C Programming Tutorial
- Character Array and Character Pointer in C
Last updated on July 27, 2020
In this chapter, we will study the difference between character array and character pointer. Consider the following example:
char arr[] = "Hello World"; // array version char ptr* = "Hello World"; // pointer version |
Can you point out similarities or differences between them?
The similarity is:
The type of both the variables is a pointer to char or (char*) , so you can pass either of them to a function whose formal argument accepts an array of characters or a character pointer.
Here are the differences:
arr is an array of 12 characters. When compiler sees the statement:
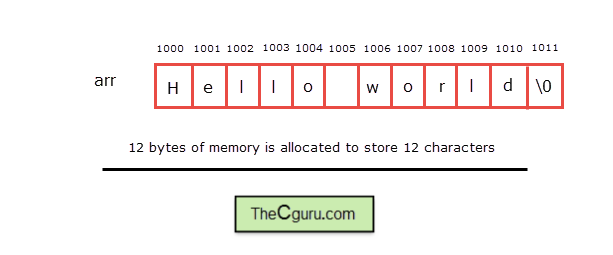
On the other hand when the compiler sees the statement.
It allocates 12 consecutive bytes for string literal "Hello World" and 4 extra bytes for pointer variable ptr . And assigns the address of the string literal to ptr . So, in this case, a total of 16 bytes are allocated.
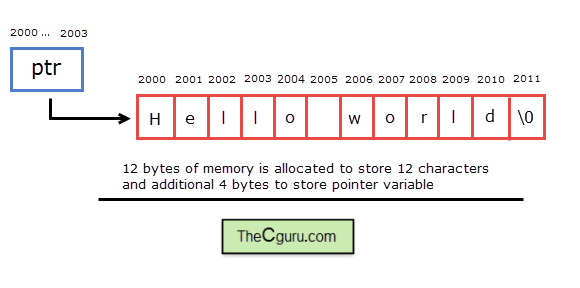
We already learned that name of the array is a constant pointer. So if arr points to the address 2000 , until the program ends it will always point to the address 2000 , we can't change its address. This means string assignment is not valid for strings defined as arrays.
On the contrary, ptr is a pointer variable of type char , so it can take any other address. As a result string, assignments are valid for pointers.
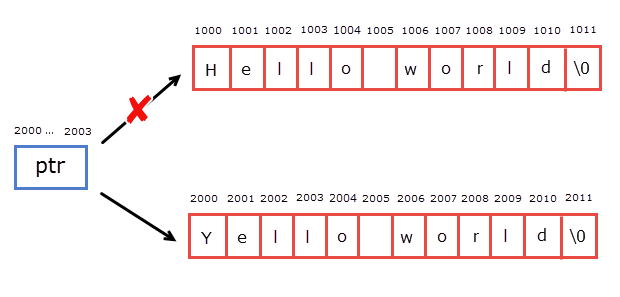
After the above assignment, ptr points to the address of "Yellow World" which is stored somewhere in the memory.
Obviously, the question arises so how do we assign a different string to arr ?
We can assign a new string to arr by using gets() , scanf() , strcpy() or by assigning characters one by one.
gets(arr); scanf("%s", arr); strcpy(arr, "new string"); arr[0] = 'R'; arr[1] = 'e'; arr[2] = 'd'; arr[3] = ' '; arr[4] = 'D'; arr[5] = 'r'; arr[6] = 'a'; arr[7] = 'g'; arr[8] = 'o'; arr[9] = 'n'; |
Recall that modifying a string literal causes undefined behavior, so the following operations are invalid.
char *ptr = "Hello"; ptr[0] = 'Y'; or *ptr = 'Y'; gets(name); scanf("%s", ptr); strcpy(ptr, "source"); strcat(ptr, "second string"); |
Using an uninitialized pointer may also lead to undefined undefined behavior.
Here ptr is uninitialized an contains garbage value. So the following operations are invalid.
ptr[0] = 'H'; gets(ptr); scanf("%s", ptr); strcpy(ptr, "source"); strcat(ptr, "second string"); |
We can only use ptr only if it points to a valid memory location.
char str[10]; char *p = str; |
Now all the operations mentioned above are valid. Another way we can use ptr is by allocation memory dynamically using malloc() or calloc() functions.
char *ptr; ptr = (char*)malloc(10*sizeof(char)); // allocate memory to store 10 characters |
Let's conclude this chapter by creating dynamic 1-d array of characters.
#include<stdio.h> #include<stdlib.h> int main() { int n, i; char *ptr; printf("Enter number of characters to store: "); scanf("%d", &n); ptr = (char*)malloc(n*sizeof(char)); for(i=0; i < n; i++) { printf("Enter ptr[%d]: ", i); /* notice the space preceding %c is necessary to read all whitespace in the input buffer */ scanf(" %c", ptr+i); } printf("\nPrinting elements of 1-D array: \n\n"); for(i = 0; i < n; i++) { printf("%c ", ptr[i]); } // signal to operating system program ran fine return 0; } |
Expected Output:
Enter number of characters to store: 6 Enter ptr[0]: a Enter ptr[1]: b Enter ptr[2]: c Enter ptr[3]: d Enter ptr[4]: y Enter ptr[5]: z Printing elements of 1-D array: a b c d y z |
Load Comments
- Intro to C Programming
- Installing Code Blocks
- Creating and Running The First C Program
- Basic Elements of a C Program
- Keywords and Identifiers
- Data Types in C
- Constants in C
- Variables in C
- Input and Output in C
- Formatted Input and Output in C
- Arithmetic Operators in C
- Operator Precedence and Associativity in C
- Assignment Operator in C
- Increment and Decrement Operators in C
- Relational Operators in C
- Logical Operators in C
- Conditional Operator, Comma operator and sizeof() operator in C
- Implicit Type Conversion in C
- Explicit Type Conversion in C
- if-else statements in C
- The while loop in C
- The do while loop in C
- The for loop in C
- The Infinite Loop in C
- The break and continue statement in C
- The Switch statement in C
- Function basics in C
- The return statement in C
- Actual and Formal arguments in C
- Local, Global and Static variables in C
- Recursive Function in C
- One dimensional Array in C
- One Dimensional Array and Function in C
- Two Dimensional Array in C
- Pointer Basics in C
- Pointer Arithmetic in C
- Pointers and 1-D arrays
- Pointers and 2-D arrays
- Call by Value and Call by Reference in C
- Returning more than one value from function in C
- Returning a Pointer from a Function in C
- Passing 1-D Array to a Function in C
- Passing 2-D Array to a Function in C
- Array of Pointers in C
- Void Pointers in C
- The malloc() Function in C
- The calloc() Function in C
- The realloc() Function in C
- String Basics in C
- The strlen() Function in C
- The strcmp() Function in C
- The strcpy() Function in C
- The strcat() Function in C
- Array of Strings in C
- Array of Pointers to Strings in C
- The sprintf() Function in C
- The sscanf() Function in C
- Structure Basics in C
- Array of Structures in C
- Array as Member of Structure in C
- Nested Structures in C
- Pointer to a Structure in C
- Pointers as Structure Member in C
- Structures and Functions in C
- Union Basics in C
- typedef statement in C
- Basics of File Handling in C
- fputc() Function in C
- fgetc() Function in C
- fputs() Function in C
- fgets() Function in C
- fprintf() Function in C
- fscanf() Function in C
- fwrite() Function in C
- fread() Function in C

Recent Posts
- Machine Learning Experts You Should Be Following Online
- 4 Ways to Prepare for the AP Computer Science A Exam
- Finance Assignment Online Help for the Busy and Tired Students: Get Help from Experts
- Top 9 Machine Learning Algorithms for Data Scientists
- Data Science Learning Path or Steps to become a data scientist Final
- Enable Edit Button in Shutter In Linux Mint 19 and Ubuntu 18.04
- Python 3 time module
- Pygments Tutorial
- How to use Virtualenv?
- Installing MySQL (Windows, Linux and Mac)
- What is if __name__ == '__main__' in Python ?
- Installing GoAccess (A Real-time web log analyzer)
- Installing Isso


- C Programming Tutorial
- Basics of C
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Type Casting
- C - Booleans
- Constants and Literals in C
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- Operators in C
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- Decision Making in C
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- Functions in C
- C - Functions
- C - Main Function
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- Scope Rules in C
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- Arrays in C
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- Pointers in C
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- Strings in C
- C - Strings
- C - Array of Strings
- C - Special Characters
- C Structures and Unions
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Structure Padding and Packing
- C - Nested Structures
- C - Anonymous Structure and Union
- C - Bit Fields
- C - Typedef
- File Handling in C
- C - Input & Output
- C - File I/O (File Handling)
- C Preprocessors
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Header Files
- Memory Management in C
- C - Memory Management
- C - Memory Address
- C - Storage Classes
- Miscellaneous Topics
- C - Error Handling
- C - Variable Arguments
- C - Command Execution
- C - Math Functions
- C - Static Keyword
- C - Random Number Generation
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Cheat Sheet
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Character Pointers and Functions in C
What is a character pointer in c.
A character pointer stores the address of a character type or address of the first character of a character array ( string ). Character pointers are very useful when you are working to manipulate the strings.
There is no string data type in C. An array of "char" type is considered as a string. Hence, a pointer of a char type array represents a string. This char pointer can then be passed as an argument to a function for processing the string.
Declaring a Character Pointer
A character pointer points to a character or a character array. Thus, to declare a character pointer, use the following syntax:
Initializing a Character Pointer
After declaring a character pointer, you need to initialize it with the address of a character variable. If there is a character array, you can simply initialize the character pointer by providing the name of the character array or the address of the first elements of it.
Character Pointer of Character
The following is the syntax to initialize a character pointer of a character type:
Character Pointer of Character Array
The following is the syntax to initialize a character pointer of a character array (string):
Character Pointer Example
In the following example, we have two variables character and character array. We are taking two pointer variables to store the addresses of the character and character array, and then printing the values of the variables using the character pointers.
Run the code and check its output −
The library functions in the header file "string.h" processes the string with char pointer parameters.
Understanding Character Pointer
A string is declared as an array as follows −
The string is a NULL terminated array of characters. The last element in the above array is a NULL character (\0).
Declare a pointer of char type and assign it the address of the character at the 0th position −
Remember that the name of the array itself is the address of 0th element.
A string may be declared using a pointer instead of an array variable (no square brackets).
This causes the string to be stored in the memory, and its address stored in ptr . We can traverse the string by incrementing the ptr .
Accessing Character Array
If you print a character array using the %s format specifier, you can do it by using the name of the character pointer. But if you want to access each character of the character array, you have to use an asterisk ( * ) before the character pointer name and then increment it.
Here is the full program code −
Alternatively, pass ptr to printf() with %s format to print the string.
On running this code, you will get the same output −
Character Pointer Functions
The "string.h" header files defines a number of library functions that perform string processing such as finding the length of a string, copying a string and comparing two strings. These functions use char pointer arguments.
The strlen() Function
The strlen() function returns the length, i.e. the number of characters in a string. The prototype of strlen() function is as follows −
The following code shows how you can print the length of a string −
When you run this code, it will produce the following output −
Effectively, the strlen() function computes the string length as per the user-defined function str_len() as shown below −
The strcpy() Function
The assignment operator ( = ) is not used to assign a string value to a string variable, i.e., a char pointer. Instead, we need to use the strcpy() function with the following prototype −
The following example shows how you can use the strcpy() function −
The strcpy() function returns the pointer to the destination string ptr1 .
Internally, the strcpy() function implements the following logic in the user-defined str_cpy() function −
When you runt his code, it will produce the following output −
The function copies each character from the source string to the destination till the NULL character "\0" is reached. After the loop, it adds a "\0" character at the end of the destination array.
The strcmp() Function
The usual comparison operators (<, >, <=, >=, ==, and !=) are not allowed to be used for comparing two strings. Instead, we need to use strcmp() function from the "string.h" header file. The prototype of this function is as follows −
The strcmp() function has three possible return values −
- When both strings are found to be identical , it returns "0".
- When the first not-matching character in str1 has a greater ASCII value than the corresponding character in str2, the function returns a positive integer . It implies that str1 appears after str2 in alphabetical order, as in a dictionary.
- When the first not-matching character in str1 has a lesser ASCII value than the corresponding character in str2, the function returns a negative integer . It implies that str1 appears before str2 in alphabetical order, as in a dictionary.
The following example demonstrates how you can use the strcmp() function in a C program −
Change s1 to BACK and run the code again. Now, you will get the following output −
You can obtain a similar result using the user-defined function str_cmp() , as shown in the following code −
The str_cmp() function compares the characters at the same index in a string till the characters in either string are exhausted or the characters are equal.
At the time of detecting unequal characters at the same index, the difference in their ASCII values is returned. It returns "0" when the loop is terminated.
Pointers in C Explained – They're Not as Difficult as You Think
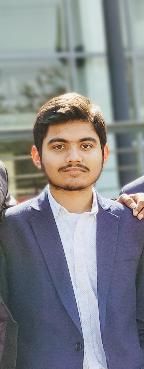
Pointers are arguably the most difficult feature of C to understand. But, they are one of the features which make C an excellent language.
In this article, we will go from the very basics of pointers to their usage with arrays, functions, and structure.
So relax, grab a coffee, and get ready to learn all about pointers.
A. Fundamentals
- What exactly are pointers?
- Definition and Notation
- Some Special Pointers
- Pointer Arithmetic
B. Arrays and Strings
- Why pointers and arrays?
- Array of Pointers
- Pointer to Array
C. Functions
- Call by Value v/s Call by Reference
- Pointers as Function Arguments
- Pointers as Function Return
- Pointer to Function
- Array Of Pointers to Functions
- Pointer to Function as an Argument
D. Structure
- Pointer to Structure
- Array of Structure
- Pointer to Structure as an Argument
E. Pointer to Pointer
F. conclusion, a. definition, notation, types and arithmetic, 1. what exactly are pointers.
Before we get to the definition of pointers, let us understand what happens when we write the following code:

A block of memory is reserved by the compiler to hold an int value. The name of this block is digit and the value stored in this block is 42 .
Now, to remember the block, it is assigned with an address or a location number (say, 24650).
The value of the location number is not important for us, as it is a random value. But, we can access this address using the & (ampersand) or address of operator.
We can get the value of the variable digit from its address using another operator * (asterisk), called the indirection or dereferencing or value at address operator.
2. Definition and Notation
The address of a variable can be stored in another variable known as a pointer variable. The syntax for storing a variable's address to a pointer is:
For our digit variable, this can be written like this:
or like this:
This can be read as - A pointer to int (integer) addressOfDigit stores the address of(&) digit variable.
Few points to understand:
dataType – We need to tell the computer what the data type of the variable is whose address we are going to store. Here, int was the data type of digit .
It does not mean that addressOfDigit will store a value of type int . An integer pointer (like addressOfDigit ) can only store the address of variables of integer type.
* – A pointer variable is a special variable in the sense that it is used to store an address of another variable. To differentiate it from other variables that do not store an address, we use * as a symbol in the declaration.
Here, we can assign the address of variable1 and variable2 to the integer pointer addressOfVariables but not to variable3 since it is of type char . We will need a character pointer variable to store its address.
We can use our addressOfDigit pointer variable to print the address and the value of digit as below:
Here, *addressOfDigit can be read as the value at the address stored in addressOfDigit .
Notice we used %d as the format identifier for addressOfDigit . Well, this is not completely correct. The correct identifier would be %p .
Using %p , the address is displayed as a hexadecimal value. But the memory address can be displayed in integers as well as octal values. Still, since it is not an entirely correct way, a warning is shown.
The output according to the compiler I'm using is the following:
This is the warning shown when you use %d - " warning: format '%d' expects argument of type 'int', but argument 2 has type 'int *' ".
3. Some Special Pointers
The wild pointer.
When we defined our character pointer alphabetAddress , we did not initialize it.
Such pointers are known as wild pointers . They store a garbage value (that is, memory address) of a byte that we don't know is reserved or not (remember int digit = 42; , we reserved a memory address when we declared it).
Suppose we dereference a wild pointer and assign a value to the memory address it is pointing at. This will lead to unexpected behaviour since we will write data at a memory block that may be free or reserved.
Null Pointer
To make sure that we do not have a wild pointer, we can initialize a pointer with a NULL value, making it a null pointer .
A null pointer points at nothing, or at a memory address that users can not access.
Void Pointer
A void pointer can be used to point at a variable of any data type. It can be reused to point at any data type we want to. It is declared like this:
Since they are very general in nature, they are also known as generic pointers .
With their flexibility, void pointers also bring some constraints. Void pointers cannot be dereferenced as any other pointer. Appropriate typecasting is necessary.
Similarly, void pointers need to be typecasted for performing arithmetic operations.
Void pointers are of great use in C. Library functions malloc() and calloc() which dynamically allocate memory return void pointers. qsort() , an inbuilt sorting function in C, has a function as its argument which itself takes void pointers as its argument.
Dangling Pointer
A dangling pointer points to a memory address which used to hold a variable. Since the address it points at is no longer reserved, using it will lead to unexpected results.
Though the memory has been deallocated by free(ptr) , the pointer to integer ptr still points to that unreserved memory address.
4. Pointer Arithmetic
We know by now that pointers are not like any other variable. They do not store any value but the address of memory blocks.
So it should be quite clear that not all arithmetic operations would be valid with them. Would multiplying or dividing two pointers ( having addresses ) make sense?
Pointers have few but immensely useful valid operations:
- You can assign the value of one pointer to another only if they are of the same type (unless they're typecasted or one of them is void * ).
2. You can only add or subtract integers to pointers.
When you add (or subtract) an integer (say n) to a pointer, you are not actually adding (or subtracting) n bytes to the pointer value. You are actually adding (or subtracting) n- times the size of the data type of the variable being pointed bytes.
The value stored in newAddress will not be 103, rather 112 .
3. Subtraction and comparison of pointers is valid only if both are members of the same array. The subtraction of pointers gives the number of elements separating them.
4. You can assign or compare a pointer with NULL .
The only exception to the above rules is that the address of the first memory block after the last element of an array follows pointer arithmetic.
Pointer and arrays exist together. These valid manipulations of pointers are immensely useful with arrays, which will be discussed in the next section.
1. Why pointers and arrays?
In C, pointers and arrays have quite a strong relationship.
The reason they should be discussed together is because what you can achieve with array notation ( arrayName[index] ) can also be achieved with pointers, but generally faster.
2. 1-D Arrays
Let us look at what happens when we write int myArray[5]; .
Five consecutive blocks of memory starting from myArray[0] to myArray[4] are created with garbage values in them. Each of the blocks is of size 4 bytes.
Thus, if the address of myArray[0] is 100 (say), the address of the rest of the blocks would be 104 , 108 , 112 , and 116 .
Have a look at the following code:
So, &prime , prime , and &prime[0] all give the same address, right? Well, wait and read because you are in for a surprise (and maybe some confusion).
Let's try to increment each of &prime , prime , and &prime[0] by 1.
Wait! How come &prime + 1 results in something different than the other two? And why are prime + 1 and &prime[0] + 1 still equal? Let's answer these questions.
prime and &prime[0] both point to the 0th element of the array prime . Thus, the name of an array is itself a pointer to the 0th element of the array .
Here, both point to the first element of size 4 bytes. When you add 1 to them, they now point to the 1st element in the array. Therefore this results in an increase in the address by 4.
&prime , on the other hand, is a pointer to an int array of size 5 . It stores the base address of the array prime[5] , which is equal to the address of the first element. However, an increase by 1 to it results in an address with an increase of 5 x 4 = 20 bytes.
In short, arrayName and &arrayName[0] point to the 0th element whereas &arrayName points to the whole array.
We can access the array elements using subscripted variables like this:
We can do the same using pointers which are always faster than using subscripts.
Both methods give the output:
Thus, &arrayName[i] and arrayName[i] are the same as arrayName + i and *(arrayName + i) , respectively.
3. 2-D Arrays
Two-dimensional arrays are an array of arrays.
Here, marks can be thought of as an array of 5 elements, each of which is a one-dimensional array containing 3 integers. Let us work through a series of programs to understand different subscripted expressions.
Like 1-D arrays, &marks points to the whole 2-D array, marks[5][3] . Thus, incrementing to it by 1 ( = 5 arrays X 3 integers each X 4 bytes = 60) results in an increment by 60 bytes.
If marks was a 1-D array, marks and &marks[0] would have pointed to the 0th element. For a 2-D array, elements are now 1-D arrays . Hence, marks and &marks[0] point to the 0th array (element), and the addition of 1 point to the 1st array.
And now comes the difference. For a 1-D array, marks[0] would give the value of the 0th element. An increment by 1 would increase the value by 1.
But, in a 2-D array, marks[0] points to the 0th element of the 0th array. Similarly, marks[1] points to the 0th element of the 1st array. An increment by 1 would point to the 1st element in the 1st array.
This is the new part. marks[i][j] gives the value of the jth element of the ith array. An increment to it changes the value stored at marks[i][j] . Now, let us try to write marks[i][j] in terms of pointers.
We know marks[i] + j would point to the ith element of the jth array from our previous discussion. Dereferencing it would mean the value at that address. Thus, marks[i][j] is the same as *(marks[i] + j) .
From our discussion on 1-D arrays, marks[i] is the same as *(marks + i) . Thus, marks[i][j] can be written as *(*(marks + i) + j) in terms of pointers.
Here is a summary of notations comparing 1-D and 2-D arrays.
Expression | 1-D Array | 2-D Array |
---|---|---|
&arrayName | points to the address of whole array adding 1 increases the address by 1 x sizeof(arrayName) | points to the address of whole array adding 1 increases the address by 1 x sizeof(arrayName) |
arrayName | points to the 0th element adding 1 increases the address to 1st element | points to the 0th element (array) adding 1 increases the address to 1st element (array) |
&arrayName[i] | points to the the ith element adding 1 increases the address to (i+1)th element | points to the ith element (array) adding 1 increases the address to the (i+1)th element (array) |
arrayName[i] | gives the value of the ith element adding 1 increases the value of the ith element | points to the 0th element of the ith array adding 1 increases the address to 1st element of the ith array |
arrayName[i][j] | Nothing | gives the value of the jth element of the ith array adding 1 increases the value of the jth element of the ith array |
Pointer Expression To Access The Elements | *( arrayName + i) | *( *( arrayName + i) + j) |
A string is a one-dimensional array of characters terminated by a null(\0) . When we write char name[] = "Srijan"; , each character occupies one byte of memory with the last one always being \0 .
Similar to the arrays we have seen, name and &name[0] points to the 0th character in the string, while &name points to the whole string. Also, name[i] can be written as *(name + i) .
A two-dimensional array of characters or an array of strings can also be accessed and manipulated as discussed before.
5. Array of Pointers
Like an array of int s and an array of char s, there is an array of pointers as well. Such an array would simply be a collection of addresses. Those addresses could point to individual variables or another array as well.
The syntax for declaring a pointer array is the following:
Following the operators precedence , the first example can be read as - example1 is an array( [] ) of 5 pointers to int . Similarly, example2 is an array of 8 pointers to char .
We can store the two-dimensional array to string top using a pointer array and save memory as well.
top will contain the base addresses of all the respective names. The base address of "Liverpool" will be stored in top[0] , "Man City" in top[1] , and so on.
In the earlier declaration, we required 90 bytes to store the names. Here, we only require ( 58 (sum of bytes of names) + 12 ( bytes required to store the address in the array) ) 70 bytes.
The manipulation of strings or integers becomes a lot easier when using an array of pointers.
If we try to put "Leicester" ahead of "Chelsea" , we just need to switch the values of top[3] and top[4] like below:
Without pointers, we would have to exchange every character of the strings, which would have taken more time. That's why strings are generally declared using pointers.
6. Pointer to Array
Like "pointer to int " or "pointer to char ", we have pointer to array as well. This pointer points to whole array rather than its elements.
Remember we discussed how &arrayName points to the whole array? Well, it is a pointer to array.
A pointer to array can be declared like this:
Notice the parentheses. Without them, these would be an array of pointers. The first example can be read as - ptr1 is a pointer to an array of 5 int (integers) .
When we dereference a pointer, it gives the value at that address. Similarly, by dereferencing a pointer to array, we get the array and the name of the array points to the base address. We can confirm that *pointerToGoals gives the array goals if we find its size.
If we dereference it again, we will get the value stored in that address. We can print all the elements using pointerToGoals .
Pointers and pointer to arrays are quite useful when paired up with functions. Coming up in the next section!
1. Call by Value vs Call by Reference
Have a look at the program below:
The function multiply() takes two int arguments and returns their product as int .
In the function call multiply(x,y) , we passed the value of x and y ( of main() ), which are actual arguments , to multiply() .
The values of the actual arguments are passed or copied to the formal arguments x and y ( of multiply() ). The x and y of multiply() are different from those of main() . This can be verified by printing their addresses.
Since we created stored values in a new location, it costs us memory. Wouldn't it be better if we could perform the same task without wasting space?
Call by reference helps us achieve this. We pass the address or reference of the variables to the function which does not create a copy. Using the dereferencing operator * , we can access the value stored at those addresses.
We can rewrite the above program using call by reference as well.
2. Pointers as Function Arguments
In this section, we will look at various programs where we give int , char , arrays and strings as arguments using pointers.
We created four functions, add() , subtract() , multiply() and divide() to perform arithmetic operations on the two numbers a and b .
The address of a and b was passed to the functions. Inside the function using * we accessed the values and printed the result.
Similarly, we can give arrays as arguments using a pointer to its first element.
Since the name of an array itself is a pointer to the first element, we send that as an argument to the function greatestOfAll() . In the function, we traverse through the array using loop and pointer.
Here, we pass in the string name to wish() using a pointer and print the message.
3. Pointers as Function Return
The function multiply() takes two pointers to int . It returns a pointer to int as well which stores the address where the product is stored.
It is very easy to think that the output would be 15. But it is not!
When multiply() is called, the execution of main() pauses and memory is now allocated for the execution of multiply() . After its execution is completed, the memory allocated to multiply() is deallocated.
Therefore, though c ( local to main() ) stores the address of the product, the data there is not guaranteed since that memory has been deallocated.
So does that mean pointers cannot be returned by a function? No!
We can do two things. Either store the address in the heap or global section or declare the variable to be static so that their values persist.
Static variables can simply be created by using the keyword static before data type while declaring the variable.
To store addresses in heap, we can use library functions malloc() and calloc() which allocate memory dynamically.
The following programs will explain both the methods. Both methods return the output as 15.
4. Pointer to Function
Like pointer to different data types, we also have a pointer to function as well.
A pointer to function or function pointer stores the address of the function. Though it doesn't point to any data. It points to the first instruction in the function.
The syntax for declaring a pointer to function is:
The below example will make it clearer.
The declaration for the pointer p to function multiply() can be read as ( following operator precedence ) - p is a pointer to function with two int eger pointers ( or two pointers to int ) as parameters and returning a pointer to int .
Since the name of the function is also a pointer to the function, the use of & is not necessary. Also removing * from the function call doesn't affect the program.
5. Array of Pointers to Functions
We have already seen how to create an array of pointers to int , char , and so on. Similarly, we can create an array of pointers to function.
In this array, every element will store an address of a function, where all the functions are of the same type. That is, they have the same type and number of parameters and return types.
We will modify a program discussed earlier in this section. We will store the addresses of add() , subtract() , multiply() and divide() in an array make a function call through subscript.
The declaration here can be read as - p is an array of pointer to functions with two float pointers as parameters and returning void .
6. Pointer to Function as an Argument
Like any other pointer, function pointers can also be passed to another function, therefore known as a callback function or called function . The function to which it is passed is known as a calling function .
A better way to understand would be to look at qsort() , which is an inbuilt function in C. It is used to sort an array of integers, strings, structures, and so on. The declaration for qsort() is:
qsort() takes four arguments:
- a void pointer to the start of an array
- number of elements
- size of each element
- a function pointer that takes in two void pointers as arguments and returns an int
The function pointer points to a comparison function that returns an integer that is greater than, equal to, or less than zero if the first argument is respectively greater than, equal to, or less than the second argument.
The following program showcases its usage:
Since a function name is itself a pointer, we can write compareIntegers as the fourth argument.
1. Pointer to Structure
Like integer pointers, array pointers, and function pointers, we have pointer to structures or structure pointers as well.
Here, we have declared a pointer ptrStudent of type struct records . We have assigned the address of student to ptrStudent .
ptrStudent stores the base address of student , which is the base address of the first member of the structure. Incrementing by 1 would increase the address by sizeof(student) bytes.
We can access the members of student using ptrStudent in two ways. Using our old friend * or using -> ( infix or arrow operator ).
With * , we will continue to use the . ( dot operator) whereas with -> we won't need the dot operator.
Similarly, we can access and modify other members as well. Note that the brackets are necessary while using * since the dot operator( . ) has higher precedence over * .
2. Array Of Structure
We can create an array of type struct records and use a pointer to access the elements and their members.
Note that ptrStudent1 is a pointer to student[0] whereas ptrStudent2 is a pointer to the whole array of 10 struct records . Adding 1 to ptrStudent1 would point to student[1] .
We can use ptrStudent1 with a loop to traverse through the elements and their members.
3. Pointer to Structure as an Argument
We can also pass the address of a structure variable to a function.
Note that the structure struct records is declared outside main() . This is to ensure that it is available globally and printRecords() can use it.
If the structure is defined inside main() , its scope will be limited to main() . Also structure must be declared before the function declaration as well.
Like structures, we can have pointers to unions and can access members using the arrow operator ( -> ).
So far we have looked at pointer to various primitive data types, arrays, strings, functions, structures, and unions.
The automatic question that comes to the mind is – what about pointer to pointer?
Well, good news for you! They too exist.
To store the address of int variable var , we have the pointer to int ptr_var . We would need another pointer to store the address of ptr_var .
Since ptr_var is of type int * , to store its address we would have to create a pointer to int * . The code below shows how this can be done.
We can use ptr_ptrvar to access the address of ptr_var and use double dereferencing to access var.
It is not required to use brackets when dereferencing ptr_ptrvar . But it is a good practice to use them. We can create another pointer ptr_ptrptrvar , which will store the address of ptr_ptrvar .
Since ptr_ptrvar is of type int** , the declaration for ptr_ptrptrvar will be
We can again access ptr_ptrvar , ptr_var and var using ptr_ptrptrvar .
If we change the value at any of the pointer(s) using ptr_ptrptrvar or ptr_ptrvar , the pointer(s) will stop pointing to the variable.
Phew! Yeah, we're finished. We started from pointers and ended with pointers (in a way). Don't they say that the curve of learning is a circle!
Try to recap all the sub-topics that you read. If you can recollect them, well done! Read the ones you can't remember again.
This article is done, but you shouldn't be done with pointers. Play with them. Next, you can look into Dynamic Memory Allocation to get to know pointers better .
Stay home, stay safe.
Sachin. Cricket. Dhoni. De Villiers. Buttler. In that order.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started

- Website Rank
- ASP.net Examples
- EMI Calculator

C Data Types
C arithmetic operators, c switch case, c function arguments, c functions returning pointers, c dynamic memory allocations, c array elements memory locations, c passing array to a function, c access array elements, c print string elements using pointer, c string functions, c string length, c string index, c escape sequences, c structures, nested structures, passing structure variable, c structure pointers, c array of structures, c file pointers, c text file write, c text file read, c binary file write, c binary file read, scanf character issue, pointers to function, command line arguments, multiple return values, implicit function declaration warning, c define directive, c include directive, c ifdef directive, undefined reference to pow, c gets function, c storage classes, switch case, conditional operator, reverse order for loop, for with multiple initializations, c programming pointers for int, float and char.
A pointer is a variable that holds the memory address of another variable (direct address of the memory location).
Every byte in the computer's memory has an address, so pointer holds the address through which variable can be directly accessed.
Addresses are just numbers as our house numbers, and address starts at NULL and goes up from 1,2, 3 etc..
Need to declare a pointer before using it to store any variable address.
Let us consider below decalration,
a is the integer variable and reserves the space in memory to hold integer value 25.
b is the integer pointer and can contain the address of integer variable a.
Value at address contained in b is an integer.
Similarly need to declare b as float pointer if needs to contain the address of float variable.
int, float, char Pointers in C Program
This c program explains how to use the pointers with int, float and character data types.
C Pointers Type Casting Example:
2 bytes used to store integer value and for character 1 bytes. during char * type casting, considers only first 1 byte of data and not another byte.
What will happen if we use incorrect pointer type?
Incorrect values (garbage values) returned for incorrect pointers.
Warning message is thrown as 'incompatible pointer type' in compilation.
C program to cmpute matrix addition using two dimensional array
C program to cmpute matrix subtraction using two dimensional array, c program to cmpute matrix multiplication using two dimensional array, c program to compute different order of matrix multiplication, c program to generate identity matrix for required rows and columns, c program to validate whether matrix is identity matrix or not, c program to validate whether matrix is sparse matrix or not, c program to validate whether matrix is dense matrix or not, c program to generate string as matrix diagonal characters using two dimesional array, c program to find number of weeks, months and years from days, c program to implement linked list and operations, c program to implement sorted linked list and operations, c program to reverse the linked list, c program to stack and operations using linked list, c program to queue and operations using linked list, c program to calculate multiplication of two numbers using pointers, c program to calculate median, c program to calculate standard deviation, c program for fahrenheit to celsius conversion, c program to calculate average, c program for quadratic equations, c program to check character type, c program to find largest of three values, c program to find max value in array, c program to find min value in array, c program to print multiplication table, c program for frequency counting, c program to read a line of text, c program to find ascii value for any character, c program to find a character is number, alphabet, operator, or special character, c program to find reverse case for any alphhabet using ctype functions, c program to find number of vowels in input string, c program pointers example code, c program to find leap year or not, c program to swap two integers using call by reference, c program to swap two integers without using third variable, c program to list prime numbers upto limit, c program to list composite numbers upto limit, c program to calculate compound interest, c program to calculate depreciation amount after of before few years, c program to calculate profit percentage, c program to calculate loss percentage, c program to find string is polindrome or not, c program to find factorial of a number, c program to check number is a polindrome or not, c program to generate random integers, c program to generate random float numbers, c program to find square root of a number, c program to find area of a rectangle, c program to find perimeter of a rectangle, c program to find area of a square, c program to find area of a triangle, c program to find area of a parallelogram, c program to find area of a rhombus, c program to find area of a trapezium, c program to find area of a circle and semi-circle, c program to find circumference of a circle and semi-circle, c program to find length of an arc, c program to find area of a sector, c program to find string length for list of strings, c program to find the character at index in a string, c program to compare characters, c program to find eligible to vote or not, c program to get system current date and time on linux, c program to get positive number, c program to implement calculator, c program to implement banking system, c program to find sum of number, c program for array traversal using pointers, c program for array traversal in reverse order using pointers, c program to find particular element occurrences count in array, c program to find even elements occurrences count in array, c program to find odd elements occurrences count in array, c program to find week day in word from week day in number using two dimentsional array, c program to find month in word from month in number using pointers, c program to compute pmt for a loan, c program to compute emi and round up using floor, c program to compute emi and round up using ceil, c program to compute the emi table with interest, principal and balance, c program to get multiple line of string, c program to find nth element in array using pointers, c program to compute the volume of a cube, c program to compute the volume of a box, c program to compute the volume of a sphere, c program to compute the volume of a triangular prism, c program to compute the volume of a cylinder, c program to compute perimeter of square, c program to compute the perimeter of triangle, c program to compute the discount rate, c program to compute the sales tax, c program to add, delete, search a record or show all using binary file, c program to add, delete, search a record or show all using text file.

cppreference.com
Pointer declaration.
(C11) | ||||
Miscellaneous | ||||
(C11) | ||||
(C23) |
Pointer is a type of an object that refers to a function or an object of another type, possibly adding qualifiers. Pointer may also refer to nothing, which is indicated by the special null pointer value.
Syntax Explanation Pointers to objects Pointers to functions Pointers to void Null pointers Notes References See also |
[ edit ] Syntax
In the declaration grammar of a pointer declaration, the type-specifier sequence designates the pointed-to type (which may be function or object type and may be incomplete), and the declarator has the form:
attr-spec-seq (optional) qualifiers (optional) declarator | |||||||||
where declarator may be the identifier that names the pointer being declared, including another pointer declarator (which would indicate a pointer to a pointer):
The qualifiers that appear between * and the identifier (or other nested declarator) qualify the type of the pointer that is being declared:
The attr-spec-seq (C23) is an optional list of attributes , applied to the declared pointer.
[ edit ] Explanation
Pointers are used for indirection, which is a ubiquitous programming technique; they can be used to implement pass-by-reference semantics, to access objects with dynamic storage duration , to implement "optional" types (using the null pointer value), aggregation relationship between structs, callbacks (using pointers to functions), generic interfaces (using pointers to void), and much more.
[ edit ] Pointers to objects
A pointer to object can be initialized with the result of the address-of operator applied to an expression of object type (which may be incomplete):
Pointers may appear as operands to the indirection operator (unary * ), which returns the lvalue identifying the pointed-to object:
Pointers to objects of struct and union type may also appear as the left-hand operands of the member access through pointer operator -> .
Because of the array-to-pointer implicit conversion, pointer to the first element of an array can be initialized with an expression of array type:
Certain addition, subtraction , compound assignment , increment, and decrement operators are defined for pointers to elements of arrays.
Comparison operators are defined for pointers to objects in some situations: two pointers that represent the same address compare equal, two null pointer values compare equal, pointers to elements of the same array compare the same as the array indices of those elements, and pointers to struct members compare in order of declaration of those members.
Many implementations also provide strict total ordering of pointers of random origin, e.g. if they are implemented as addresses within continuous ("flat") virtual address space.
[ edit ] Pointers to functions
A pointer to function can be initialized with an address of a function. Because of the function-to-pointer conversion, the address-of operator is optional:
Unlike functions, pointers to functions are objects and thus can be stored in arrays, copied, assigned, passed to other functions as arguments, etc.
A pointer to function can be used on the left-hand side of the function call operator ; this invokes the pointed-to function:
Dereferencing a function pointer yields the function designator for the pointed-to function:
Equality comparison operators are defined for pointers to functions (they compare equal if pointing to the same function).
Because compatibility of function types ignores top-level qualifiers of the function parameters, pointers to functions whose parameters only differ in their top-level qualifiers are interchangeable:
[ edit ] Pointers to void
Pointer to object of any type can be implicitly converted to pointer to void (optionally const or volatile -qualified), and vice versa:
Pointers to void are used to pass objects of unknown type, which is common in generic interfaces: malloc returns void * , qsort expects a user-provided callback that accepts two const void * arguments. pthread_create expects a user-provided callback that accepts and returns void * . In all cases, it is the caller's responsibility to convert the pointer to the correct type before use.
[ edit ] Null pointers
Pointers of every type have a special value known as null pointer value of that type. A pointer whose value is null does not point to an object or a function (dereferencing a null pointer is undefined behavior), and compares equal to all pointers of the same type whose value is also null .
To initialize a pointer to null or to assign the null value to an existing pointer, a null pointer constant ( NULL , or any other integer constant with the value zero) may be used. static initialization also initializes pointers to their null values.
Null pointers can indicate the absence of an object or can be used to indicate other types of error conditions. In general, a function that receives a pointer argument almost always needs to check if the value is null and handle that case differently (for example, free does nothing when a null pointer is passed).
[ edit ] Notes
Although any pointer to object can be cast to pointer to object of a different type, dereferencing a pointer to the type different from the declared type of the object is almost always undefined behavior. See strict aliasing for details.
It is possible to indicate to a function that accesses objects through pointers that those pointers do not alias. See for details. | (since C99) |
lvalue expressions of array type, when used in most contexts, undergo an implicit conversion to the pointer to the first element of the array. See array for details.
Pointers to char are often used to represent strings . To represent a valid byte string, a pointer must be pointing at a char that is an element of an array of char, and there must be a char with the value zero at some index greater or equal to the index of the element referenced by the pointer.
[ edit ] References
- C23 standard (ISO/IEC 9899:2024):
- 6.7.6.1 Pointer declarators (p: TBD)
- C17 standard (ISO/IEC 9899:2018):
- 6.7.6.1 Pointer declarators (p: 93-94)
- C11 standard (ISO/IEC 9899:2011):
- 6.7.6.1 Pointer declarators (p: 130)
- C99 standard (ISO/IEC 9899:1999):
- 6.7.5.1 Pointer declarators (p: 115-116)
- C89/C90 standard (ISO/IEC 9899:1990):
- 3.5.4.1 Pointer declarators

[ edit ] See also
for Pointer declaration |
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 9 April 2024, at 00:10.
- Privacy policy
- About cppreference.com
- Disclaimers

- Skip to main content
- Skip to primary sidebar
- Skip to secondary sidebar
- Skip to footer
Computer Notes
- Computer Fundamental
- Computer Memory
- DBMS Tutorial
- Operating System
- Computer Networking
- C Programming
- C++ Programming
- Java Programming
- C# Programming
- SQL Tutorial
- Management Tutorial
- Computer Graphics
- Compiler Design
- Style Sheet
- JavaScript Tutorial
- Html Tutorial
- Wordpress Tutorial
- Python Tutorial
- PHP Tutorial
- JSP Tutorial
- AngularJS Tutorial
- Data Structures
- E Commerce Tutorial
- Visual Basic
- Structs2 Tutorial
- Digital Electronics
- Internet Terms
- Servlet Tutorial
- Software Engineering
- Interviews Questions
- Basic Terms
- Troubleshooting
Header Right
How to pointer assignment and initialization in c.
By Dinesh Thakur
When we declare a pointer, it does not point to any specific variable. We must initialize it to point to the desired variable. This is achieved by assigning the address of that variable to the pointer variable, as shown below.
int a = 10;
pa = &a; /* pointer variable pa now points to variable a */
In this example, the first line declares an int variable named a and initializes it to 10. The second line declares a pointer pa of type pointer to int. Finally, the address of variable a is assigned to pa.Now pa is said to point to variable a.
We can also initialize a pointer when it is declared using the format given below.
type * ptr_var = init_expr ;
where init_expr is an expression that specifies the address of a previously defined variable of appropriate type or it can be NULL, a constant defined in the <stdio.h> header file.
Consider the example given below.
float x = 0.5;
float *px = &x;
int *p = NULL;
The second line declares a pointer variable px of type float * and initializes it with the address of variable x declared in the first line. Thus, pointer px now points to variable x. The third line declares pointer variable p of type int * and initializes it to NULL. Thus, pointer p does not point to any variable and it is an error to dereference such a pointer.
Note that a character pointer can be initialized using a character string constant as in
char *msg = “Hello, world!”;
Here, the C compiler allocates the required memory for the string constant (14 characters, in the above example, including the null terminator), stores the string constant in this memory and then assigns the initial address of this memory to pointer msg,as iliustrated in Fig.

The C language also permits initialization of more that one pointer variable in a single statement using the format shown below.
type *ptr_var1 = init_expr1, *ptr_var2 = init_expr2, … ;
It is also possible to mix the declaration and initialization of ordinary variables and pointers. However, we should avoid it to maintain program readability.
Example of Pointer Assignment and Initialization
char a= ‘A’;
char *pa = &a;
printf(“The address of character variable a: %p\n”, pa);
printf(“The address of pointer variable pa : %p\n”, &pa);
printf(“The value pointed by pointer variable pa: %c\n”, *pa);
Here, pa is a character pointer variable that is initialized with the address of character variable a defined in the first line. Thus, pa points to variable a. The first two printf statements print the address of variables a and pa using the %p (p for pointer) conversion. The last printf statement prints the value of a using the pointer variable pa. When the program containing this code is executed in Code::Blocks, the output is displayed as shown below. ·
The address of character variable a: 0022FF1F
The address of pointer variable pa : 0022FF18
The value pointed by pointer variable pa: A
Note that the addresses displayed in the output will usually be different depending on other variables declared in the program and the compiler/IDE used.
Another example is given below in which pointers are initialized with the addresses of variables of incompatible type.
char c = ‘Z’;
int i = 10;
float f = 1.1;
char *pcl = &i, *pc2 = &f;
int *pil = &c, *pi2 = &f;
float *pfl = &c, *pf2 = &i;
printf(“Character: %c %c\n”, *pcl, *pc2);
printf(“Integer : %d %d\n”, *pil, *pi2);
printf (“Float : %f %f\n”, =pfl, *pf2);
Note that the character pointer variables pcl and pc2 are initialized with the addresses of the int and float variables, respectively. Similarly, the int and float pointer variables are also initialized with addresses of variables of incompatible type. When the program containing this code is compiled in Code::Blocks, the compiler reports six warning messages (initialization from incompatible pointer type), one for each incompatible pointer initialization.
It is not a good idea to ignore such warnings associated with pointers. Although, the program executes in the presence of these warnings, it displays wrong results as shown below.
Integer : 90 1066192077
Float : 0.000000 0.000000
You’ll also like:
- Write A C++ Program To Signify Importance Of Assignment (=) And Shorthand Assignment (+=) Operator.
- Write C++ Example to illustrate two dimensional array implemented as pointer to a pointer.
- Two-Dimensional Arrays Using a Pointer to Pointer
- Declaration and Initialization of Pointers in C
- Initialization of Two Dimensional Arrays Java
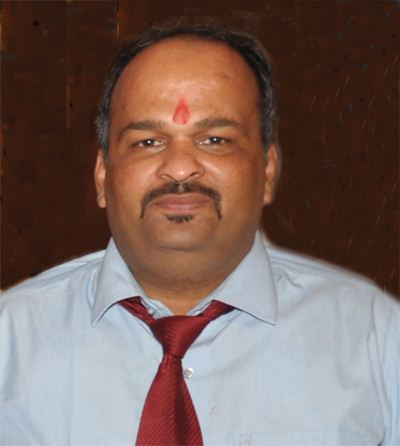
Dinesh Thakur is a Freelance Writer who helps different clients from all over the globe. Dinesh has written over 500+ blogs, 30+ eBooks, and 10000+ Posts for all types of clients.
For any type of query or something that you think is missing, please feel free to Contact us .
Basic Course
- Database System
- Management System
- Electronic Commerce
Programming
- Structured Query (SQL)
- Java Servlet
World Wide Web
- Java Script
- HTML Language
- Cascading Style Sheet
- Java Server Pages
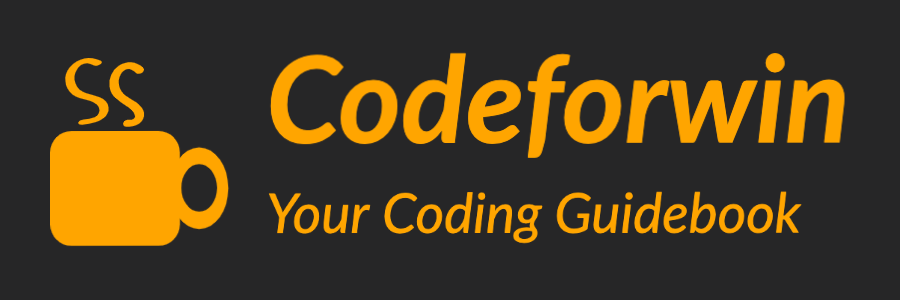
Pointer programming exercises and solutions in C
Pointer is a variable that stores memory addresses. Unlike normal variables it does not store user given or processed value, instead it stores valid computer memory address.
Pointer allows various magical things to be performed in C.
- Pointers are more efficient in handling arrays and structures.
- Pointers are used to return multiple values from a function.
- Pointer allows dynamic memory allocation and deallocation (creation and deletion of variables at runtime) in C. Which undoubtedly is the biggest advantage of pointers.
- Pointer allows to refer and pass a function as a parameter to functions.
and many more…
For beginners pointers can be a bad dream if not practiced well. However, once mastered you can do anything you want to do in C programming language.
In this exercise I will cover most of the pointer related topics from a beginner level. Always feel free to drop your queries and suggestion down below in the comments section .
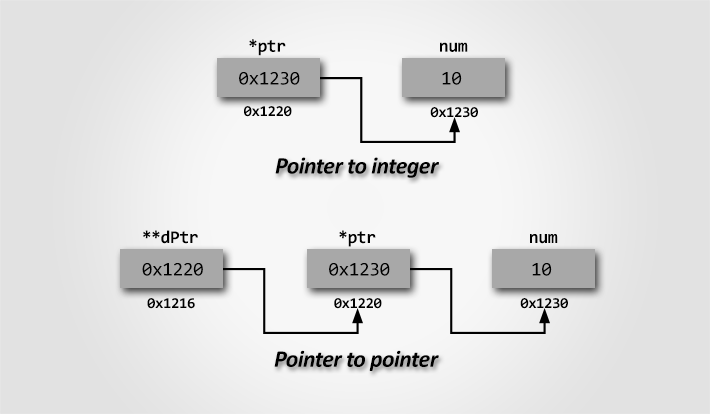
Required knowledge
Pointers , Pointer Arithmetic , Pointer to Pointer , Pointer and Arrays , Function Pointer
Please go through above tutorials to get a good grasp of following examples.
List of pointer programming exercises
- Write a C program to create, initialize and use pointers .
- Write a C program to add two numbers using pointers .
- Write a C program to swap two numbers using pointers .
- Write a C program to input and print array elements using pointer .
- Write a C program to copy one array to another using pointers .
- Write a C program to swap two arrays using pointers .
- Write a C program to reverse an array using pointers .
- Write a C program to search an element in array using pointers .
- Write a C program to access two dimensional array using pointers .
- Write a C program to add two matrix using pointers .
- Write a C program to multiply two matrix using pointers .
- Write a C program to find length of string using pointers .
- Write a C program to copy one string to another using pointers .
- Write a C program to concatenate two strings using pointers .
- Write a C program to compare two strings using pointers .
- Write a C program to find reverse of a string using pointers .
- Write a C program to sort array using pointers .
- Write a C program to return multiple value from function using pointers .
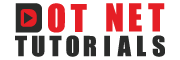
Character Pointer in C
Back to: C Tutorials For Beginners and Professionals
Character Pointer in C Language with Examples
In this article, I will discuss Character Pointers in C Language with Examples. Please read our previous articles discussing Passing Pointers to Function in C Language with Examples. At the end of this article, you will understand what Character Pointer is, why we need Character Pointers, and how to create Character Pointers in C Language.
Character Pointer in C Language:
In C programming, a character pointer points to a character data type. This type of pointer is commonly used for dealing with strings (arrays of characters) because, in C, strings are represented as arrays of characters terminated by a null character (‘\0’). Understanding character pointers is crucial for effective C string manipulation and memory management.
Key Points:
- Character pointers are essential for string manipulation in C.
- Be cautious with string literals, as they are read-only. Attempting to modify a string literal via a pointer will cause undefined behavior.
- You can use character pointers to traverse and manipulate strings.
- Understanding the difference between a string literal and a character array is crucial when working with character pointers.
Let’s see multiple examples to understand how character pointers are used in various scenarios.
Example: Pointing to a Single Character
In this example, char_ptr is a pointer to a character variable ch.
Example: Pointing to a String Literal Using Character in C
Here, str is a pointer to the first character of the string literal “Hello, world!”. Remember, string literals are immutable, so attempting to modify them (like str[0] = ‘h’;) leads to undefined behavior.
Example: Traversing a String with a Pointer
In this example, ptr traverses and prints each character in the string str.
Example 4: Modifying a String Array Using Pointers
Since str is an array, it can be modified. The pointer ptr is used to change the second character of str.
Example: Function to Calculate String Length Using Character Pointers
This function calculates the length of a string by using a character pointer to traverse the string until the null character \0 is encountered.
Example: Dynamic Memory Allocation
Character pointers are often used with dynamic memory allocation for strings:
In this example, str is a pointer to a dynamically allocated block of memory, large enough to hold a string of 49 characters plus the null terminator.
Points to Remember:
- When using character pointers, especially with dynamic memory, it’s crucial to ensure you don’t access memory outside the allocated range. This can lead to undefined behavior.
- Ensure that strings are null-terminated, as many string-handling functions in C rely on this.
- Read-Only vs. Modifiable: Understand the difference between pointing to a string literal (read-only) and pointing to a character array (modifiable).
- Memory Management: Be cautious with dynamic memory allocation and ensure proper memory deallocation to avoid leaks.
- When using dynamically allocated memory, make sure to free the allocated memory using free() to avoid memory leaks.
- Safety and Stability: Incorrect use of character pointers can lead to errors like segmentation faults, especially when dealing with uninitialized pointers, null pointers, or going beyond the bounds of the string.
When to Use Character Pointer in C?
Using a character pointer in C is particularly beneficial in several scenarios. Understanding when and how to use character pointers is crucial for efficient and effective C programming, especially in string manipulation and memory management. Here are some common situations where you would use a character pointer:
- Handling String Literals: You often use character pointers when working with string literals. For example, char *str = “Hello World”; creates a pointer str that points to the first character of the string literal. Remember, string literals are stored in read-only memory, so they should not be modified.
- Dynamic Strings: If you need to create strings whose size is not known at compile time or that can change during program execution, you use character pointers with dynamic memory allocation functions like malloc and realloc.
- Passing Strings to Functions: It’s more efficient to pass a pointer to a character array (string) to a function rather than passing the whole array. This way, the function can work with the original string without creating a copy.
- Modifying Strings in Functions: If you need to modify a string in a function, you pass a character pointer to the function. This allows the function to modify the original string.
- Memory Efficiency: Using character pointers instead of character arrays can save memory, especially when dealing with large strings or a large number of strings, as pointers are usually smaller in size than arrays.
- Handling Arrays of Strings: When dealing with arrays of strings (e.g., command-line arguments), character pointers are used to create arrays of pointers, with each pointer pointing to a different string.
- Reading and Writing Strings: In operations involving I/O, like reading from or writing to files, network sockets, etc., character pointers are used to point to buffers (character arrays) where the data is read into or written from.
- Defining String Constants: For defining constants that won’t change during the execution of the program, character pointers to string literals are often used.
In the next article, I will discuss Pointer to Constant in C Language with Examples. In this article, I try to explain the Character Pointer in C Language with Examples . I hope you enjoy this article. I would like to have your feedback. Please post your feedback, questions, or comments about this article.
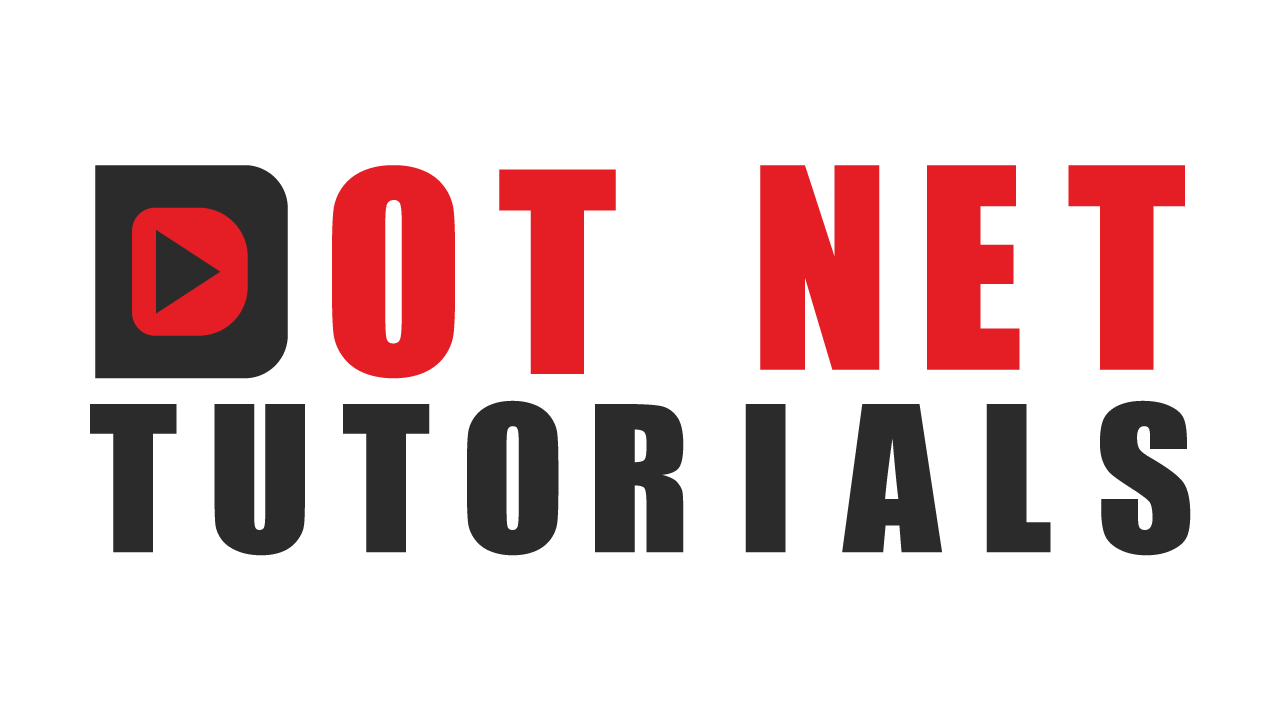
About the Author: Pranaya Rout
Pranaya Rout has published more than 3,000 articles in his 11-year career. Pranaya Rout has very good experience with Microsoft Technologies, Including C#, VB, ASP.NET MVC, ASP.NET Web API, EF, EF Core, ADO.NET, LINQ, SQL Server, MYSQL, Oracle, ASP.NET Core, Cloud Computing, Microservices, Design Patterns and still learning new technologies.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, c introduction.
- Getting Started with C
- Your First C Program
C Fundamentals
- C Variables, Constants and Literals
- C Data Types
C Input Output (I/O)
- C Programming Operators
C Flow Control
- C if...else Statement
- C while and do...while Loop
- C break and continue
- C switch Statement
- C goto Statement
- C Functions
- C User-defined functions
- Types of User-defined Functions in C Programming
- C Recursion
- C Storage Class
C Programming Arrays
- C Multidimensional Arrays
- Pass arrays to a function in C
C Programming Pointers
Relationship Between Arrays and Pointers
- C Pass Addresses and Pointers
- C Dynamic Memory Allocation
- C Array and Pointer Examples
C Programming Strings
String Manipulations In C Programming Using Library Functions
- String Examples in C Programming
C Structure and Union
- C structs and Pointers
- C Structure and Function
C Programming Files
- C File Handling
C Files Examples
C Additional Topics
- C Keywords and Identifiers
- C Precedence And Associativity Of Operators
- C Bitwise Operators
- C Preprocessor and Macros
- C Standard Library Functions
C Tutorials
- Find the Frequency of Characters in a String
- Sort Elements in Lexicographical Order (Dictionary Order)
- Remove all Characters in a String Except Alphabets
In C programming, a string is a sequence of characters terminated with a null character \0 . For example:
When the compiler encounters a sequence of characters enclosed in the double quotation marks, it appends a null character \0 at the end by default.

- How to declare a string?
Here's how you can declare strings:
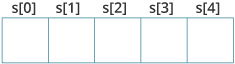
Here, we have declared a string of 5 characters.
- How to initialize strings?
You can initialize strings in a number of ways.
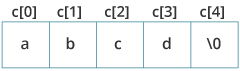
Let's take another example:
Here, we are trying to assign 6 characters (the last character is '\0' ) to a char array having 5 characters. This is bad and you should never do this.
Assigning Values to Strings
Arrays and strings are second-class citizens in C; they do not support the assignment operator once it is declared. For example,
Note: Use the strcpy() function to copy the string instead.
Read String from the user
You can use the scanf() function to read a string.
The scanf() function reads the sequence of characters until it encounters whitespace (space, newline, tab, etc.).
Example 1: scanf() to read a string
Even though Dennis Ritchie was entered in the above program, only "Dennis" was stored in the name string. It's because there was a space after Dennis .
Also notice that we have used the code name instead of &name with scanf() .
This is because name is a char array, and we know that array names decay to pointers in C.
Thus, the name in scanf() already points to the address of the first element in the string, which is why we don't need to use & .
How to read a line of text?
You can use the fgets() function to read a line of string. And, you can use puts() to display the string.
Example 2: fgets() and puts()
Here, we have used fgets() function to read a string from the user.
fgets(name, sizeof(name), stdlin); // read string
The sizeof(name) results to 30. Hence, we can take a maximum of 30 characters as input which is the size of the name string.
To print the string, we have used puts(name); .
Note: The gets() function can also be to take input from the user. However, it is removed from the C standard. It's because gets() allows you to input any length of characters. Hence, there might be a buffer overflow.
Passing Strings to Functions
Strings can be passed to a function in a similar way as arrays. Learn more about passing arrays to a function .
Example 3: Passing string to a Function
Strings and pointers.
Similar like arrays, string names are "decayed" to pointers. Hence, you can use pointers to manipulate elements of the string. We recommended you to check C Arrays and Pointers before you check this example.
Example 4: Strings and Pointers
Commonly used string functions.
- strlen() - calculates the length of a string
- strcpy() - copies a string to another
- strcmp() - compares two strings
- strcat() - concatenates two strings
Table of Contents
- Read and Write String: gets() and puts()
- Passing strings to a function
- Strings and pointers
- Commonly used string functions
Video: C Strings
Sorry about that.
Related Tutorials
- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
Assignment Operators in C
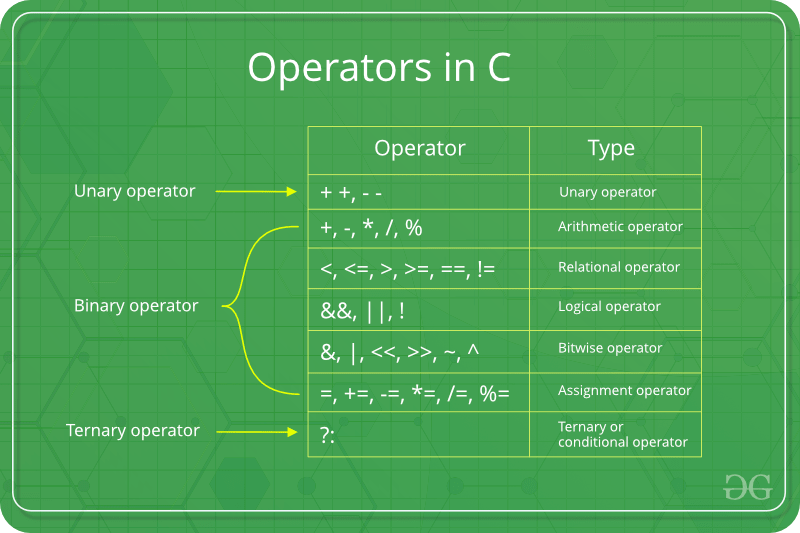
Assignment operators are used for assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error.
Different types of assignment operators are shown below:
1. “=”: This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example:
2. “+=” : This operator is combination of ‘+’ and ‘=’ operators. This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a += 6) = 11.
3. “-=” This operator is combination of ‘-‘ and ‘=’ operators. This operator first subtracts the value on the right from the current value of the variable on left and then assigns the result to the variable on the left. Example:
If initially value stored in a is 8. Then (a -= 6) = 2.
4. “*=” This operator is combination of ‘*’ and ‘=’ operators. This operator first multiplies the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a *= 6) = 30.
5. “/=” This operator is combination of ‘/’ and ‘=’ operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a /= 2) = 3.
Below example illustrates the various Assignment Operators:
Please Login to comment...
Similar reads.
- C-Operators
- cpp-operator
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
How to assign a string to a char pointer?
How to assign a string to a char* (char pointer) in C++?
- 1 char* pw = "some string"; Is this not you are expecting? – Jagannath Commented Feb 10, 2010 at 8:38
- if i use like char* pw = "some string"; i am getting exception. "corruption of heap" – Anuya Commented Feb 10, 2010 at 8:41
- 1 This is one of those "take a step back" questions. You can't really "assign a string" to a char * , because although a char* parameter is sometimes referred to as a "string parameter", it isn't actually a string, it's a pointer (to a string). If you're getting heap corruption, then the problem isn't the assignment, the problem is your management of allocated memory. Most likely there's something vital about allocated memory you don't know. Even if this one line could be replaced with a code snippet that stops your program crashing, the important thing is to understand the whole picture. – Steve Jessop Commented Feb 10, 2010 at 13:42
5 Answers 5
For constant initialization you can simply use
if the string is stored in a variable, and you need to make a copy of the string then you can use strcpy() function

- 4 You should use const char *pw on literals. – Notinlist Commented Feb 10, 2010 at 9:13
- Do I need to free the pw pointer in the first example and if not how does the compiler know when and how to free it. – Borislav Stoilov Commented Sep 9, 2019 at 20:55
- The first examples doesn't use dynamic memory, therefore there is no need to free any allocated buffer. – sergiom Commented Oct 21, 2019 at 11:52
If you just want to assign a string literal to pw , you can do it like char *pw = "Hello world"; .
If you have a C++ std::string object, the value of which you want to assign to pw , you can do it like char *pw = some_string.c_str() . However, the value that pw points to will only be valid for the life time of some_string .
- It's only guranteed to be valid until you perform a mutating operation on some_string . – Bill Commented Feb 10, 2010 at 15:11
If you mean a std::string , you can get a pointer to a C-style string from it, by calling c_str . But the pointer needs to be const .
If pw points to a buffer you've previously allocated, you might instead want to copy the contents of a string into that buffer:
If you're starting with a string literal, it's already a pointer:
Notice the const again - string literals should not be modified, as they are compiled into your program.
But you'll have a better time generally if you use std::string everywhere:
By the way, you need to include the right header:

I think you may want to do this:
But consider doing it another way. Check out http://tiswww.case.edu/php/chet/readline/rltop.html (GNU Readline library). I don't know details about it, just heard about it. Others may have more detailed or other tips for reading passwords from standard input.
If you only want to use it for a single call for something you do not need to copy the contents of someString, you may use someString.c_str() directly if it is required as const char * .
You have to use free on pw some time later,
- 2 strdup is handy but beware of portability issues: stackoverflow.com/questions/482375/c-strdup-function – Manuel Commented Feb 10, 2010 at 8:44
String must be enclosed in double quotes like :
char *pStr = "stackoverflow";
It will store this string literal in the read only memory of the program. And later on modification to it may cause UB.
- if i use like char* pw = "some string"; i am getting exception. "corruption of heap" – karthik 4 secs ago edit [delete this comment] – Anuya Commented Feb 10, 2010 at 8:42
- 3 @Mac - your code works because of C compatibility but it's good practice to use a const char * – Manuel Commented Feb 10, 2010 at 8:51
- Since C++11 this is literally invalid and won't compile. Prior to C++11 it was poor practice & deprecated. – Lightness Races in Orbit Commented May 16, 2018 at 13:29
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c++ or ask your own question .
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Announcing a change to the data-dump process
Hot Network Questions
- Is free will skepticism self-refuting?
- Check if layer has features in map canvas
- Ratio of real world to risk-neutral density
- Can any analytic function be written as the difference of two monotonically increasing analytic functions?
- Square roots and prime numbers
- Schengen Visa from Spain refused
- Is this really a neon lamp?
- Finitely generated group that is not finitely presented
- Can "the" mean "enough"? — E.g.: "She will bake a pie, if she has the ingredients."
- Can I add a PCIe card to a motherboard without rebooting, safely?
- What role can scrum master have/take when product owners differ from opinion?
- If an agreement puts some condition on a file, does the condition also apply to its content? (the content itself is under CC BY-SA)
- In a world where snowfall is "reliably common", how would an injured person with an immobile leg get around?
- Wreath Product and Generalized Symmetric Group
- How will space telescopes catch T Coronae Borealis going nova?
- Can it be predicted if an Interstellar Object will get bound to the solar system by knowing its speed and direction?
- What would a city/arcology designed to survive atomic bombings look like
- Why do tip vortices seem to 'bend' inwards at the tip of a plane wing?
- Assessing model fit in logistic regression with multiple imputation
- What is this switch in the video link below that Bill Jenkins holds down before launching his drag car?
- Does space dust fall on the roof of my house and if so can I detect it with a cheap home microscope?
- In "avoid primitive obsession", what is the meaning of "make the domain model more explicit"?
- Why is there bamboo in 筋?
- Feynman famously criticized the Space Shuttle program for not delivering any value to science. Did things improve in that regard since the 1980s?

COMMENTS
You need to create an int variable somewhere in memory for the int * variable to point at. Your second example does this, but it does other things that aren't relevant here. Here's the simplest thing you need to do: int main(){. int variable; int *ptr = &variable; *ptr = 20; printf("%d", *ptr); return 0;
However, this returns a char. So your assignment. cString1 = strToLower(cString1); has different types on each side of the assignment operator .. you're actually assigning a 'char' (sort of integer) to an array, which resolves to a simple pointer. Due to C++'s implicit conversion rules this works, but the result is rubbish and further access to ...
The type of both the variables is a pointer to char or (char*), so you can pass either of them to a function whose formal argument accepts an array of characters or a character pointer. Here are the differences: arr is an array of 12 characters. When compiler sees the statement: char arr[] = "Hello World";
Assignment of pointers of the same type. C // C program to illustrate Pointer Arithmetic #include <stdio.h> int main {// Declare an array int v [3] ... In C++, cout shows different printing behaviour with character pointers/arrays unlike pointers/arrays of other data types. So this article will firstly explain how cout behaves differently with ...
A character pointer stores the address of a character type or address of the first character of a character array ( string ). Character pointers are very useful when you are working to manipulate the strings. There is no string data type in C. An array of "char" type is considered as a string. Hence, a pointer of a char type array represents a ...
4. Strings. A string is a one-dimensional array of characters terminated by a null(\0).When we write char name[] = "Srijan";, each character occupies one byte of memory with the last one always being \0.. Similar to the arrays we have seen, name and &name[0] points to the 0th character in the string, while &name points to the whole string. Also, name[i] can be written as *(name + i).
Explanation of the program. int* pc, c; Here, a pointer pc and a normal variable c, both of type int, is created. Since pc and c are not initialized at initially, pointer pc points to either no address or a random address. And, variable c has an address but contains random garbage value.; c = 22; This assigns 22 to the variable c.That is, 22 is stored in the memory location of variable c.
This distinctive feature in C empowers programmers to navigate through memory with efficiency. Proficiency in incrementing or decrementing pointers is key to developing optimized and sophisticated code. char firstChar = *ptr; // Dereferencing. char secondChar = *(ptr+1); // Pointer arithmetic.
$ cc c-pointers.c c-pointers.c: In function 'main': c-pointers.c:17:9: warning: assignment from incompatible pointer type [-Wincompatible-pointer-types] chp = &i; ^ c-pointers.c:18:8: warning: assignment from incompatible pointer type [-Wincompatible-pointer-types] ip = &ch; ^ $ ./a.out Enter character: E Enter integer: 43 Enter float: 44.3 ...
Pointer declaration is a fundamental concept in C and C++ programming. It allows the creation of variables that store the address of another variable or function. Learn how to declare, initialize, and use pointers, as well as their syntax, types, and operators. Compare pointers with other C and C++ features, such as arrays, variadic functions, and atomic references.
This is achieved by assigning the address of that variable to the pointer variable, as shown below. int a = 10; int *pa; pa = &a; /* pointer variable pa now points to variable a */. In this example, the first line declares an int variable named a and initializes it to 10. The second line declares a pointer pa of type pointer to int.
Pointer allows various magical things to be performed in C. Pointers are more efficient in handling arrays and structures. Pointers are used to return multiple values from a function. Pointer allows dynamic memory allocation and deallocation (creation and deletion of variables at runtime) in C. Which undoubtedly is the biggest advantage of ...
printf("weight: %f", personPtr->weight); return 0; } Run Code. In this example, the address of person1 is stored in the personPtr pointer using personPtr = &person1;. Now, you can access the members of person1 using the personPtr pointer. By the way, personPtr->age is equivalent to (*personPtr).age. personPtr->weight is equivalent to ...
the expressions "123" and "abc" are converted from "3-element array of char" to "pointer to char", and src will point to the '1' in "123", and des will point to the 'a' in "abc". Again, attempting to modify the contents of a string literal results in undefined behavior, so when you write. des[0] = src[0];
Back to: C Tutorials For Beginners and Professionals Character Pointer in C Language with Examples. In this article, I will discuss Character Pointers in C Language with Examples.Please read our previous articles discussing Passing Pointers to Function in C Language with Examples. At the end of this article, you will understand what Character Pointer is, why we need Character Pointers, and how ...
Structure Pointer in C. A structure pointer is defined as the pointer which points to the address of the memory block that stores a structure known as the structure pointer. Complex data structures like Linked lists, trees, graphs, etc. are created with the help of structure pointers. The structure pointer tells the address of a structure in ...
C Programming Strings. In C programming, a string is a sequence of characters terminated with a null character \0. For example: char c[] = "c string"; When the compiler encounters a sequence of characters enclosed in the double quotation marks, it appends a null character \0 at the end by default. Memory Diagram.
In case 0 you're trying to set x to a character (of type char), but in case 1 you're trying to set x to a C string (of type char const[2]).It's the type of quotes that make a difference; single-quotes are for characters, and double quotes are for C-style strings. If you're meaning to set it to a string both times, put double quotes around the 0 in x = '0'.
1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current value of the variable on left to the value on the right and ...
This is one of those "take a step back" questions. You can't really "assign a string" to a char *, because although a char* parameter is sometimes referred to as a "string parameter", it isn't actually a string, it's a pointer (to a string). If you're getting heap corruption, then the problem isn't the assignment, the problem is your management of allocated memory.