , and , or , not , True , False , None , in , and is . You can also use the for keyword when it’s part of a comprehension and the if keyword when it’s used as part of a ternary operator .
In an assignment, you can never use a compound statement as the right operand. Compound statements are those that require an indented block, such as for and while loops, conditionals, with statements, try … except blocks, and class or function definitions.
Sometimes, you need to name variables, but the desired or ideal name is already taken and used as a built-in name. If this is your case, think harder and find another name. Don’t shadow the built-in.
Shadowing built-in names can cause hard-to-identify problems in your code. A common example of this issue is using list or dict to name user-defined variables. In this case, you override the corresponding built-in names, which won’t work as expected if you use them later in your code.
Consider the following example:
The exception in this example may sound surprising. How come you can’t use list() to build a list from a call to map() that returns a generator of square numbers?
By using the name list to identify your list of numbers, you shadowed the built-in list name. Now that name points to a list object rather than the built-in class. List objects aren’t callable, so your code no longer works.
In Python, you’ll have nothing that warns against using built-in, standard-library, or even relevant third-party names to identify your own variables. Therefore, you should keep an eye out for this practice. It can be a source of hard-to-debug errors.
In programming, a constant refers to a name associated with a value that never changes during a program’s execution. Unlike other programming languages, Python doesn’t have a dedicated syntax for defining constants. This fact implies that Python doesn’t have constants in the strict sense of the word.
Python only has variables. If you need a constant in Python, then you’ll have to define a variable and guarantee that it won’t change during your code’s execution. To do that, you must avoid using that variable as the left operand in an assignment statement.
To tell other Python programmers that a given variable should be treated as a constant, you must write your variable’s name in capital letters with underscores separating the words. This naming convention has been adopted by the Python community and is a recommendation that you’ll find in the Constants section of PEP 8 .
In the following examples, you define some constants in Python:
The problem with these constants is that they’re actually variables. Nothing prevents you from changing their value during your code’s execution. So, at any time, you can do something like the following:
These assignments modify the value of two of your original constants. Python doesn’t complain about these changes, which can cause issues later in your code. As a Python developer, you must guarantee that named constants in your code remain constant.
The only way to do that is never to use named constants in an assignment statement other than the constant definition.
You’ve learned a lot about Python’s assignment operators and how to use them for writing assignment statements . With this type of statement, you can create, initialize, and update variables according to your needs. Now you have the required skills to fully manage the creation and mutation of variables in your Python code.
In this tutorial, you’ve learned how to:
- Write assignment statements using Python’s assignment operators
- Work with augmented assignments in Python
- Explore assignment variants, like assignment expression and managed attributes
- Identify illegal and dangerous assignments in Python
Learning about the Python assignment operator and how to use it in assignment statements is a fundamental skill in Python. It empowers you to write reliable and effective Python code.
š Python Tricks š
Get a short & sweet Python Trick delivered to your inbox every couple of days. No spam ever. Unsubscribe any time. Curated by the Real Python team.
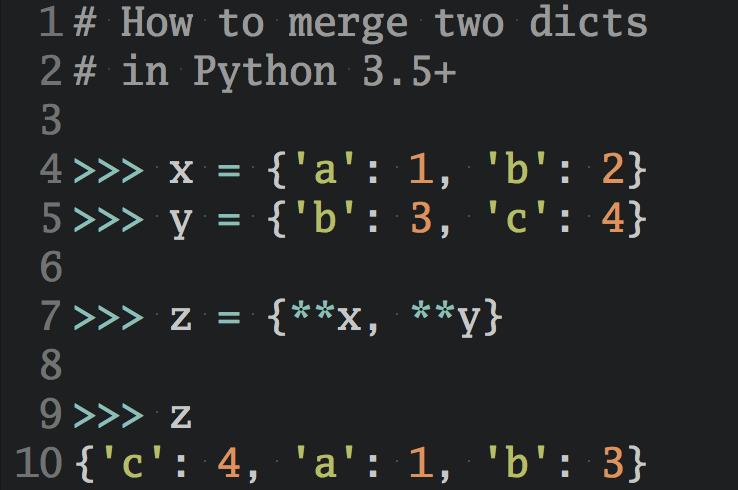
About Leodanis Pozo Ramos
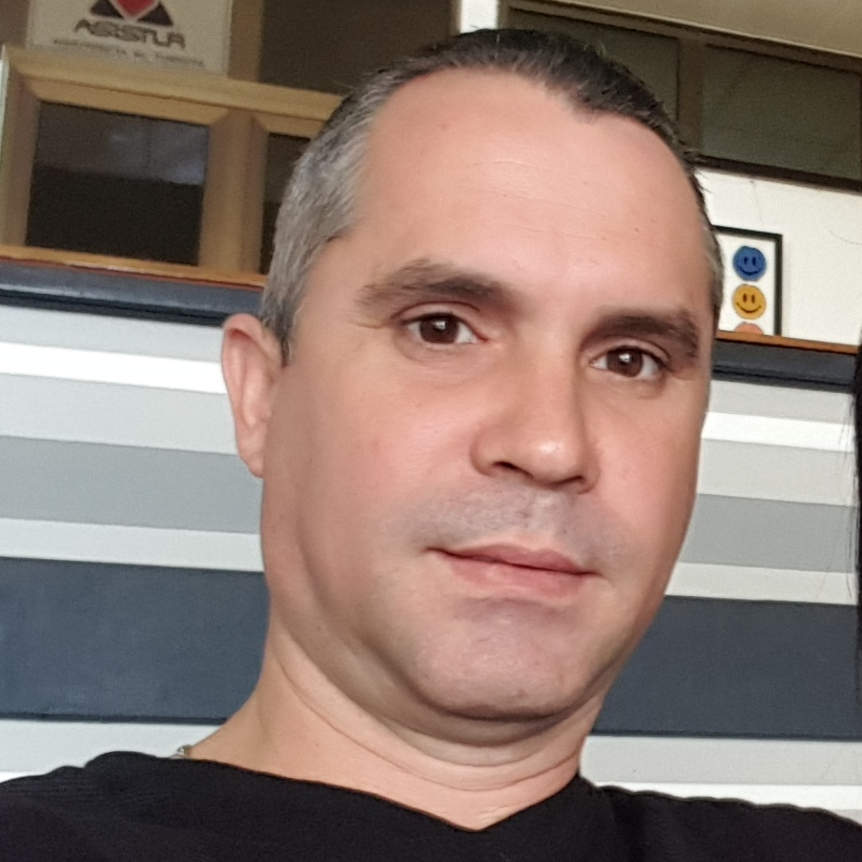
Leodanis is an industrial engineer who loves Python and software development. He's a self-taught Python developer with 6+ years of experience. He's an avid technical writer with a growing number of articles published on Real Python and other sites.
Each tutorial at Real Python is created by a team of developers so that it meets our high quality standards. The team members who worked on this tutorial are:
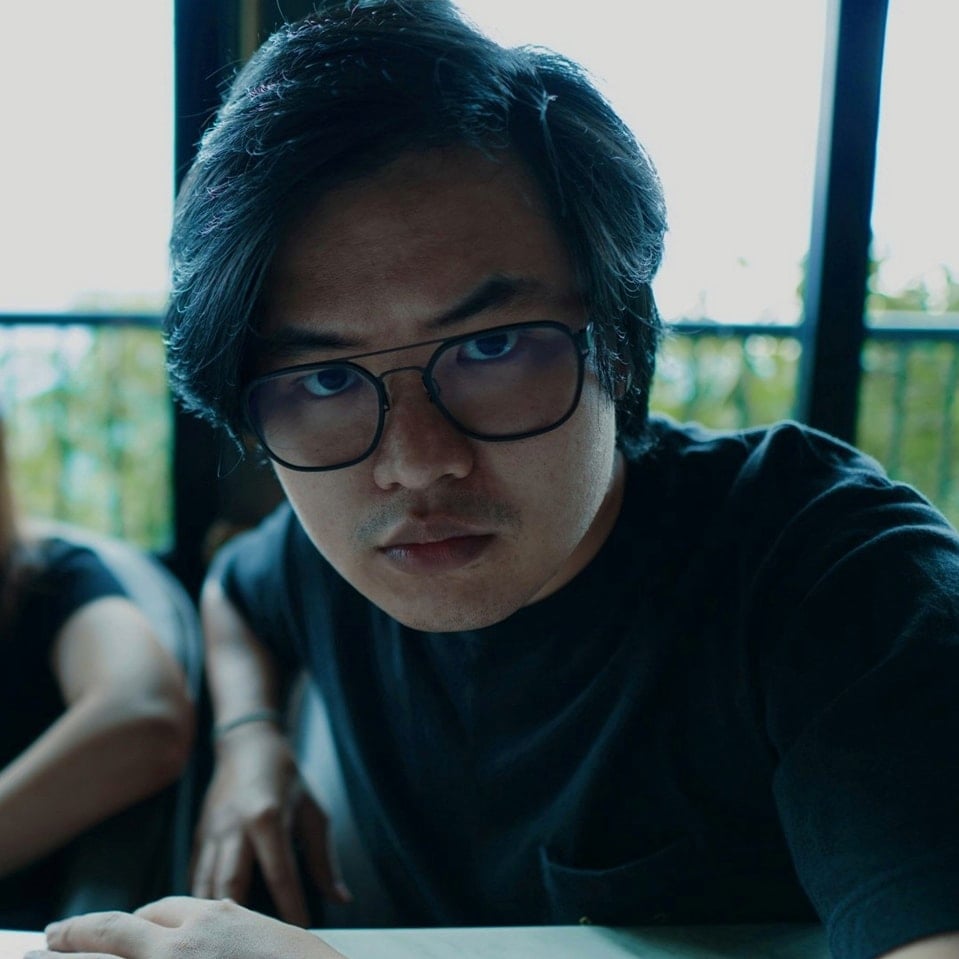
Master Real-World Python Skills With Unlimited Access to Real Python
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
What Do You Think?
Whatās your #1 takeaway or favorite thing you learned? How are you going to put your newfound skills to use? Leave a comment below and let us know.
Commenting Tips: The most useful comments are those written with the goal of learning from or helping out other students. Get tips for asking good questions and get answers to common questions in our support portal . Looking for a real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session . Happy Pythoning!
Keep Learning
Related Topics: intermediate best-practices python
Keep reading Real Python by creating a free account or signing in:
Already have an account? Sign-In
Almost there! Complete this form and click the button below to gain instant access:
Python's Assignment Operator: Write Robust Assignments (Source Code)
š No spam. We take your privacy seriously.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
?: operator - the ternary conditional operator
- 11 contributors
The conditional operator ?: , also known as the ternary conditional operator, evaluates a Boolean expression and returns the result of one of the two expressions, depending on whether the Boolean expression evaluates to true or false , as the following example shows:
As the preceding example shows, the syntax for the conditional operator is as follows:
The condition expression must evaluate to true or false . If condition evaluates to true , the consequent expression is evaluated, and its result becomes the result of the operation. If condition evaluates to false , the alternative expression is evaluated, and its result becomes the result of the operation. Only consequent or alternative is evaluated. Conditional expressions are target-typed. That is, if a target type of a conditional expression is known, the types of consequent and alternative must be implicitly convertible to the target type, as the following example shows:
If a target type of a conditional expression is unknown (for example, when you use the var keyword) or the type of consequent and alternative must be the same or there must be an implicit conversion from one type to the other:
The conditional operator is right-associative, that is, an expression of the form
is evaluated as
You can use the following mnemonic device to remember how the conditional operator is evaluated:
Conditional ref expression
A conditional ref expression conditionally returns a variable reference, as the following example shows:
You can ref assign the result of a conditional ref expression, use it as a reference return or pass it as a ref , out , in , or ref readonly method parameter . You can also assign to the result of a conditional ref expression, as the preceding example shows.
The syntax for a conditional ref expression is as follows:
Like the conditional operator, a conditional ref expression evaluates only one of the two expressions: either consequent or alternative .
In a conditional ref expression, the type of consequent and alternative must be the same. Conditional ref expressions aren't target-typed.
Conditional operator and an if statement
Use of the conditional operator instead of an if statement might result in more concise code in cases when you need conditionally to compute a value. The following example demonstrates two ways to classify an integer as negative or nonnegative:
Operator overloadability
A user-defined type can't overload the conditional operator.
C# language specification
For more information, see the Conditional operator section of the C# language specification .
Specifications for newer features are:
- Target-typed conditional expression
- Simplify conditional expression (style rule IDE0075)
- C# operators and expressions
- if statement
- ?. and ?[] operators
- ?? and ??= operators
- ref keyword
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Conditional branching: if, '?'
Sometimes, we need to perform different actions based on different conditions.
To do that, we can use the if statement and the conditional operator ? , thatās also called a āquestion markā operator.
The āifā statement
The if(...) statement evaluates a condition in parentheses and, if the result is true , executes a block of code.
For example:
In the example above, the condition is a simple equality check ( year == 2015 ), but it can be much more complex.
If we want to execute more than one statement, we have to wrap our code block inside curly braces:
We recommend wrapping your code block with curly braces {} every time you use an if statement, even if there is only one statement to execute. Doing so improves readability.
Boolean conversion
The if (ā¦) statement evaluates the expression in its parentheses and converts the result to a boolean.
Letās recall the conversion rules from the chapter Type Conversions :
- A number 0 , an empty string "" , null , undefined , and NaN all become false . Because of that they are called āfalsyā values.
- Other values become true , so they are called ātruthyā.
So, the code under this condition would never execute:
ā¦and inside this condition ā it always will:
We can also pass a pre-evaluated boolean value to if , like this:
The āelseā clause
The if statement may contain an optional else block. It executes when the condition is falsy.
Several conditions: āelse ifā
Sometimes, weād like to test several variants of a condition. The else if clause lets us do that.
In the code above, JavaScript first checks year < 2015 . If that is falsy, it goes to the next condition year > 2015 . If that is also falsy, it shows the last alert .
There can be more else if blocks. The final else is optional.
Conditional operator ā?ā
Sometimes, we need to assign a variable depending on a condition.
For instance:
The so-called āconditionalā or āquestion markā operator lets us do that in a shorter and simpler way.
The operator is represented by a question mark ? . Sometimes itās called āternaryā, because the operator has three operands. It is actually the one and only operator in JavaScript which has that many.
The syntax is:
The condition is evaluated: if itās truthy then value1 is returned, otherwise ā value2 .
Technically, we can omit the parentheses around age > 18 . The question mark operator has a low precedence, so it executes after the comparison > .
This example will do the same thing as the previous one:
But parentheses make the code more readable, so we recommend using them.
In the example above, you can avoid using the question mark operator because the comparison itself returns true/false :
Multiple ā?ā
A sequence of question mark operators ? can return a value that depends on more than one condition.
It may be difficult at first to grasp whatās going on. But after a closer look, we can see that itās just an ordinary sequence of tests:
- The first question mark checks whether age < 3 .
- If true ā it returns 'Hi, baby!' . Otherwise, it continues to the expression after the colon ā:ā, checking age < 18 .
- If thatās true ā it returns 'Hello!' . Otherwise, it continues to the expression after the next colon ā:ā, checking age < 100 .
- If thatās true ā it returns 'Greetings!' . Otherwise, it continues to the expression after the last colon ā:ā, returning 'What an unusual age!' .
Hereās how this looks using if..else :
Non-traditional use of ā?ā
Sometimes the question mark ? is used as a replacement for if :
Depending on the condition company == 'Netscape' , either the first or the second expression after the ? gets executed and shows an alert.
We donāt assign a result to a variable here. Instead, we execute different code depending on the condition.
Itās not recommended to use the question mark operator in this way.
The notation is shorter than the equivalent if statement, which appeals to some programmers. But it is less readable.
Here is the same code using if for comparison:
Our eyes scan the code vertically. Code blocks which span several lines are easier to understand than a long, horizontal instruction set.
The purpose of the question mark operator ? is to return one value or another depending on its condition. Please use it for exactly that. Use if when you need to execute different branches of code.
if (a string with zero)
Will alert be shown?
Yes, it will.
Any string except an empty one (and "0" is not empty) becomes true in the logical context.
We can run and check:
The name of JavaScript
Using the if..else construct, write the code which asks: āWhat is the āofficialā name of JavaScript?ā
If the visitor enters āECMAScriptā, then output āRight!ā, otherwise ā output: āYou donāt know? ECMAScript!ā
Demo in new window
Show the sign
Using if..else , write the code which gets a number via prompt and then shows in alert :
- 1 , if the value is greater than zero,
- -1 , if less than zero,
- 0 , if equals zero.
In this task we assume that the input is always a number.
Rewrite 'if' into '?'
Rewrite this if using the conditional operator '?' :
Rewrite 'if..else' into '?'
Rewrite if..else using multiple ternary operators '?' .
For readability, itās recommended to split the code into multiple lines.
Lesson navigation
- Ā© 2007ā2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Verilog conditional assignments without using procedural blocks like VHDL with/select
I am trying to find a way to conditionally assign values to a signal in verilog similar to the with/select in VHDL. So far I found two ways https://www.chipverify.com/verilog/verilog-4to1-mux .
This is the first one:
Second one:
Both examples were taken from the link I shared before, my QUESTION IS if there is something in Verilog, similar to VHDL using the with/select, that is easy to understand without using procedural blocks?.
The first verilog example does the job but it takes a bit to understand the logic. Please keep in mind I am not complaining about or criticizing any language, I just want to know how to improve code readability for this particular case.
- \$\begingroup\$ What do you mean by "better way"? Better readability? Optimized for speed/power/area? \$\endgroup\$ – Mitu Raj Commented Apr 9, 2021 at 17:27
- 1 \$\begingroup\$ @MituRaj, you got it, I meant better readability, I will try to edit my post. Thanks \$\endgroup\$ – JC_Onp Commented Apr 12, 2021 at 13:44

2 Answers 2
If you really want something based on an assign statement, your version can be improved:
It's much more readable your version. If you're not used to the ternary conditional operator, well you just have to get used to it. It's part of C and many C-like languages (including Perl) but it's very widely used in Verilog since it's a natural multiplex operator, and it can be used in the middle of complex expressions. Very helpful, and something I miss a lot now that I'm at a VHDL company.
You can simplify the procedural block by using an implicit sensitivity list, always @* in Verilog and preferably always_comb in SystemVerilog. And you should not be using non-blocking assignments in combinational logic.
Almost the same amount of typing as the VHDL with/select.
Your Answer
Sign up or log in, post as a guest.
Required, but never shown
By clicking āPost Your Answerā, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged fpga vhdl verilog hdl or ask your own question .
- The Overflow Blog
- Scaling systems to manage all the metadata ABOUT the data
- Navigating cities of code with Norris Numbers
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
Hot Network Questions
- Does Gimp do batch processing?
- What is the meaning of "Exit, pursued by a bear"?
- What is a word/phrase that best describes a "blatant disregard or neglect" for something, but with the connotation of that they should have known?
- A study on the speed of gravity
- How to Vertically Join Images?
- How would a culture living in an extremely vertical environment deal with dead bodies?
- Can a Statute of Limitations claim be rejected by the court?
- Power line crossing data lines via the ground plane
- Do "Whenever X becomes the target of a spell" abilities get triggered by counterspell?
- Why would Space Colonies even want to secede?
- Making wobbly 6x4’ table stable
- Is there any point "clean-installing" on a brand-new MacBook?
- What is the airspeed record for a helicopter flying backwards?
- Questions about best way to raise the handlebar on my bike
- Base change in Chriss-Ginzburg
- How do you "stealth" a relativistic superweapon?
- What was the reason for not personifying God's spirit in NABRE's translation of John 14:17?
- DIN Rail Logic Gate
- How is 'or' used in the sentence 'alerting no one or killing no one but key targets'?
- Three 7-letter words, 21 unique letters, excludes either HM or QS
- On Schengen Visa - Venice to Zagreb - by bus
- How to cite a book if only its chapters have DOIs?
- Will the US Customs be suspicious of my luggage if i bought a lot of the same item?
- Repeats: Simpler at the cost of more redundant?

Verilog Conditional Operator
Just what the heck is that question mark doing.
Have you ever come across a strange looking piece of Verilog code that has a question mark in the middle of it? A question mark in the middle of a line of code looks so bizarre; they’re supposed to go at the end of sentences! However in Verilog the ? operator is a very useful one, but it does take a bit of getting used to.
The question mark is known in Verilog as a conditional operator though in other programming languages it also is referred to as a ternary operator , an inline if , or a ternary if . It is used as a short-hand way to write a conditional expression in Verilog (rather than using if/else statements). Let’s look at how it is used:
Here, condition is the check that the code is performing. This condition might be things like, “Is the value in A greater than the value in B?” or “Is A=1?”. Depending on if this condition evaluates to true, the first expression is chosen. If the condition evaluates to false, the part after the colon is chosen. I wrote an example of this. The code below is really elegant stuff. The way I look at the question mark operator is I say to myself, “Tell me about the value in r_Check. If it’s true, then return “HI THERE” if it’s false, then return “POTATO”. You can also use the conditional operator to assign signals , as shown with the signal w_Test1 in the example below. Assigning signals with the conditional operator is useful!
Nested Conditional Operators
There are examples in which it might be useful to combine two or more conditional operators in a single assignment. Consider the truth table below. The truth table shows a 2-input truth table. You need to know the value of both r_Sel[1] and r_Sel[0] to determine the value of the output w_Out. This could be achieved with a bunch of if-else if-else if combinations, or a case statement, but it’s much cleaner and simpler to use the conditional operator to achieve the same goal.
r_Sel[1] | r_Sel[0] | Output w_Out |
0 | 0 | 1 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
Learn Verilog
One Comment
The input “r_Sel” must be reg type? Could it be wire type?
Leave A Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.
Verilog Conditional Statements
In Verilog, conditional statements are used to control the flow of execution based on certain conditions. There are several types of conditional statements in Verilog listed below.
Conditional Operator
The conditional operator allows you to assign a value to a variable based on a condition. If the condition is true, expression_1 is assigned to the variable. Otherwise, expression_2 is assigned.
Nested conditional operators
Conditional operators can be nested to any level but it can affect readability of code.
Here are some of the advantages of using conditional operators:
- Concise syntax : The conditional operator allows for a compact and concise representation of conditional assignments. It reduces the amount of code needed compared to using if-else statements or case statements.
- Readability : The conditional operator can enhance code readability, especially for simple conditional assignments. It clearly expresses the intent of assigning different values based on a condition in a single line.
And some disadvantages:
- Limited functionality : The conditional operator is primarily used for simple conditional assignments. It may not be suitable for complex conditions or multiple actions, as it can quickly become unreadable and difficult to maintain.
- Lack of flexibility : The conditional operator only allows for a binary choice based on the condition. It cannot handle multiple cases or multiple actions within a single line of code.
- Potential for reduced readability : While the conditional operator can enhance code readability for simple assignments, it can also make the code more difficult to understand if the condition and assigned values become complex.
if-else statement
The if-else statement allows you to perform different actions based on a condition.
If the condition evaluates to true, statement 1 is executed. Otherwise, statement 2 is executed.
Read more on Verilog if-else-if statements
case statement
The case statement is used when you have multiple conditions and want to perform different actions based on the value of a variable.
The expression is evaluated, and based on its value, the corresponding statement is executed. If none of the values match the expression, the statement under default is executed.
Read more on Verilog case statement
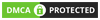

Data to Fish
5 ways to apply an IF condition in Pandas DataFrame
In this guide, you’ll see 5 different ways to apply an IF condition in Pandas DataFrame.
Specifically, you’ll see how to apply an IF condition for:
- Set of numbers
- Set of numbers and lambda
- Strings and lambda
- OR condition
Applying an IF condition in Pandas DataFrame
Let’s now review the following 5 cases:
(1) IF condition – Set of numbers
Suppose that you created a DataFrame in Python that has 10 numbers (from 1 to 10). You then want to apply the following IF conditions:
- If the number is equal or lower than 4, then assign the value of ‘ Yes ‘
- Otherwise, if the number is greater than 4, then assign the value of ‘ No ‘
This is the general structure that you may use to create the IF condition:
For our example, the Python code would look like this:
Here is the result that you’ll get in Python:
(2) IF condition – set of numbers and lambda
You’ll now see how to get the same results as in case 1 by using lambda, where the conditions are:
Here is the generic structure that you may apply in Python:
For our example:
This is the result that you’ll get, which matches with case 1:
(3) IF condition – strings
Now, let’s create a DataFrame that contains only strings/text with 4 names : Jon, Bill, Maria and Emma.
The conditions are:
- If the name is equal to ‘Bill,’ then assign the value of ‘ Match ‘
- Otherwise, if the name is not ‘Bill,’ then assign the value of ‘ Mismatch ‘
Once you run the above Python code, you’ll see:
(4) IF condition – strings and lambda
You’ll get the same results as in case 3 by using lambda:
And here is the output from Python:
(5) IF condition with OR
Now let’s apply these conditions:
- If the name is ‘Bill’ or ‘Emma,’ then assign the value of ‘ Match ‘
- Otherwise, if the name is neither ‘Bill’ nor ‘Emma,’ then assign the value of ‘ Mismatch ‘
Run the Python code, and you’ll get the following result:
Applying an IF condition under an existing DataFrame column
So far you have seen how to apply an IF condition by creating a new column.
Alternatively, you may store the results under an existing DataFrame column.
For example, let’s say that you created a DataFrame that has 12 numbers, where the last two numbers are zeros :
‘set_of_numbers’: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 0 , 0 ]
You may then apply the following IF conditions, and then store the results under the existing ‘ set_of_numbers ‘ column:
- If the number is equal to 0 , then change the value to 999
- If the number is equal to 5 , then change the value to 555
Here are the before and after results, where the ‘5’ became ‘555’ and the 0’s became ‘999’ under the existing ‘set_of_numbers’ column:
On another instance, you may have a DataFrame that contains NaN values . You can then apply an IF condition to replace those values with zeros , as in the example below:
Before you’ll see the NaN values, and after you’ll see the zero values:
You just saw how to apply an IF condition in Pandas DataFrame . There are indeed multiple ways to apply such a condition in Python. You can achieve the same results by using either lambda, or just by sticking with Pandas.
At the end, it boils down to working with the method that is best suited to your needs.
Finally, you may want to check the following external source for additional information about Pandas DataFrame .
Leave a Comment Cancel reply
I agree to comply with the Terms of Service and Privacy Policy when posting a comment.
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
Conditional or Ternary Operator (?:) in C
The conditional operator in C is kind of similar to the if-else statement as it follows the same algorithm as of if-else statement but the conditional operator takes less space and helps to write the if-else statements in the shortest way possible. It is also known as the ternary operator in C as it operates on three operands.
Syntax of Conditional/Ternary Operator in C
The conditional operator can be in the form
Or the syntax can also be in this form
Or syntax can also be in this form
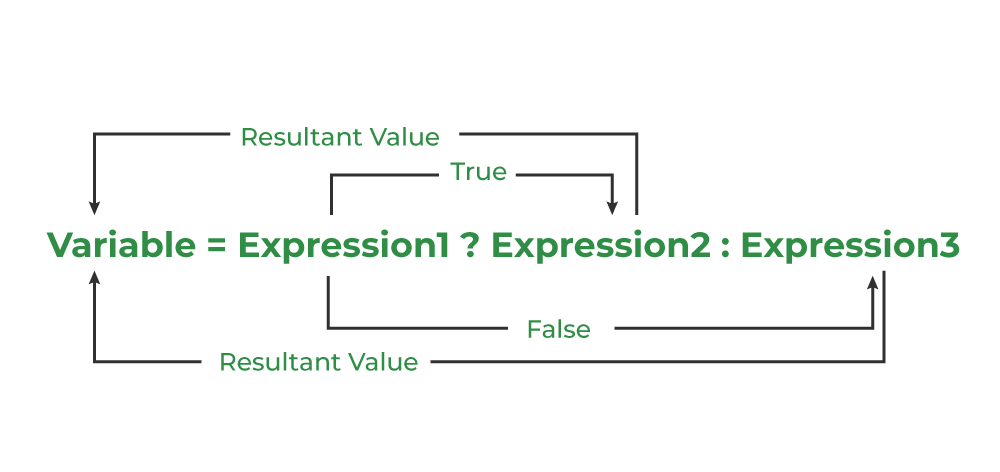
Conditional/Ternary Operator in C
It can be visualized into an if-else statement as:
Since the Conditional Operator ‘?:’ takes three operands to work, hence they are also called ternary operators .
Note: The ternary operator have third most lowest precedence, so we need to use the expressions such that we can avoid errors due to improper operator precedence management.
Working of Conditional/Ternary Operator in C
The working of the conditional operator in C is as follows:
- Step 1: Expression1 is the condition to be evaluated.
- Step 2A: If the condition( Expression1 ) is True then Expression2 will be executed.
- Step 2B : If the condition( Expression1 ) is false then Expression3 will be executed.
- Step 3: Results will be returned.
Flowchart of Conditional/Ternary Operator in C
To understand the working better, we can analyze the flowchart of the conditional operator given below.
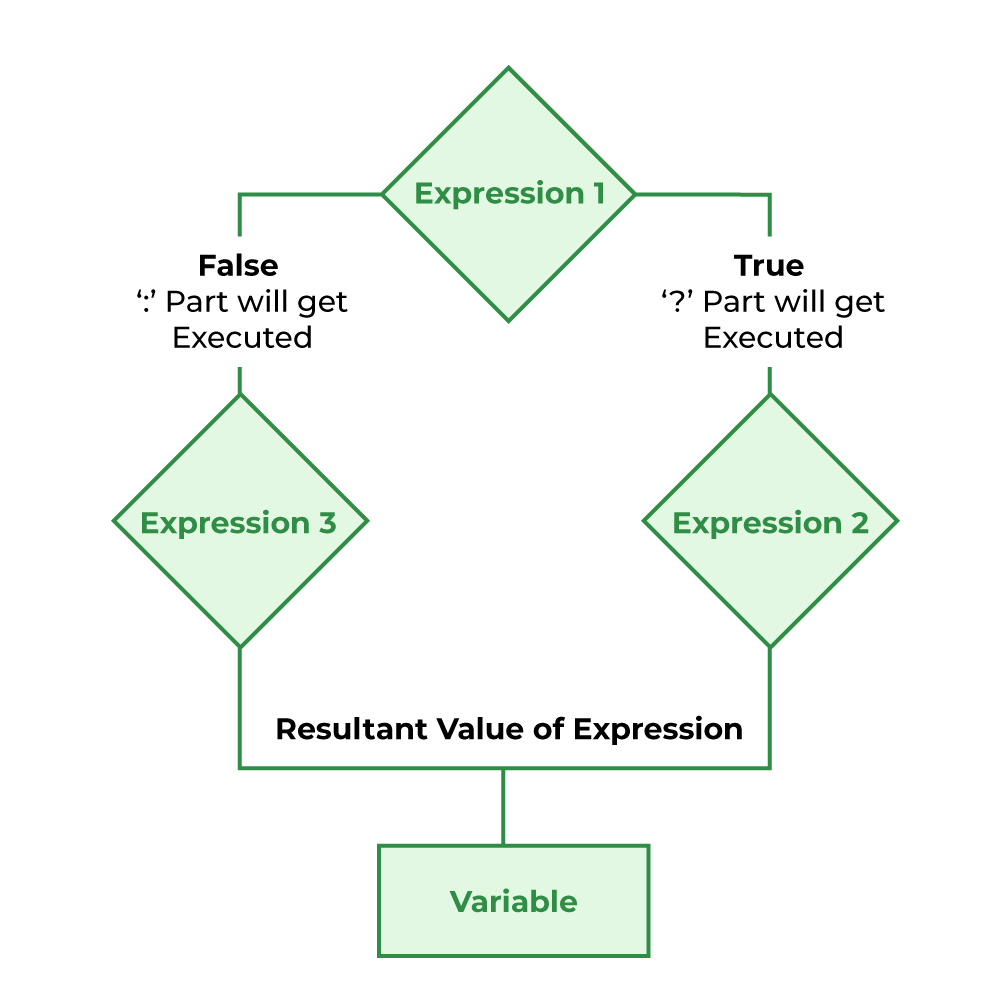
Flowchart of conditional/ternary operator in C
Examples of C Ternary Operator
Example 1: c program to store the greatest of the two numbers using the ternary operator.
Example 2: C Program to check whether a year is a leap year using ternary operator
The conditional operator or ternary operator in C is generally used when we need a short conditional code such as assigning value to a variable based on the condition. It can be used in bigger conditions but it will make the program very complex and unreadable.
FAQs on Conditional/Ternary Operators in C
1. what is the ternary operator in c.
The ternary operator in C is a conditional operator that works on three operands. It works similarly to the if-else statement and executes the code based on the specified condition. It is also called conditional Operator
2. What is the advantage of the conditional operator?
It reduces the line of code when the condition and statements are small.
Please Login to comment...
Similar reads.
- C# Programs
- C/C++ Puzzles
- C-Operators
- cpp-operator
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Ruby Tricks, Idiomatic Ruby, Refactorings and Best Practices
Conditional assignment.
Ruby is heavily influenced by lisp. One piece of obvious inspiration in its design is that conditional operators return values. This makes assignment based on conditions quite clean and simple to read.
There are quite a number of conditional operators you can use. Optimize for readability, maintainability, and concision.
If you're just assigning based on a boolean, the ternary operator works best (this is not specific to ruby/lisp but still good and in the spirit of the following examples!).
If you need to perform more complex logic based on a conditional or allow for > 2 outcomes, if / elsif / else statements work well.
If you're assigning based on the value of a single variable, use case / when A good barometer is to think if you could represent the assignment in a hash. For example, in the following example you could look up the value of opinion in a hash that looks like {"ANGRY" => comfort, "MEH" => ignore ...}
?: conditional operator in Verilog
Compact conditional operators.
Many Verilog designs make use of a compact conditional operator:
A comman example, shown below, is an āenableā mask. Suppose there is some internal signal named a . When enabled by en== 1 , the module assigns q = a , otherwise it assigns q = 0 :
The syntax is also permitted in always blocks:
Assigned Tasks
This assignment uses only a testbench simulation, with no module to implement. Open the file src/testbench.v and examine how it is organized. It uses the conditional operator in an always block to assign q = a^b (XOR) when enabled, else q= 0 .
Run make simulate to test the operation. Verify that the console output is correct. Then modify the testbench to use an assign statement instead of an always block . Change the type of q as appropriate for the assign statement.
Turn in your work using git :
Indicate on Canvas that your assignment is done.
Fact check: Walz retired from Army National Guard after 24 years to run for Congress
Gop vice presidential candidate jd vance claims that the minnesota governor and democratic vice presidential candidate bailed as his unit headed to iraq, but walz retired before his unit was called up..
By Rochelle Olson
Star Tribune
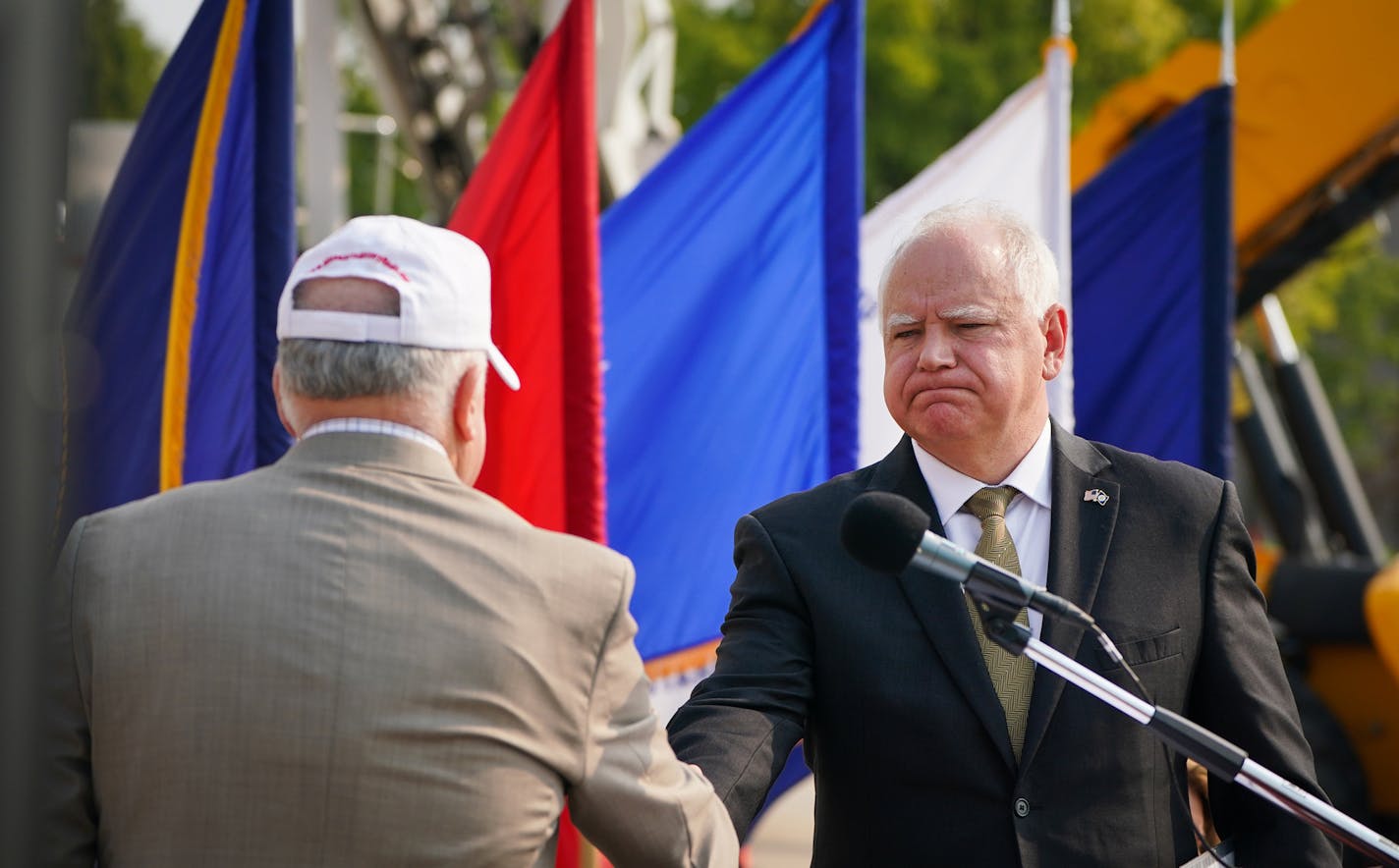
Republican vice presidential nominee JD Vance has renewed an attack on Gov. Tim Walzās military record that his GOP opponent wielded against him during the 2022 gubernatorial campaign, accusing Walz of āstolen valorā for retiring from the military before his regiment deployed to Iraq.
The Ohio senator pitted his service against the governorās on Wednesday in Michigan, saying that he had served honorably in Iraq with the U.S. Marine Corps. Vance, former President Donald Trumpās running mate, was deployed in public affairs as a correspondent. Neither Vance nor Walz saw combat.
āWhen Tim Walz was asked by his country to go to Iraq, you know what he did? He dropped out of the Army and allowed his unit to go without him, a fact that heās been criticized for aggressively by a lot of the people he served. I think itās shameful,ā said Vance , who was on active duty from 2003 to 2007, including deployment to Iraq in 2005 and 2006.
The second-term Minnesota governor and running mate to Vice President Kamala Harris retired from the Army National Guard as a command sergeant major in May 2005 to run for Congress in the First District in southeastern Minnesotan. Walz defeated GOP incumbent Rep. Gil Gutknecht in November 2006.
When Walz retired
Hereās the timeline: Walzās congressional campaign issued a statement in March 2005 saying he still planned to run despite a possible mobilization of Minnesota National Guard soldiers to Iraq. Walz submitted his Guard retirement papers in May 2005. The unitās first call-up notice came in July 2005, and the regiment deployed in March 2006.
Walzās Guard service began when he enlisted in 1981, the day after he turned 17, military records show. The governor recently retold the story of driving with his dad, a Korean War-era veteran, to sign up in his native Nebraska. Like his father, Walz said he expected to go to college on the G.I. Bill and thatās what he eventually did.
Walz re-upped in the Guard multiple times, including signing on for another six-year stint in 2001.
In the years after the Sept. 11, 2001, attack, Walz was a senior enlisted member and a master sergeant, according to military records. He lived in Mankato and served with the southern Minnesota-based First Battalion, 125th Field Artillery. The battalion was deployed to Italy in 2003 to protect against potential threats in Europe while active military forces were deployed to Iraq and Afghanistan, according to Walz.
After the units returned to Minnesota in early 2004, Walz was promoted to command sergeant major.
Walzās critics say the governor inflated his credentials because he retired as a master sergeant, not at the higher rank of command sergeant major. Walz served at the higher rank but left before completing all the extra training necessary for the rank.
Military records
In response to a Star Tribune request, Walzās 2022 campaign provided a two-page military record confirming the dates and his ranks. The Minnesota National Guard also confirmed the outlines of his tenure, saying that Walz served from April 8, 1981, until May 16, 2005.
Related Coverage
āWalz attained the rank of command sergeant major and served in that role but retired as a master sergeant in 2005 for benefit purposes due to not completing additional coursework,ā according to the statement from Army public affairs officer Lt. Col. Kristen AugĆ©.
Walz critics
In the 2022 gubernatorial race, Walzās Republican opponent, former state Sen. Scott Jensen, stood with a few veterans to accuse Walz of leaving the National Guard shortly before the battalion he led was deployed to Iraq.
Jensen, a physician who narrowly avoided the Vietnam-era draft, said in 2022 that Walzās departure from the Guard fits a pattern and āis just one of a long line of instances ⦠where Tim Walz failed to lead and ran from his duty.ā
During the gubernatorial campaign, Walz made no apologies for the timing of his departure, saying his life has been about public service, including his time in the military.
āWe all do what we can. Iām proud I did 24 years,ā Walz said. āI have an honorable record.ā
In his Minnesota campaigns and during his tenure as governor, Walz hasnāt spent much time talking about his time in the National Guard, more often touting his background as a high school teacher and football coach.
Joseph Eustice, a 32-year veteran of the Guard who led the same battalion as Walz, said the governor fulfilled his duty.
āHe was a great soldier,ā Eustice said. āWhen he chose to leave, he had every right to leave.ā
Eustice said claims to the contrary are ill-informed and possibly sour grapes by a soldier who was passed over for the promotion to command sergeant major that went to Walz.
That man, Thomas Behrends, was among those standing with Jensen to criticize the governor. Jensen and Behrends, a longtime critic of the governor, argue that Walz bailed on his troops when the going was about to get tough.
Walz defenders
Eustice, an Ortonville, Minn., teacher who describes himself as nonpartisan, said he unequivocally supports the governorās version of events.
Eustice said he recalled talking to Walz in 2005 when they were at Camp Ripley. He said Walz told him he was thinking about resigning from the Guard and running for Congress.
āThe man did nothing wrong with when he chose to leave the service; he didnāt break any rules,ā he said.
Like Walz, Eustice said that he also left in the middle of a six-year re-enrollment because members are free to leave at any time after their initial six-year stint.
āIf you choose to re-up, you can walk in any day and be done,ā Eustice said.
Jumping to Walzās defense in light of the Vance criticism was U.S. Sen. Mark Kelly of Arizona, a Navy combat veteran. He asked Vance if heād forgotten what the Marines taught about respect.
āTim Walz spent DECADES in uniform. You both deserve to be thanked for your service. Donāt become Donald Trump. He calls veterans suckers and losers and that is beneath those of us who have actually served,ā Kelly wrote on X, formerly Twitter, on Wednesday.
Former U.S. Rep. Adam Kinzinger, R-Ill. and an Air National Guard pilot, also defended Walz, saying the GOP is trying to āswiftboatā Walz. Swiftboat is a reference to the attacks questioning the Vietnam combat service of U.S. Sen. John Kerry when he was the Democratic presidential nominee in 2004.
āTim Walz joined the Army Guard and served honorably for 24 years, achieving the highest enlisted rank offered. That is quite an accomplishment. The nation should be proud, and JD Vance should be respectful of his fellow warrior,ā Kinzinger wrote on his Substack .
In 2022, Walz responded to the criticism from Jensen and Behrends at a Medal of Honor Memorial dedication on the State Capitol grounds, saying he was proud of his tenure in the Guard.
āI donāt know if Tom just disagrees with my politics or whatever, but my record speaks for itself and my accomplishments in uniform speak for itself, and thereās many people in this crowd, too, that I served with,ā Walz said. āItās just unfortunate.ā
The Associated Press contributed to this report.
Rochelle Olson
Rochelle Olson is a reporter on the politics and government team.
More from Elections
Tim walz agrees to an oct. 1 vice presidential debate on cbs. jd vance hasnāt responded.
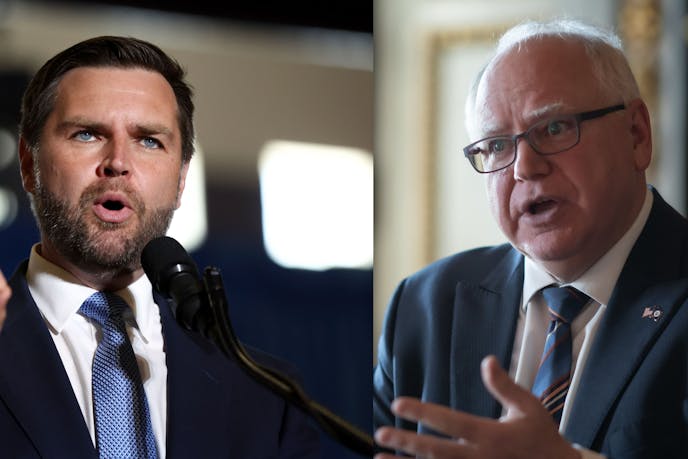
CBS News announced it had invited both Walz and Vance to a debate in New York City, and offered four date options.
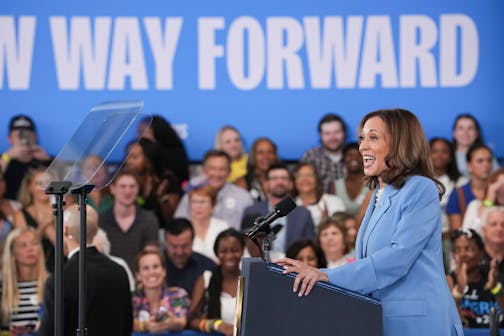
Harris offers proposals to cut food and housing costs, trying to blunt Trump's economic attacks
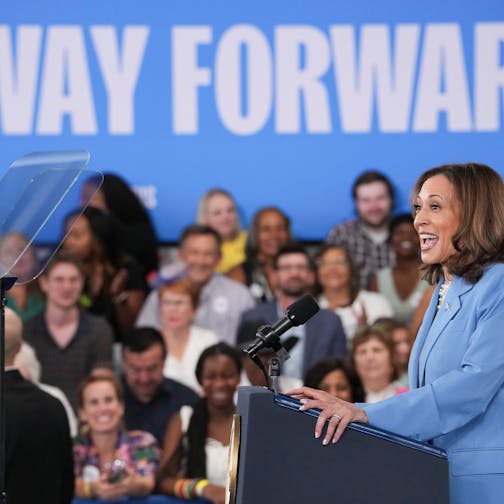
A chameleon or pragmatist? Minnesota Gov. Tim Walzās political evolution in the spotlight in VP race
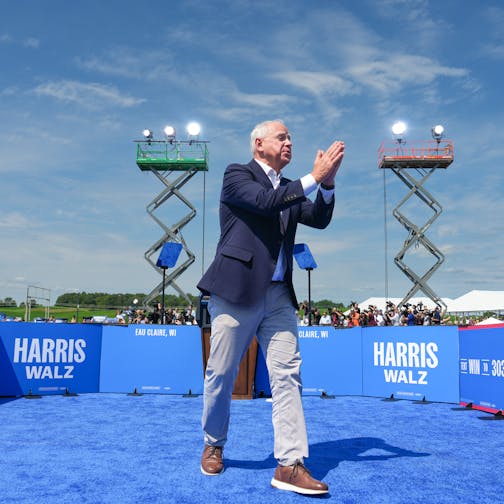
The conditional colour-magnitude distribution: II. A comparison of galaxy colour and luminosity distribution in galaxy groups
- Zheng, Zheng
- Yang, Xiaohu
- Li, Qingyang
The Conditional Colour-Magnitude Distribution (CCMD) is a comprehensive formalism of the colour-magnitude-halo mass relation of galaxies. With joint modelling of a large sample of SDSS galaxies in fine bins of galaxy colour and luminosity, Xu et al. inferred parameters of a CCMD model that well reproduces colour- and luminosity-dependent abundance and clustering of present-day galaxies. In this work, we provide a test and investigation of the CCMD model by studying the colour and luminosity distribution of galaxies in galaxy groups. An apples-to-apples comparison of group galaxies is achieved by applying the same galaxy group finder to identify groups from the CCMD galaxy mocks and from the SDSS data, avoiding any systematic effect of group finding and mass assignment on the comparison. We find an overall nice agreement in the conditional luminosity function (CLF), the conditional colour function (CCF), and the CCMD of galaxies in galaxy groups inferred from CCMD mock and SDSS data. We also discuss the subtle differences revealed by the comparison. In addition, using two external catalogues constructed to only include central galaxies with halo mass measured through weak lensing, we find that their colour-magnitude distribution shows two distinct and orthogonal components, in line with the prediction of the CCMD model. Our results suggest that the CCMD model provides a good description of halo mass dependent galaxy colour and luminosity distribution. The halo and CCMD mock catalogues are made publicly available to facilitate other investigations.
- Astrophysics - Astrophysics of Galaxies;
- Astrophysics - Cosmology and Nongalactic Astrophysics
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives⢠on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
When would you want to assign a variable in an if condition? [duplicate]
I recently just lost some time figuring out a bug in my code which was caused by a typo:
instead of:
I was wondering if there is any particular case you would want to assign a value to a variable in a if statement, or if not, why doesn't the compiler throw a warning or an error?
- if-statement

- 4 I depends, my compiler throws a warning when a = b – user1944441 Commented Jul 16, 2013 at 16:03
- 3 The compiler doesn't issue a diagnostic because it is not mandated to do so. It is perfectly valid C++ code. – Alok Save Commented Jul 16, 2013 at 16:05
- 7 Most compilers will warn about this, as long as you enable the warning. – Mike Seymour Commented Jul 16, 2013 at 16:07
- 3 I vote against treating this as a duplicate of stackoverflow.com/q/151850/2932052 , because this is an actual C/C++ question that arises often whereas the other is very vague and language-agnostic. Maybe it's really only a C/C++ problem? In this case the other should get the tag and this question should be treated as duplicate. – Wolf Commented Apr 23, 2019 at 9:27
- 2 Does this answer your question? Inadvertent use of = instead of == – outis Commented Jun 30, 2022 at 5:42
9 Answers 9
Though this is oft cited as an anti-pattern ("use virtual dispatch!"), sometimes the Derived type has functionality that the Base simply does not (and, consequently, distinct functions), and this is a good way to switch on that semantic difference.

- 17 The anti-pattern is putting a definition in the condition. It's a sure recipe for unreadable and unmaintainable code. – James Kanze Commented Jul 16, 2013 at 16:25
- 75 Putting the definition in the condition tightens the scope so that you don't accidentally refer to derived on the next line after the if-statement. It's the same reason we use for (int i = 0... instead of int i; for (i = 0; ... . – Adrian McCarthy Commented Jul 16, 2013 at 16:36
- 61 @JamesKanze - Others find it increases readability and maintainability, partly by minimizing the scope of the variable. "To avoid accidental misuse of a variable, it is usually a good idea to introduce the variable into the smallest scope possible. In particular, it is usually best to delay the definition of a variable until one can give it an initial value ... One of the most elegant applications of these two principles is to declare a variable in a conditional." -- Stroustrup, "The C++ Programming Language." – Andy Thomas Commented Jul 16, 2013 at 16:40
- 13 @James: Nobody in my team has had a problem either reading or maintaining the code, in the ten years our codebase has taken this approach. – Lightness Races in Orbit Commented Jul 16, 2013 at 17:42
- 15 Funny thing about the scope of the variable when putting a definition in the condition is that the variable is also accessible in the else -clause as well. if (T t = foo()) { dosomething(t); } else { dosomethingelse(t); } – dalle Commented Jul 18, 2013 at 8:57
Here is some history on the syntax in question.
In classical C, error handling was frequently done by writing something like:
Or, whenever there was a function call that might return a null pointer, the idiom was used the other way round:
However, this syntax is dangerously close to
which is why many people consider the assignment inside a condition bad style, and compilers started to warn about it (at least with -Wall ). Some compilers allow avoiding this warning by adding an extra set of parentheses:
However, this is ugly and somewhat of a hack, so it's better avoid writing such code.
Then C99 came around, allowing you to mix definitions and statements, so many developers would frequently write something like
which does feel awkward. This is why the newest standard allows definitions inside conditions, to provide a short, elegant way to do this:
There isn't any danger in this statement anymore. You explicitly give the variable a type, obviously wanting it to be initialized. It also avoids the extra line to define the variable, which is nice. But most importantly, the compiler can now easily catch this sort of bug:
Without the variable definition inside the if statement, this condition would not be detectable.
To make a long answer short: The syntax in you question is the product of old C's simplicity and power, but it is evil, so compilers can warn about it. Since it is also a very useful way to express a common problem, there is now a very concise, bug robust way to achieve the same behavior. And there are a lot of good, possible uses for it.

- 1 But adding extra parenthesis is not "bug robust": approxion.com/a-gcc-compiler-mistake The MISRA-compliant code if ((a == b) && (c = d)) won't generate a warning or error because of GCC's "feature". Instead of "bug robust" it's perniciously buggy , You think using -Wall -Werror protects you from such bugs, but it actually doesn't . – Andrew Henle Commented Jun 6, 2021 at 12:12
- 2 @AndrewHenle Well, that part about assignment in extra brackets was only to illustrate what has been done many times in real existing code. Of course , the extra parentheses are only a hack. Of course , they look ugly. Of course , this is just an extra reason to prefer the C++ variable declaration over a C assignment in an if() statement. You may question the sensibility of GCC's warning behavior, but that has no impact on my answer at all. – cmaster - reinstate monica Commented Jun 6, 2021 at 13:16
- 1 The issue isn't with your answer - I think it's about the best possible factual discussion of the history of the "assignment in an if-statement" style came about. The problem is your answer was referred by someone who utterly missed your characterization of GCC's hack and actually used your answer as a defense of that hack. I was pointing out how GCC's hack actually opens the door to even more subtle bugs when more complex code is involved. That evil aspect of the GCC "extra parentheses" hack seems to be utterly missed by proponents of cramming assignments into if statements. – Andrew Henle Commented Jun 6, 2021 at 16:12
- 3 @AndrewHenle Thanks for the explanation. It gets me thinking that it might be beneficial to point out that this is a hack, and that I discourage using it. – cmaster - reinstate monica Commented Jun 6, 2021 at 18:44
The assignment operator returns the value of the assigned value . So, I might use it in a situation like this:
I assign x to be the value returned by getMyNumber and I check if it's not zero.
Avoid doing that. I gave you an example just to help you understand this.
To avoid such bugs up to some extent, one should write the if condition as if(NULL == ptr) instead of if (ptr == NULL) . Because when you misspell the equality check operator == as operator = , the compiler will throw an lvalue error with if (NULL = ptr) , but if (res = NULL) passed by the compiler (which is not what you mean) and remain a bug in code for runtime.
One should also read Criticism regarding this kind of code.

- 14 I want to learn/improve myself, please mention why you downvote. – Maroun Commented Jul 16, 2013 at 16:16
- 2 I frequently use if(fp = fopen("file", "w")){ // file not open} else{ // file processing } – Grijesh Chauhan Commented Jul 16, 2013 at 19:00
- 18 Reversing the operands of an equality comparison, such as writing (NULL == ptr) rather than (ptr == NULL) , is controversial. Personally, I hate it; I find it jarring, and I have to mentally re-reverse it to understand it. (Others obviously don't have that issue). The practice is referred to as "Yoda conditions" . Most compilers can be persuaded to warn about assignments in conditions anyway. – Keith Thompson Commented Jul 16, 2013 at 19:30
- 1 I think its nice to know the trick! I added as an information, Some people may like it. That is why its just a suggestion. – Grijesh Chauhan Commented Jul 16, 2013 at 20:01
- 1 @LilianA.Moraru For some it is helpful. That's why it's a suggestion, if you don't like it you don't have to use it. – Maroun Commented Oct 17, 2017 at 8:36
why doesn't the compiler throw a warning
Some compilers will generate warnings for suspicious assignments in a conditional expression, though you usually have to enable the warning explicitly.
For example, in Visual C++ , you have to enable C4706 (or level 4 warnings in general). I generally turn on as many warnings as I can and make the code more explicit in order to avoid false positives. For example, if I really wanted to do this:
Then I'd write it as:
The compiler sees the explicit test and assumes that the assignment was intentional, so you don't get a false positive warning here.
The only drawback with this approach is that you can't use it when the variable is declared in the condition. That is, you cannot rewrite:
Syntactically, that doesn't work. So you either have to disable the warning, or compromise on how tightly you scope x .
C++17 added the ability to have an init-statement in the condition for an if statement ( p0305r1 ), which solves this problem nicely (for kind of comparison, not just != 0 ).
Furthermore, if you want, you can limit the scope of x to just the if statement:

- The explicit comparison if((x = Foo()) != 0) is unnecessary: adding an extra pair of parentheses if((x = Foo())) will silence the warning just as well. Also, there is a big difference between if(x = Foo()) and if(int x = Foo()) , the later clearly states that you want to do an initialization, not a comparison, so no compiler in their right mind would want to throw a warning on this. – cmaster - reinstate monica Commented Aug 26, 2013 at 17:35
- 2 To be fair, you don't have to compromise how tightly you scope x if you are willing to surround your block with extra braces. But I wager this is not usually worth it. – Thomas Eding Commented Aug 26, 2013 at 18:30
- 2 @cmaster: Explicit comparison is necessary if you want to check something other than != 0 . Explicit comparison is also clearer than an extra set of parentheses. – Adrian McCarthy Commented Aug 26, 2013 at 20:33
- 1 @AdrianMcCarthy: Explicit comparison also requires that extra set of parentheses (due to operator precedence). So the addition of ` != 0` is always just extra code. Of course, you are right that explicit comparison can compare to anything. If you are comparing against 0, however, I heartily disagree with you: This is such a common idiom that I expect the shorter version in that case, so that the extra ` != 0` has the effect of a small pothole to me... – cmaster - reinstate monica Commented Aug 26, 2013 at 20:53
- 1 @cmaster: That may be the case for you. But other programmers may be confused and think the extra parentheses are superfluous. They could delete them (which might not bother the compiler if they haven't enabled the same warning). Then someone will come along and think the assignment was supposed to be == , and now you've got two bugs. The explicit comparison makes it obvious clear why the extra parentheses are there and, more importantly, that the assignment was not a typo. – Adrian McCarthy Commented Mar 27, 2019 at 12:11
In C++17 , one can use:
Similar to a for loop iterator initializer.
Here is an example:

It depends on whether you want to write clean code or not. When C was first being developed, the importance of clean code wasn't fully recognized, and compilers were very simplistic: using nested assignment like this could often result in faster code. Today, I can't think of any case where a good programmer would do it. It just makes the code less readable and more difficult to maintain.
- 1 I agree that it's less readable but only if the assignment is the only thing in the if statement. If it's something like if ((x = function()) > 0) then I think that's perfectly fine. – ashishduh Commented Mar 24, 2014 at 19:29
- 2 @user1199931 Not really. If you're scanning the code to get a general understanding of it, you'll see the if and continue, and miss the fact that there is a change of state. There are exceptions ( for , but the keyword itself says that it is both looping and managing the loop state), but in general, a line of code should do one, and only one thing. If it controls flow, it shouldn't modify state. – James Kanze Commented Mar 26, 2014 at 13:41
- 3 "Clean code" is a very subjective term, unfortunately. – Michael Warner Commented Jan 11, 2016 at 22:01
Suppose you want to check several conditions in a single if , and if any one of the conditions is true, you'd like to generate an error message. If you want to include in your error message which specific condition caused the error, you could do the following:
So if the second condition is the one that evaluates to true, e will be equal to "cd". This is due to the short-circuit behaviour of || which is mandated by the standard (unless overloaded). See this answer for more details on short-circuiting.
- Interesting, but isn't it also for showing cleverness? – Wolf Commented Apr 23, 2019 at 9:50
- Using const char* e; instead of std::string e; would make it faster, but the similarity of the lines seems to ask for a loop over {"ab", "cd", "ef"}; . – Wolf Commented Oct 1, 2021 at 10:15
Doing assignment in an if is a fairly common thing, though it's also common that people do it by accident.
The usual pattern is:
The anti-pattern is where you're mistakenly assigning to things:
You can avoid this to a degree by putting your constants or const values first, so your compiler will throw an error:
= vs. == is something you'll need to develop an eye for. I usually put whitespace around the operator so it's more obvious which operation is being performed, as longname=longername looks a lot like longname==longername at a glance, but = and == on their own are obviously different.
- 10 They're both anti patterns. And as for if ( 1 == x ) , it's not the natural way of expressing the test, and any compiler worth its salt will warn for if ( x = 1 ) , so there's really no reason for being ugly here either. – James Kanze Commented Jul 16, 2013 at 16:10
- 1 The 1 == x pattern, anti or not, comes from Code Complete so you'll see it fairly often. It's a bit ugly, but might be a reasonable requirement for people that have a problem getting this right, serving as training wheels. – tadman Commented Jul 16, 2013 at 16:17
- 3 @tadman The way to teach people is by annoying them with warnings about this typo, not to switch to yoda conditions. – stefan Commented Jul 16, 2013 at 16:18
- 2 @stefan Especially as most compilers have an option to turn warnings into errors. – James Kanze Commented Jul 16, 2013 at 16:21
- @tadman: And reasonable compiler warnings catch more instances of this mistake than Yoda conditions do. And Yoda conditions only help when you remember to use them. – Adrian McCarthy Commented Jul 16, 2013 at 16:22
a quite common case. use
this kind of typo will cause compile error
Not the answer you're looking for? Browse other questions tagged c++ if-statement or ask your own question .
- The Overflow Blog
- Scaling systems to manage all the metadata ABOUT the data
- Navigating cities of code with Norris Numbers
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Feedback requested: How do you use tag hover descriptions for curating and do...
Hot Network Questions
- Power line crossing data lines via the ground plane
- Why does editing '/etc/shells' file using 'sudo open' shows an error saying I don't own the file?
- A short story about a demon, in a modernising Japan, living in electric wires and starting fires
- Non-linear recurrence for rational sequences with generating function with radicals?
- Has the application of a law ever being appealed anywhere due to the lawmakers not knowing what they were voting/ruling?
- Cumulative Addition of Index Switch Node Values
- How would a culture living in an extremely vertical environment deal with dead bodies?
- Cover letter format in Germany
- If Venus had a sapient civilisation similar to our own prior to global resurfacing, would we know it?
- How to prevent erasing buffer content when an external program used in filter fails?
- Did the Space Shuttle weigh itself before deorbit?
- A short story where a space pilot has a device that sends the ship back in time just before losing a space battle. He is duplicated by accident
- How come I was allowed to bring 1.5 liters of liquid through security at Frankfurt Airport?
- Does Gimp do batch processing?
- Get the first character of a string or macro
- Would several years of appointment as a lecturer hurt you when you decide to go for a tenure-track position later on?
- With 42 supernovae in 37 galaxies, how do we know SH0ES results is robust?
- Age is just a number!
- Do "Whenever X becomes the target of a spell" abilities get triggered by counterspell?
- Is an invalid date considered the same as a NULL value?
- Wiring 2 generators and utilizing a subpanel
- A post-apocalyptic short story where very sick people from our future save people in our timeline that would be killed in plane crashes
- Splitting an infinite sum in two parts results in a different result
- Erase the loops

IMAGES
COMMENTS
Python Conditional Assignment When you want to assign a value to a variable based on some condition, like if the condition is true then assign a value to the variable, else assign some other value to the variable, then you can use the conditional assignment operator.
Best way to do conditional assignment in python Asked 13 years, 1 month ago Modified 2 years, 7 months ago Viewed 24k times
In this step-by-step tutorial you'll learn how to work with conditional ("if") statements in Python. Master if-statements and see how to write complex decision making code in your programs.
Problem: How to perform one-line if conditional assignments in Python? Example: Say, you start with the following code.
Lean how to create a Pandas conditional column use Pandas apply, map, loc, and numpy select in order to use values of one or more columns.
In this tutorial, you'll learn how to use Python's assignment operators to write assignment statements that allow you to create, initialize, and update variables in your code.
The conditional operator ?:, also known as the ternary conditional operator, evaluates a Boolean expression and returns the result of one of the two expressions, depending on whether the Boolean expression evaluates to true or false, as the following example shows:
Conditional branching: if, '?' Sometimes, we need to perform different actions based on different conditions. To do that, we can use the if statement and the conditional operator ?, that's also called a "question mark" operator.
Verilog conditional assignments without using procedural blocks like VHDL with/select Ask Question Asked 3 years, 4 months ago Modified 3 years, 4 months ago Viewed 1k times
Conditional statements in programming are used to control the flow of a program based on certain conditions. These statements allow the execution of different code blocks depending on whether a specified condition evaluates to true or false, providing a fundamental mechanism for decision-making in algorithms. In this article, we will learn about the basics of Conditional Statements along with ...
Python conditional assignment operator Asked 13 years, 1 month ago Modified 2 years, 7 months ago Viewed 191k times
The question mark is known in Verilog as a conditional operator though in other programming languages it also is referred to as a ternary operator, an inline if, or a ternary if. It is used as a short-hand way to write a conditional expression in Verilog (rather than using if/else statements). Let's look at how it is used: condition ? value_if_true : value_if_false
Here are some of the advantages of using conditional operators: Concise syntax: The conditional operator allows for a compact and concise representation of conditional assignments. It reduces the amount of code needed compared to using if-else statements or case statements.
5 ways to apply an IF condition in Pandas DataFrame In this guide, you'll see 5 different ways to apply an IF condition in Pandas DataFrame.
The conditional operator in C is kind of similar to the if-else statement as it follows the same algorithm as of if-else statement but the conditional operator takes less space and helps to write the if-else statements in the shortest way possible. It is also known as the ternary operator in C as it operates on three operands.
Here is a a simplified use of the conditional assignment operator to illustrate the definition: a ||= b. If a is false, nil or undefined, then evaluate b and set a to the result. This seems to ...
For putting a conditional statement on one line, you could use the ternary operator. Note, however, that this can only be used in assignments, i.e. if the functions actually return something and you want to assign that value to a variable.
Conditional assignment Ruby is heavily influenced by lisp. One piece of obvious inspiration in its design is that conditional operators return values. This makes assignment based on conditions quite clean and simple to read.
Assigned Tasks This assignment uses only a testbench simulation, with no module to implement. Open the file src/testbench.v and examine how it is organized. It uses the conditional operator in an always block to assign q = a^b (XOR) when enabled, else q=0.
GOP vice presidential candidate JD Vance claims that the Minnesota governor and Democratic vice presidential candidate bailed as his unit headed to Iraq, but Walz retired before his unit was ...
If the SELECT statement returns more than one value, the variable is assigned the last value that is returned. As you may have noticed, SQL uses = for assignment, comparison and introducing aliases. Which one is determined by the position that the = appears in. In the WHERE clause, = can only be comparison. edited Jul 25, 2013 at 12:31.
The Conditional Colour-Magnitude Distribution (CCMD) is a comprehensive formalism of the colour-magnitude-halo mass relation of galaxies. With joint modelling of a large sample of SDSS galaxies in fine bins of galaxy colour and luminosity, Xu et al. inferred parameters of a CCMD model that well reproduces colour- and luminosity-dependent abundance and clustering of present-day galaxies. In ...
which is why many people consider the assignment inside a condition bad style, and compilers started to warn about it (at least with -Wall ). Some compilers allow avoiding this warning by adding an extra set of parentheses: