- Java Course
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot

Compound assignment operators in Java
Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand. The following are all possible assignment operator in java:
Implementation of all compound assignment operator
Rules for resolving the Compound assignment operators
At run time, the expression is evaluated in one of two ways.Depending upon the programming conditions:
- First, the left-hand operand is evaluated to produce a variable. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason; the right-hand operand is not evaluated and no assignment occurs.
- Otherwise, the value of the left-hand operand is saved and then the right-hand operand is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason and no assignment occurs.
- Otherwise, the saved value of the left-hand variable and the value of the right-hand operand are used to perform the binary operation indicated by the compound assignment operator. If this operation completes abruptly, then the assignment expression completes abruptly for the same reason and no assignment occurs.
- Otherwise, the result of the binary operation is converted to the type of the left-hand variable, subjected to value set conversion to the appropriate standard value set, and the result of the conversion is stored into the variable.
- First, the array reference sub-expression of the left-hand operand array access expression is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason; the index sub-expression (of the left-hand operand array access expression) and the right-hand operand are not evaluated and no assignment occurs.
- Otherwise, the index sub-expression of the left-hand operand array access expression is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason and the right-hand operand is not evaluated and no assignment occurs.
- Otherwise, if the value of the array reference sub-expression is null, then no assignment occurs and a NullPointerException is thrown.
- Otherwise, the value of the array reference sub-expression indeed refers to an array. If the value of the index sub-expression is less than zero, or greater than or equal to the length of the array, then no assignment occurs and an ArrayIndexOutOfBoundsException is thrown.
- Otherwise, the value of the index sub-expression is used to select a component of the array referred to by the value of the array reference sub-expression. The value of this component is saved and then the right-hand operand is evaluated. If this evaluation completes abruptly, then the assignment expression completes abruptly for the same reason and no assignment occurs.
Examples : Resolving the statements with Compound assignment operators
We all know that whenever we are assigning a bigger value to a smaller data type variable then we have to perform explicit type casting to get the result without any compile-time error. If we did not perform explicit type-casting then we will get compile time error. But in the case of compound assignment operators internally type-casting will be performed automatically, even we are assigning a bigger value to a smaller data-type variable but there may be a chance of loss of data information. The programmer will not responsible to perform explicit type-casting. Let’s see the below example to find the difference between normal assignment operator and compound assignment operator. A compound assignment expression of the form E1 op= E2 is equivalent to E1 = (T) ((E1) op (E2)), where T is the type of E1, except that E1 is evaluated only once.
For example, the following code is correct:
and results in x having the value 7 because it is equivalent to:
Because here 6.6 which is double is automatically converted to short type without explicit type-casting.
Refer: When is the Type-conversion required?
Explanation: In the above example, we are using normal assignment operator. Here we are assigning an int (b+1=20) value to byte variable (i.e. b) that’s results in compile time error. Here we have to do type-casting to get the result.
Explanation: In the above example, we are using compound assignment operator. Here we are assigning an int (b+1=20) value to byte variable (i.e. b) apart from that we get the result as 20 because In compound assignment operator type-casting is automatically done by compile. Here we don’t have to do type-casting to get the result.
Reference: http://docs.oracle.com/javase/specs/jls/se7/html/jls-15.html#jls-15.26.2
Please Login to comment...
Similar reads.
- Java-Operators
- How to Get a Free SSL Certificate
- Best SSL Certificates Provider in India
- Elon Musk's xAI releases Grok-2 AI assistant
- What is OpenAI SearchGPT? How it works and How to Get it?
- Content Improvement League 2024: From Good To A Great Article
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
All Subjects
1.4 Compound Assignment Operators
7 min read • june 18, 2024
user_sophia9212
Avanish Gupta
Compound Assignment Operators
Compound operators.
Sometimes, you will encounter situations where you need to perform the following operation:
This is a bit clunky with the repetition of integerOne in line two. We can condense this with this statement:
The "* = 2" is an example of a compound assignment operator , which multiplies the current value of integerOne by 2 and sets that as the new value of integerOne. Other arithmetic operators also have compound assignment operators as well, with addition, subtraction, division, and modulo having +=, -=, /=, and %=, respectively.
Incrementing and Decrementing
There are special operators for the two following operations in the following snippet well:
These can be replaced with a pre-increment /pre-decrement (++i or - -i) or post-increment /post-decrement (i++ or i- -) operator . You only need to know the post-variant in this course, but it is useful to know the difference between the two. Here is an example demonstrating the difference between them:
By itself, there is no difference between the pre-increment and post-increment operators, but it's evident when you use it in a method such as the println method. For this statement, I will write a debugging output , which happens when we trace the code, which means to follow it line-by-line.
Code Tracing Practice
Now that you’ve learned about code tracing , let’s do some practice! You can use trace tables like the ones shown below to keep track of the values of your variables as they change.
x | y | z | output |
| x | | | --- | --- | | y | | | z | | | output | |
Here are some practice problems that you can use to practice code tracing. Feel free to use whichever method you’re the most comfortable with!
Trace through the following code:
Note: Your answers could look different depending on how you’re tracking your code tracing.
- a *= 3: This line multiplies a by 3 and assigns the result back to a. The value of a is now 18.
- b -= 2: This line subtracts 2 from b and assigns the result back to b. The value of b is now 2.
- c = a % b: This line calculates the remainder of a divided by b and assigns the result to c. The value of c is now 0.
- a += c: This line adds c to a and assigns the result back to a. The value of a is now 18.
- b = a - b: This line subtracts b from a and assigns the result back to b. The value of b is now 16.
- c *= b: This line multiplies c by b and assigns the result back to c. The value of c is now 0. The final values of the variables are:
- c: 0 Trace through the following code:
double x = 15.0;
double y = 4.0;
double z = 0;
- x /= y: This line divides x by y and assigns the result back to x. The value of x is now 3.75.
- y *= x: This line multiplies y by x and assigns the result back to y. The value of y is now 15.0.
- z = y % x: This line calculates the remainder of y divided by x and assigns the result to z. The value of z is now 3.75.
- x += z: This line adds z to x and assigns the result back to x. The value of x is now 7.5.
- y = x / z: This line divides x by z and assigns the result back to y. The value of y is now 2.0.
- z *= y: This line multiplies z by y and assigns the result back to z. The value of z is now 7.5. The final values of the variables are:
- z: 7.5 Trace through the following code:
int a = 100;
int b = 50;
int c = 25;
- a -= b: This line subtracts b from a and assigns the result back to a. The value of a is now 50.
- b *= 2: This line multiplies b by 2 and assigns the result back to b. The value of b is now 100.
- c %= 4: This line calculates the remainder of c divided by 4 and assigns the result back to c. The value of c is now 1.
- a = b + c: This line adds b and c and assigns the result to a. The value of a is now 101.
- b = c - a: This line subtracts a from c and assigns the result to b. The value of b is now -100.
- c = a * b: This line multiplies a and b and assigns the result to c. The value of c is now -10201. The final values of the variables are:
- c: -10201 Trace through the following code:
- a *= 2: This line multiplies a by 2 and assigns the result back to a. The value of a is now 10.
- b -= 1: This line subtracts 1 from b and assigns the result back to b. The value of b is now 2.
- a += c: This line adds c to a and assigns the result back to a. The value of a is now 10.
- b = a - b: This line subtracts b from a and assigns the result back to b. The value of b is now 8.
int y = 10;
int z = 15;
- x *= 2: This line multiplies x by 2 and assigns the result back to x. The value of x is now 10.
- y /= 3: This line divides y by 3 and assigns the result back to y. The value of y is now 3.3333... (rounded down to 3).
- z -= x: This line subtracts x from z and assigns the result back to z. The value of z is now 5.
- x = y + z: This line adds y and z and assigns the result to x. The value of x is now 8.
- y = z - x: This line subtracts x from z and assigns the result to y. The value of y is now -3.
- z = x * y: This line multiplies x and y and assigns the result to z. The value of z is now -24. The final values of the variables are:
- z: -24 Trace through the following code:
double x = 10;
double y = 3;
- x /= y: This line divides x by y and assigns the result back to x. The value of x is now 3.3333... (rounded down to 3.33).
- y *= x: This line multiplies y by x and assigns the result back to y. The value of y is now 10.
- z = y - x: This line subtracts x from y and assigns the result to z. The value of z is now 6.67.
- x += z: This line adds z to x and assigns the result back to x. The value of x is now 10.0.
- y = x / z: This line divides x by z and assigns the result back to y. The value of y is now 1.5.
- z *= y: This line multiplies z by y and assigns the result back to z. The value of z is now 10.0. The final values of the variables are:
- z: 10.0 Want some additional practice? CSAwesome created this really cool Operators Maze game that you can do with a friend for a little extra practice!Â
Key Terms to Review ( 5 )
© 2024 fiveable inc. all rights reserved., ap® and sat® are trademarks registered by the college board, which is not affiliated with, and does not endorse this website..
Compound-Assignment Operators
- Java Programming
- PHP Programming
- Javascript Programming
- Delphi Programming
- C & C++ Programming
- Ruby Programming
- Visual Basic
- M.A., Advanced Information Systems, University of Glasgow
Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand.
Compound-Assignment Operators in Java
Java supports 11 compound-assignment operators:
Example Usage
To assign the result of an addition operation to a variable using the standard syntax:
But use a compound-assignment operator to effect the same outcome with the simpler syntax:
- Java Expressions Introduced
- Understanding the Concatenation of Strings in Java
- Conditional Operators
- Conditional Statements in Java
- Working With Arrays in Java
- Declaring Variables in Java
- If-Then and If-Then-Else Conditional Statements in Java
- How to Use a Constant in Java
- Common Java Runtime Errors
- What Is Java?
- What Is Java Overloading?
- Definition of a Java Method Signature
- What a Java Package Is In Programming
- Java: Inheritance, Superclass, and Subclass
- Understanding Java's Cannot Find Symbol Error Message
- Using Java Naming Conventions
C++ Primer, Fifth Edition by
Get full access to C++ Primer, Fifth Edition and 60K+ other titles, with a free 10-day trial of O'Reilly.
There are also live events, courses curated by job role, and more.
Compound Assignment Operators
We often apply an operator to an object and then assign the result to that same object. As an example, consider the sum program from § 1.4.2 (p. 13 ):
int sum = 0;// sum values from 1 through 10 inclusive for (int val = 1; val <= 10; ++val)Â Â Â Â sum += val; //Â Â equivalent to sum = sum + val
This kind of operation is common not just for addition but for the other arithmetic operators and the bitwise operators, which we cover in § 4.8 (p. 152 ). There are compound assignments for each of these operators:
 +=   -=   *=   /=   %=         // arithmetic operators <<=  >>=   &=   ^=   |=         // bitwise operators; see § 4.8 (p. 152 )
Each compound operator is essentially equivalent to
a = a op b;
with the exception that, when ...
Get C++ Primer, Fifth Edition now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.
Don’t leave empty-handed
Get Mark Richards’s Software Architecture Patterns ebook to better understand how to design components—and how they should interact.
It’s yours, free.
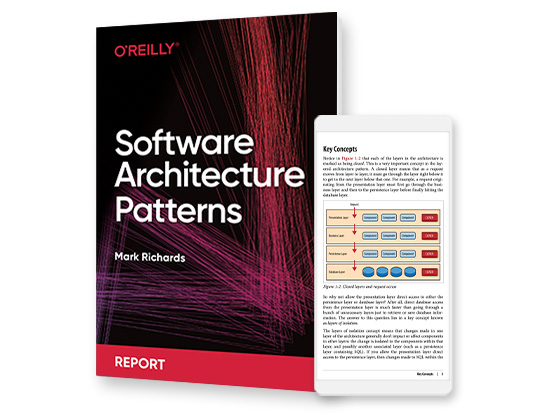
Check it out now on O’Reilly
Dive in for free with a 10-day trial of the O’Reilly learning platform—then explore all the other resources our members count on to build skills and solve problems every day.
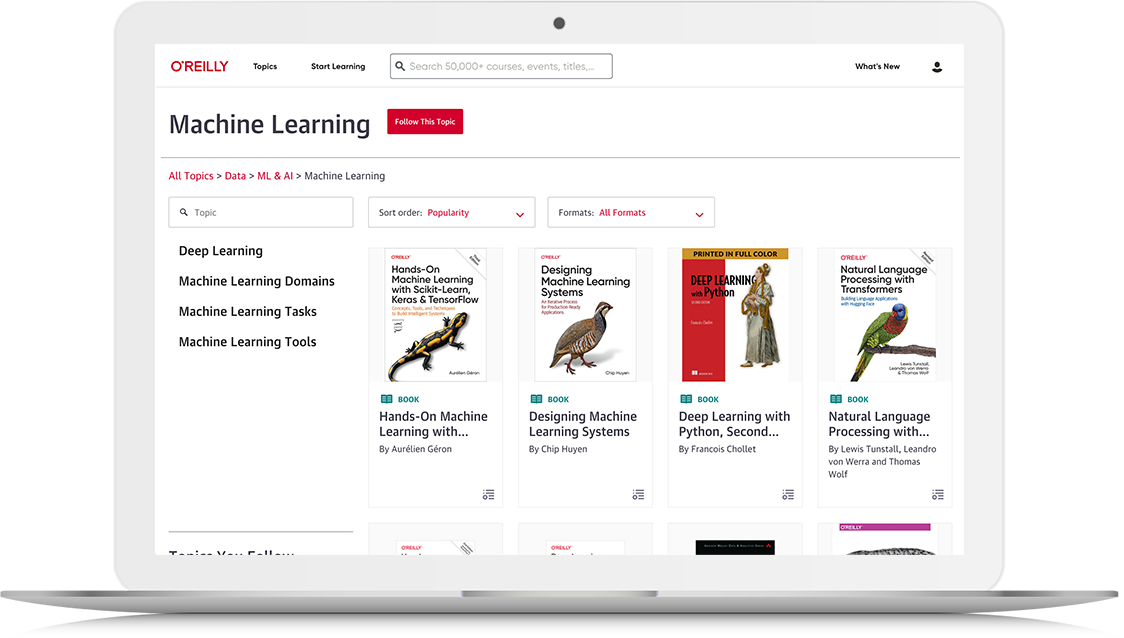
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
C Compound Assignment
- 6 contributors
The compound-assignment operators combine the simple-assignment operator with another binary operator. Compound-assignment operators perform the operation specified by the additional operator, then assign the result to the left operand. For example, a compound-assignment expression such as
expression1 += expression2
can be understood as
expression1 = expression1 + expression2
However, the compound-assignment expression is not equivalent to the expanded version because the compound-assignment expression evaluates expression1 only once, while the expanded version evaluates expression1 twice: in the addition operation and in the assignment operation.
The operands of a compound-assignment operator must be of integral or floating type. Each compound-assignment operator performs the conversions that the corresponding binary operator performs and restricts the types of its operands accordingly. The addition-assignment ( += ) and subtraction-assignment ( -= ) operators can also have a left operand of pointer type, in which case the right-hand operand must be of integral type. The result of a compound-assignment operation has the value and type of the left operand.
In this example, a bitwise-inclusive-AND operation is performed on n and MASK , and the result is assigned to n . The manifest constant MASK is defined with a #define preprocessor directive.
C Assignment Operators
Was this page helpful?
Additional resources
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog

Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Incrementor logic
I'm trying to get deeper with post and pre incrementors but am a bit stuck with the following expression :
I know I'm missing the logic somewhere but where?
What I've tried :
- Going from left to right (though I know it is not recommended)
- Going from the insidest bracket and starting from there.
Thanks for the help
PS : The comments are the details of my calculus
I tried to change de hard coded value from the expression from 2 to something else and the result always gives 0
Look at this example :
This expression should logically be nowhere near 0 but somehow it does print it.
The same happens when I use a negative :
Now, I changed the value of i to begin with :
It gives the double of i each time, whatever the hard coded value is.
- post-increment
- pre-increment

- 1 Ahh didn´t catch that – SomeJavaGuy Commented Oct 14, 2015 at 8:51
- 1 well going from left to right won't solve it.. the compilers convert normal expressions to reverse polish notations and evaluates them and then constructs a expression tree and solves the expression. – Minato Commented Oct 14, 2015 at 8:54
- 1 so simply going from left to right or insidest won't give you the result program will generate – Minato Commented Oct 14, 2015 at 8:56
- 1 @Thomas This is simply curiosity indeed. I want to understand everything I'm learning. – Yassin Hajaj Commented Oct 14, 2015 at 9:23
- 1 @JiriTousek I compiled with 1.4, then ran with following versions, and all produced 0 : 1.4.2_19, 1.5.0_22, 1.6.0_45, 1.7.0_79, 1.8.0_51 on Windows 7, Sun/Oracle JVMs. – Andreas Commented Oct 16, 2015 at 3:53
7 Answers 7
Quoting Java Language Specification, 15.7 Evaluation Order :
The Java programming language guarantees that the operands of operators appear to be evaluated in a specific evaluation order, namely, from left to right . The left-hand operand of a binary operator appears to be fully evaluated before any part of the right-hand operand is evaluated. If the operator is a compound-assignment operator ( §15.26.2 ), then evaluation of the left-hand operand includes both remembering the variable that the left-hand operand denotes and fetching and saving that variable's value for use in the implied binary operation.
So, essentially, i += ++i will remember the old value of i on the left side, before evaluating the right side.
Remember, evaluation order of operands and precedence of operators are two different things.
Showing evaluation order, step by step, with saved value in {braces}:
Followup to edits to question
If we name the initial value I and the constant N :
Then we can see that the values are:
- Hi Andreas ! This seems to be an acceptable answer indeed but would you mind (if it is not too much asked) apply the same logic when i = something else and also when the hard coded value is bigger than 2 ? That would be awesome, I tried to do it but came with a wrong answer – Yassin Hajaj Commented Oct 14, 2015 at 9:54
- 2 @YassinHajaj Just did that. :-) – Andreas Commented Oct 14, 2015 at 9:55
- Thank you very much ! That is great ! – Yassin Hajaj Commented Oct 14, 2015 at 9:55
This is the logic taking into account your first edit (with an unknown X ):
Tricks here:
- += is an assignement operator so it is right-associative: in the snippets, I added parenthesis to express this more clearly
- The result of the assignment expression is the value of the variable after the assignment has occurred
- The postfix increment operator ++ and postfix decrement operator -- add or subtract 1 from the value and the result is stored back into the variable.
- The + additive operator first computes the left-hand operand then the right-hand operand.
For your second edit (with an unknown I added):

- following that logic, could you eleborate why i = i+= (++i + (i+=2)) == i = i+= (++i + (i+=2 + --i)); = 4 – SomeJavaGuy Commented Oct 14, 2015 at 9:18
- I'm editing the question because I came to something else really weird ! – Yassin Hajaj Commented Oct 14, 2015 at 9:19
- Hey @Tunaki, could you check the edit I made to the post. This logic does not apply anymore – Yassin Hajaj Commented Oct 14, 2015 at 9:29
I suggest the following: format the code differently, so that there's only 1 statement per line, e.g.
Then run it under the debugger and press F5 ("Step into") always. This will help you to understand in which order items get evaluated:
- i= : ... (needs to wait for result of calculation A)
- i+= ... (needs to wait B)
- i+= ... (needs to wait C)
- ...: i=3 (result for wait C)
- ++i : i=4 and operand of - is also 4
- ...: i=0 (result for wait B)
- ...: i=0 (result for wait A)
Line 10 will always make the result of line 3 0 , so the initial value of i will never be changed by the whole operation.

Ok, let's break down every thing:
Then starting into the most inner parenthesis:
- it first decrement i and use the result ( -1 )
- then add 2 ( -1+2=1 )
- and add the result to i (which is 0 at the start of the operation) ( 0+1=1=i )
At the end, the first decrement is ignored by the reassignation.
Next parenthesis:
- it increase the i ( with ++i ) at two points
- then the operation becomes (i+1) + previous_result - (i+2) (notice how the increment are not simultaneous) that gives 2 + 1 - 3 = 0 .
- the result of the operation is added to the initial i ( 0 )
Again the increment will get discard by the reassignation.
Which gives 0 :)

I traced the value of i and here are the results:
The value of the 2nd i from the left is not zero, it is the value of i after all the operations to the right are finished.
Because of the highest precedence (...) will be evaluated first then ++ & -- and then remaining operators. Your expression is like
Please fully parenthesize your expression. You will understand evaluation order of expression.
For your EDIT 1
Paranthesis takes the precedence. order would be from inner most to outermost
Each expression evaluates to 0

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged java logic post-increment pre-increment or ask your own question .
- The Overflow Blog
- From PHP to JavaScript to Kubernetes: how one backend engineer evolved over time
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Feedback requested: How do you use tag hover descriptions for curating and do...
Hot Network Questions
- Are automorphisms of matrix algebras necessarily determinant preservers?
- "Authorized ESTA After Incorrectly Answering Criminal Offense Question: What Should I Do?"
- Op Amp Feedback Resistors
- How does one go about writing papers as a nobody?
- Old TV episode about a rich man and his guests trapped in his high-tech underground bunker after a nuclear disaster
- grep command fails with out-of-memory error
- Idiomatic alternative to “going to Canossa”
- Jacobi two square's theorem last step to conclusion
- What is the difference between ‘coming to Jesus’ and ‘believing in Jesus’ in John 6:35
- Sticker on caption phone says that using the captions can be illegal. Why?
- Video game where the hero gets transported to another world with his sister to aid a king in a war
- Can the speed of light inhibit the synchronisation of a power grid?
- Postdoc supervisor has stopped helping
- How do I input box-drawing characters?
- Meaning of capacitor "× 2" symbol on data sheet schematic
- When a submarine blows its ballast and rises, where did the energy for the ascent come from?
- Why if gravity were higher, designing a fully reusable rocket would be impossible?
- How can I address my colleague communicating with us via chatGPT?
- Which game is "that 651"?
- An integral using Mathematica or otherwise
- Name of engineering civil construction device for flattening tarmac
- Can pedestrians and cyclists board shuttle trains in the Channel Tunnel?
- On page 31 of ISL, why is the minimum possible test MSE over all methods (dashed line) 1 instead of another number?
- TeXbook Exercise 21.10 Answer
New Sandbox Program
Click on one of our programs below to get started coding in the sandbox!
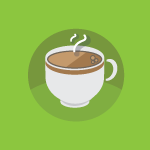
- What is CodeHS?
- Course Catalog
- 6-12 Curriculum Pathway
- All Courses
- Hour of Code
- Assignments
- Classroom Management
- Progress Tracking
- Lesson Plans
- Offline Handouts
- Problem Guides
- Problem Bank
- Playlist Bank
- Quiz Scores
- Integrations
- Google Classroom
- Brightspace (D2L)
- Professional Development
- In-Person PD
- Virtual PD Workshops
- Certification Prep
- Free PD Workshops
- Testimonials
- K-12 Framework
- Common Core Math
- State Standards
- Scope and Sequence
- Connecticut
- Massachusetts
- Mississippi
- New Hampshire
- North Carolina
- North Dakota
- Pennsylvania
- Rhode Island
- South Carolina
- South Dakota
- West Virginia
- Bring to My School
- Homeschools
- Individual Learners
Sign up for a free teacher account to get access to curriculum, teacher tools and teacher resources.
Sign up as a student if you are in a school and have a class code given to you by your teacher.
Interested in teaching with CodeHS? Get in touch, so we can help you bring CodeHS to your school!

- Introduction to Python
- Download and Install Python
- The First Python Program in IDLE
- Data Types in Python
- Python Keywords and Identifiers
- Python Variables and Constants
- Python If Statement
- Python If Else Statement
- Python Elif Statement
- Python Nested If Statement
- Python Nested If Else Statement
- Python While Loop
- Python For Loop
- Python Break Statement
- Python Arithmetic Operators
- Python Compound Assignment Operators
- Python Relational Operators
- Python Logical Operators
- Python Conditional Operator
- Python Classes
- Python Constructors
- Python Static Variable
- Python Static Method
- Python Class Method
- Python Inheritance
- Python Super() Function
- Python Method Overriding
- Python Polymorphism
- Python Garbage Collection
- Python Array from Array Module
- Python Array from Numpy Module
- Python two-dimensional Array
- Python Exception Handling
- Python Exception Propagation
- Python Try, Except Block
- Python Multiple Except Block
- Python Try, Except, Else Block
- Python Finally Block
- Python Raise Keyword
- Python User-Defined Exception
- Python Functions
- Python Functions with Arguments
- Python Functions with Default Arguments
- Python Recursive Function
- Python Local Variables
- Python Global Variables
- Python Tuple
- Python List
- Python Dictionary
- Python - String
- Python - String Methods
- Python - String capitalize() Method
- Python - String upper() Method
- Python - String isupper() Method
- Python - String casefold() Method
- Python - String lower() Method
- Python - String islower() Method
- Python - String swapcase() Method
- Python - String title() Method
- Python - String istitle() Method
- Python - String strip() Method
- Python - String lstrip() Method
- Python - String rstrip() Method
- Python - String isnumeric() Method
- Python - String isdigit() Method
- Python - String isalpha() Method
- Python - String isalnum() Method
- Python - String isdecimal() Method
- Python - String isidentifier() Method
- Python - String isprintable() Method
- Python - String startswith() Method
- Python - String endswith() Method
- Python - String index() Method
- Python - String find() Method
- Python - String rfind() Method
- Python - String rindex() Method
- Python - String center() Method
- Python - String rjust() Method
- Python - String ljust() Method
- Python - String replace() Method
- Python - String count() Method
- Python - String split() Method
- Python - String rsplit() Method
- Python - String splitlines() Method
- Python - String partition() Method
- Python - String rpartition() Method
- Python - String zfill() Method
- Python - String Formatting
- Python - Input/Output Functions
- Python - File and File Modes
- Python - Read a File
- Python - Write a File
- Python - Append to a File
- Python - Modify a File
Advertisement
+= operator
- First, an add operation.
- Next, the assignment of the result of an add operation.
- Statement i+=2 is equal to i=i+2 , hence 2 will be added to the value of i, which gives us 4.
- Finally, the result of addition, 4 is assigned back to i, updating its original value from 2 to 4.
A special case scenario for all the compound assigned operators
Example with += operator, -= operator.
- Subtraction operation.
- Assignment of the result of a subtract operation.
- Statement i-=2 is equal to i=i-2 , hence 2 will be subtracted from the value of i, which gives us 0.
- Finally, the result of subtraction i.e. 0 is assigned back to i, updating its value to 0.
Example with -= operator
*= operator.
- Multiplication operation.
- Assignment of the result of a multiplication operation.
- Statement i*=2 is equal to i=i*2 , hence 2 will be multiplied with the value of i, which gives us 4.
- Finally, the result of multiplication, 4 is assigned back to i, updating its value to 4.
Example with *= operator
/= operator.
- floating-point division operation.
- Assignment of the result of floating-point division operation.
- Statement i/=2 is equal to i=i/2 , hence 4 will be divided by the value of i, which gives us 2.0
- Finally, the result of division i.e. 2.0 is assigned back to i, updating its value from 4 to 2.0.
Example with /= operator
//= operator.
- Integer division operation, which gives us an integer quotient value after dividing two integers and it gives a floating-point quotient after dividing a floating-point number with an integer value or vice versa.
- Assignment of the result of an integer division operation.
- Statement i/=2 is equal to i=i//2 , hence 4 will be divided by the value of i, which gives us 2.
- Finally, the result of division i.e. 2 is assigned back to i, updating its value from 4 to 2.
Example with //= operator
Please share this article -.

Please Subscribe

Notifications
Please check our latest addition C#, PYTHON and DJANGO

- Table of Contents
- Course Home
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- This Chapter
- 1. Getting Started and Primitive Types' data-toggle="tooltip" >

AP CSA Java Course ¶
Welcome to CSAwesome! It’s time to start your journey to learn how to program with Java. A shortcut way to get to this site is to type in the url: course.csawesome.org
CSAwesome is a College Board endorsed curriculum for AP Computer Science A, an introductory college-level computer programming course in Java. If you are a teacher using this curriculum, please join the teaching CSAwesome group which will give you access to teacher resources at csawesome.org .
To make sure the site saves your answers on questions, please click on the person icon at the top to register or login to your Runestone course. As you complete lessons, click the “Mark as completed” button at the bottom. Enjoy the journey!
ATTENTION high school women of color taking AP CSA or CSP: if you identify as female and as Black, Hispanic/Latina, and/or Native American, apply to participate in Sisters Rise Up . The goal of Sisters Rise Up is to help you succeed in your AP Computer Science course and on the exam. They offer one-hour help sessions several times a week and once a month special help sessions often with guest speakers from computing. If you enroll in Sisters Rise Up and send in your AP CS exam score by the end of August, you will be sent a gift card for $100. See the flyer and apply at https://tinyurl.com/55z7tyb9 .
CSAwesome Units:
Table of Contents ¶
- 1.1.1. Preface
- 1.1.2. About the AP CSA Exam
- 1.1.3. Transitioning from AP CSP to AP CSA
- 1.1.4. Java Development Environments
- 1.1.5. Growth Mindset and Pair Programming
- 1.1.6. Pretest for the AP CSA Exam
- 1.1.7. Survey
- 1.2.1. First Java Program
- 1.2.2. Print Methods
- 1.2.3. Syntax Errors and Debugging
- 1.2.4. Reading Error Messages
- 1.2.5. Comments
- 1.2.6. Debugging Challenge
- 1.2.7. Summary
- 1.2.8. AP Practice
- 1.3.1. What is a Variable?
- 1.3.2. Data Types
- 1.3.3. Declaring Variables in Java
- 1.3.4. Naming Variables
- 1.3.5. Debugging Challenge : Weather Report
- 1.3.6. Summary
- 1.3.7. AP Practice
- 1.4.1. Assignment Statements
- 1.4.2. Adding 1 to a Variable
- 1.4.3. Input with Variables
- 1.4.4. Operators
- 1.4.5. The Remainder Operator
- 1.4.6. Programming Challenge : Dog Years
- 1.4.7. Summary
- 1.4.8. AP Practice
- 1.5.1. Code Tracing Challenge and Operators Maze
- 1.5.2. Summary
- 1.6.1. Programming Challenge : Average 3 Numbers
- 1.6.2. Bonus Challenge : Unicode
- 1.6.3. Summary
- 1.7.1. Concept Summary
- 1.7.2. Java Keyword Summary
- 1.7.3. Vocabulary Practice
- 1.7.4. Common Mistakes
- 1.8. Mixed Up Code Practice
- 1.9. Toggle Mixed Up or Write Code Practice
- 1.10. Coding Practice
- 1.11. Multiple Choice Exercises
- 1.12.1. Methods and Procedural Abstraction
- 1.12.2. Method Calls
- 1.12.3. Methods Signature, Parameters, Arguments
- 1.12.4. Overloading
- 1.12.5. Programming Challenge: Song with Parameters
- 1.12.6. Summary
- 1.12.7. AP Practice
- 1.13.1. Non-void Methods
- 1.13.2. Common Errors with Methods
- 1.13.3. Methods Outside the Class
- 1.13.4. Programming Challenge: Ladder on Tower
- 1.13.5. Summary
- 1.13.6. AP Practice
- 2.1.1. What are Objects and Classes?
- 2.1.2. Intro to Objects with Turtles
- 2.1.3. Creating Turtle Objects
- 2.1.4. Programming Challenge : Turtle Drawing
- 2.1.5. Summary
- 2.1.6. AP Practice
- 2.2.1. Overloading Constructors
- 2.2.2. The World Class Constructors
- 2.2.3. The Turtle Class Constructors
- 2.2.4. Object Variables and References
- 2.2.5. Constructor Signatures
- 2.2.6. Formal and Actual Parameters
- 2.2.7. Programming Challenge: Custom Turtles
- 2.2.8. Summary
- 2.2.9. AP Practice
- 2.3.1. Procedural Abstraction
- 2.3.2. Programming Challenge : Draw a Letter
- 2.3.3. Summary
- 2.3.4. AP Practice
- 2.4.1. Tracing Methods
- 2.4.2. Programming Challenge : Turtle House
- 2.4.3. Summary
- 2.4.4. AP Practice
- 2.5.1. Accessors / Getters
- 2.5.2. Methods with Arguments and a Return Value
- 2.5.3. Programming Challenge : Turtle Distances
- 2.5.4. Summary
- 2.5.5. AP Practice
- 2.6.1. String Operators - Concatenation
- 2.6.2. Programming Challenge : Mad Libs
- 2.6.3. Summary
- 2.7.1. String Methods: length, substring, indexOf
- 2.7.2. CompareTo and Equals
- 2.7.3. Common Mistakes with Strings
- 2.7.4. Programming Challenge : Pig Latin
- 2.7.5. Summary
- 2.7.6. String Methods Game
- 2.8.1. Programming Challenge : Debugging
- 2.8.2. Summary
- 2.9.1. Mathematical Functions
- 2.9.2. Random Numbers
- 2.9.3. Programming Challenge : Random Numbers
- 2.9.4. Summary
- 2.10.1. Concept Summary
- 2.10.2. Java Keyword Summary
- 2.10.3. Vocabulary Practice
- 2.10.4. Common Mistakes
- 2.11. Mixed Up Code Practice
- 2.12. Toggle Mixed Up or Write Code Practice
- 2.13. Coding Practice
- 2.14. Practice Test for Objects (2.1-2.5)
- 2.15.1. Easier Multiple Choice Questions
- 2.15.2. Medium Multiple Choice Questions
- 2.15.3. Hard Multiple Choice Questions
- 2.16.1. FRQ Description of Level Class
- 2.16.2. FRQ Practice
- 2.17. Java Swing GUIs (optional)
- 3.1.1. Testing Equality (==)
- 3.1.2. Relational Operators (<, >)
- 3.1.3. Testing with remainder (%)
- 3.1.4. Programming Challenge : Prime Numbers POGIL
- 3.1.5. Summary
- 3.1.6. AP Practice
- 3.1.7. Relational Operators Practice Game
- 3.2.1. Relational Operators in If Statements
- 3.2.2. Common Errors with If Statements
- 3.2.3. Programming Challenge : Magic 8 Ball
- 3.2.4. Summary
- 3.2.5. AP Practice
- 3.3.1. Nested Ifs and Dangling Else
- 3.3.2. Programming Challenge : 20 Questions
- 3.3.3. Summary
- 3.3.4. AP Practice
- 3.4.1. Programming Challenge : Adventure
- 3.4.2. Summary
- 3.4.3. AP Practice
- 3.5.1. And (&&), Or (||), and Not (!)
- 3.5.2. Truth Tables
- 3.5.3. Short Circuit Evaluation
- 3.5.4. Programming Challenge : Truth Tables POGIL
- 3.5.5. Summary
- 3.5.6. AP Practice
- 3.5.7. Boolean Game
- 3.6.1. De Morgan’s Laws
- 3.6.2. Truth Tables
- 3.6.3. Simplifying Boolean Expressions
- 3.6.4. Programming Challenge : Truth Tables POGIL
- 3.6.5. Summary
- 3.6.6. AP Practice
- 3.7.1. String Equality
- 3.7.2. Equality with New Strings
- 3.7.3. Comparing with null
- 3.7.4. Programming Challenge : Tracing Code
- 3.7.5. Summary
- 3.7.6. AP Practice
- 3.8.1. Concept Summary
- 3.8.2. Java Keyword Summary
- 3.8.3. Vocabulary Practice
- 3.8.4. Common Mistakes
- 3.9. Mixed Up Code Practice
- 3.10. Toggle Mixed Up or Write Code Practice
- 3.11. Coding Practice
- 3.12.1. Easier Multiple Choice Questions
- 3.12.2. Medium Multiple Choice Questions
- 3.12.3. Hard Multiple Choice Questions
- 3.13.1. Magpie ChatBot Lab
- 3.13.2. Activity 2: Running Simplified Magpie Code
- 3.13.3. Activity 3: Better Keyword Detection
- 3.13.4. Activity 4: Responses that Transform Statements
- 3.13.5. Mixed Up Code Practice
- 3.14.1. FRQ 1 Part A Description (2022)
- 3.14.2. Warm up Exercises
- 3.14.3. Solve the Problem
- 3.14.4. AP Scoring Rubric
- 3.15. FRQ Style Coding Practice
- 4.1.1. Three Steps to Writing a Loop
- 4.1.2. Tracing Loops
- 4.1.3. Common Errors with Loops
- 4.1.4. Input-Controlled Loops
- 4.1.5. Programming Challenge : Guessing Game
- 4.1.6. Summary
- 4.1.7. AP Practice
- 4.2.1. Three Parts of a For Loop
- 4.2.2. Decrementing Loops
- 4.2.3. Turtle Loops
- 4.2.4. Programming Challenge : Turtles Drawing Shapes
- 4.2.5. Summary
- 4.2.6. AP Practice
- 4.3.1. While Find and Replace Loop
- 4.3.2. For Loops: Reverse String
- 4.3.3. Programming Challenge : String Replacement Cats and Dogs
- 4.3.4. Summary
- 4.4.1. Nested Loops with Turtles
- 4.4.2. Programming Challenge : Turtle Snowflakes
- 4.4.3. Summary
- 4.5.1. Tracing Loops
- 4.5.2. Counting Loop Iterations
- 4.5.3. Programming Challenge : POGIL Analyzing Loops
- 4.5.4. Summary
- 4.5.5. Loop Analysis Game
- 4.6.1. Concept Summary
- 4.6.2. Java Keyword Summary
- 4.6.3. Vocabulary Practice
- 4.6.4. Common Mistakes
- 4.7.1. Going Beyond Remainder 4
- 4.7.2. Look Deeper
- 4.7.3. More Practice
- 4.7.4. Long Division with a Remainder
- 4.7.5. Dividing Evenly
- 4.7.6. Describe the Remainder (%) Operator
- 4.8. Mixed Up Code Practice
- 4.9. Toggle Mixed Up or Write Code Practice
- 4.10. Coding Practice with Loops
- 4.11.1. Easier Multiple Choice Questions
- 4.11.2. Medium Multiple Choice Questions
- 4.11.3. More Practice
- 4.12.1. 2019 APCalendar FRQ
- 4.12.2. Part A: numberOfLeapYear()
- 4.12.3. How to solve numberOfLeapYears()
- 4.12.4. Part B: dayOfWeek()
- 4.13.1. How to solve this problem
- 4.13.2. Video - One way to code the solution
- 4.14.1. How to solve this problem
- 4.14.2. Figuring out the algorithm
- 4.14.3. Write the Code
- 4.14.4. Video - One way to code the solution
- 4.15.1. Activity 0 Analyzing Reviews
- 4.15.2. Activity 1 : Sentiment Value
- 4.15.3. Activity 2 :Total Sentiment Value and Star Ratings
- 4.15.4. Activity 3 : Autogenerate a Fake Review
- 4.15.5. Activity 4 : Create a More Positive or Negative Review
- 4.15.6. Activity 5 : Open-ended Activity
- 5.1.1. Creating a Class
- 5.1.2. Designing a Class
- 5.1.3. Instance Variables
- 5.1.4. Methods
- 5.1.5. Object-Oriented Design
- 5.1.6. Programming Challenge : Riddle Class
- 5.1.7. Design a Class for your Community
- 5.1.8. Summary
- 5.1.9. AP Practice
- 5.2.1. Constructor Signature
- 5.2.2. The Job of a Constructor
- 5.2.3. Advanced AP Topic: Reference parameters
- 5.2.4. Programming Challenge : Student Class
- 5.2.5. Design a Class for your Community
- 5.2.6. Summary
- 5.2.7. AP Practice
- 5.3.1. Comments
- 5.3.2. Preconditions and Postconditions
- 5.3.3. Software Validity and Use-Case Diagrams
- 5.3.4. Agile Software Development
- 5.3.5. Programming Challenge : Comments and Conditions
- 5.3.6. Summary
- 5.4.1. toString
- 5.4.2. Programming Challenge : Class Pet
- 5.4.3. Summary
- 5.4.4. AP Practice
- 5.5.1. How to write a setter
- 5.5.2. Programming Challenge : Class Pet Setters
- 5.5.3. Summary
- 5.5.4. AP Practice
- 5.6.1. Parameters
- 5.6.2. Programming Challenge : Song with Parameters
- 5.6.3. Design a Class for your Community
- 5.6.4. Summary
- 5.6.5. AP Practice
- 5.7.1. Programming Challenge : Static Song and counter
- 5.7.2. Summary
- 5.8.1. Programming Challenge : Debugging
- 5.8.2. Summary
- 5.8.3. AP Practice
- 5.9.1. Programming Challenge : Bank Account
- 5.9.2. Summary
- 5.9.3. AP Practice
- 5.10.1. POGIL Groupwork: Impacts of CS
- 5.10.2. Summary
- 5.11.1. Concept Summary
- 5.11.2. Java Keyword Summary
- 5.11.3. Vocabulary Practice
- 5.11.4. Common Mistakes
- 5.12. Mixed Up Code Practice
- 5.13. Toggle Mixed Up or Write Code Practice
- 5.14. Multiple-Choice Exercises
- 5.15. Midterm Test
- 5.16.1. 2019 StepTracker Class FRQ
- 5.16.2. Determining the Instance Variables
- 5.16.3. Writing the Class Header and Constructor
- 5.16.4. Writing the Accessor Method activeDays
- 5.16.5. Writing the Mutator Method addDailySteps
- 5.16.6. Writing the Accessor Method averageSteps
- 5.17. Free Response Question - Time
- 5.18.1. Try and Solve It
- 5.19. College Board Celebrity and Data Labs
- 5.20.1. Code your Class
- 5.20.2. Optional Swing GUI
- 6.1.1. Declaring and Creating an Array
- 6.1.2. Using new to Create Arrays
- 6.1.3. Initializer Lists to Create Arrays
- 6.1.4. Array length
- 6.1.5. Access and Modify Array Values
- 6.1.6. Programming Challenge : Countries Array
- 6.1.7. Design an Array of Objects for your Community
- 6.1.8. Summary
- 6.1.9. AP Practice
- 6.1.10. Arrays Game
- 6.2.1. Index Variables
- 6.2.2. For Loop to Traverse Arrays
- 6.2.3. Looping From Back to Front
- 6.2.4. Looping through Part of an Array
- 6.2.5. Common Errors When Looping Through an Array
- 6.2.6. Programming Challenge : SpellChecker
- 6.2.7. Design an Array of Objects for your Community
- 6.2.8. Summary
- 6.2.9. Arrays Game
- 6.3.1. Foreach Loop Limitations
- 6.3.2. Foreach Loop Algorithms
- 6.3.3. Programming Challenge : SpellChecker 2
- 6.3.4. Design an Array of Objects for your Community
- 6.3.5. Summary
- 6.4.1. Free Response - Horse Barn A
- 6.4.2. Free Response - Horse Barn B
- 6.4.3. Free Response - Self Divisor B
- 6.4.4. Free Response - Sound A
- 6.4.5. Free Response - Sound B
- 6.4.6. Free Response - Number Cube A
- 6.4.7. Free Response - Number Cube B
- 6.5.1. Concept Summary
- 6.5.2. Java Keyword Summary
- 6.5.3. Vocabulary Practice
- 6.5.4. Common Mistakes
- 6.6. Mixed Up Code Practice
- 6.7. Toggle Mixed Up or Write Code Practice
- 6.8.1. More Practice
- 6.9.1. Easier Multiple Choice Questions
- 6.9.2. Medium Multiple Choice Questions
- 6.9.3. Hard Multiple Choice Questions
- 6.10. Practice Exam for Arrays
- 6.11. More Code Practice with Arrays
- 7.1.1. Packages and imports
- 7.1.2. Declaring and Creating ArrayLists
- 7.1.3. Programming Challenge : FRQ Digits
- 7.1.4. Summary
- 7.2.1. size()
- 7.2.2. add(obj)
- 7.2.3. add(index,obj)
- 7.2.4. remove(index)
- 7.2.5. get(index) and set(index, obj)
- 7.2.6. Comparing arrays and ArrayList s
- 7.2.7. Programming Challenge : Array to ArrayList
- 7.2.8. Summary
- 7.3.1. Enhanced For Each Loop
- 7.3.2. For Loop
- 7.3.3. While Loop
- 7.3.4. ArrayList of Student Objects
- 7.3.5. Programming Challenge : FRQ Word Pairs
- 7.3.6. Summary
- 7.4.1. Free Response - String Scramble B
- 7.4.2. Free Response - Climbing Club A
- 7.4.3. Free Response - Climbing Club B
- 7.4.4. Free Response - Climbing Club C
- 7.4.5. Free Response - CookieOrder A
- 7.4.6. Free Response - CookieOrder B
- 7.4.7. Free Response - StringFormatter A
- 7.4.8. Free Response - StringFormatter B
- 7.4.9. Free Response - Delimiters A
- 7.4.10. Free Response - Delimiters B
- 7.4.11. Free Response - Grid World A
- 7.5.1. Sequential Search
- 7.5.2. Binary Search
- 7.5.3. Runtimes
- 7.5.4. Programming Challenge : Search Runtimes
- 7.5.5. Summary
- 7.6.1. Selection Sort
- 7.6.2. Insertion Sort
- 7.6.3. Programming Challenge : Sort Runtimes
- 7.6.4. Summary
- 7.7.1. POGIL Groupwork: Data Privacy
- 7.7.2. Summary
- 7.8.1. Concept Summary
- 7.8.2. Vocabulary Practice
- 7.8.3. Common Mistakes
- 7.9.1. Java File , Scanner , and IOException Classes
- 7.9.2. Reading in Data with Scanner
- 7.9.3. Reading in Files with java.nio.file
- 7.9.4. Object-Oriented Design with CSV Files
- 7.9.5. Programming Challenge: ArrayList of Objects from Input File
- 7.10. Mixed Up Code Practice
- 7.11. Toggle Mixed Up or Write Code Practice
- 7.12. Code Practice with ArrayLists
- 7.13.1. Easier Multiple Choice Questions
- 7.13.2. Medium Multiple Choice Questions
- 7.13.3. Hard Multiple Choice Questions
- 7.13.4. Easier Search/Sort Multiple Choice Questions
- 7.13.5. Medium Search/Sort Multiple Choice Questions
- 7.13.6. Hard Search/Sort Multiple Choice Questions
- 7.14. College Board Celebrity and Data Labs
- 8.1.1. 2D Arrays (Day 1)
- 8.1.2. Array Storage
- 8.1.3. How Java Stores 2D Arrays
- 8.1.4. Declaring 2D Arrays
- 8.1.5. Set Value(s) in a 2D Array (Day 2)
- 8.1.6. Initializer Lists for 2D Arrays
- 8.1.7. Get a Value from a 2D Array
- 8.1.8. Programming Challenge : ASCII Art
- 8.1.9. Summary
- 8.1.10. 2D Arrays Game
- 8.2.1. Nested Loops for 2D Arrays (Day 1)
- 8.2.2. Getting the Number of Rows and Columns
- 8.2.3. Looping Through a 2D Array
- 8.2.4. AP Practice
- 8.2.5. Enhanced For-Each Loop for 2D Arrays (Day 2)
- 8.2.6. 2D Array Algorithms
- 8.2.7. 2D Array of Objects
- 8.2.8. Programming Challenge : Picture Lab
- 8.2.9. Summary
- 8.2.10. AP Practice
- 8.2.11. 2D Arrays and Loops Game
- 8.3.1. Concept Summary
- 8.3.2. Vocabulary Practice
- 8.3.3. Common Mistakes
- 8.4. Mixed Up Code Practice
- 8.5. Toggle Mixed Up or Write Code Practice
- 8.6. Code Practice with 2D Arrays
- 8.7.1. Free Response - Gray Image A
- 8.7.2. Free Response - Gray Image B
- 8.7.3. Free Response - Route Cipher A
- 8.7.4. Free Response - Route Cipher B
- 8.8.1. Easier Multiple Choice Questions
- 8.8.2. Medium Multiple Choice Questions
- 8.8.3. Hard Multiple Choice Questions
- 8.9.1. Picture Lab A1 - A3
- 8.9.2. Picture Lab A4: 2D Arrays in Java
- 8.9.3. Picture Lab A5: Modifying a Picture
- 8.9.4. Picture Lab A6: Mirroring Pictures
- 8.9.5. Picture Lab A7: Mirroring Part of a Picture
- 8.9.6. Picture Lab A8: Creating a Collage
- 8.9.7. Picture Lab A9: Simple Edge Detection
- 8.10. More Code Practice with 2D Arrays
- 9.1.1. Inheritance (Day 1)
- 9.1.2. Subclass extends Superclass
- 9.1.3. Why Use Inheritance?
- 9.1.4. is-a vs. has-a (Day 2)
- 9.1.5. is-a Substitution Test
- 9.1.6. Programming Challenge : Online Store
- 9.1.7. Summary
- 9.2.1. Chain of initialization
- 9.2.2. Programming Challenge : Square is-a Rectangle
- 9.2.3. Summary
- 9.3.1. Overloading Methods
- 9.3.2. Inherited Get/Set Methods
- 9.3.3. Programming Challenge : Pet Sounds
- 9.3.4. Summary
- 9.4.1. Programming Challenge : Customer Info
- 9.4.2. Summary
- 9.5.1. Superclass References
- 9.5.2. Superclass Method Parameters
- 9.5.3. Superclass Arrays and ArrayLists
- 9.5.4. Programming Challenge : Shopping Cart
- 9.5.5. Summary
- 9.6.1. Programming Challenge : Shopping Cart 2
- 9.6.2. Summary
- 9.7.1. toString() method
- 9.7.2. equals Method
- 9.7.3. Overriding the equals Method
- 9.7.4. Programming Challenge : Savings Account
- 9.7.5. Summary
- 9.8.1. Concept Summary
- 9.8.2. Java Keyword Summary
- 9.8.3. Vocabulary Practice
- 9.8.4. Common Mistakes
- 9.9.1. Free Response - Trio A
- 9.9.2. Trio Student Solution 1
- 9.9.3. Trio Student Solution 2
- 9.9.4. Trio Student Solution 3
- 9.10. Mixed Up Code Practice
- 9.11. Toggle Mixed Up or Write Code Practice
- 9.12. Code Practice with Object Oriented Concepts
- 9.13.1. Easier Multiple Choice Questions
- 9.13.2. Medium Multiple Choice Questions
- 9.13.3. Hard Multiple Choice Questions
- 9.13.4. More Practice
- 9.14. College Board Celebrity Lab
- 10.1.1. What is Recursion? (Day 1)
- 10.1.2. Why use Recursion?
- 10.1.3. Factorial Method
- 10.1.4. Base Case
- 10.1.5. Tracing Recursive Methods (Day 2)
- 10.1.6. Tracing Challenge : Recursion
- 10.1.7. Summary
- 10.2.1. Recursive Binary Search
- 10.2.2. Merge Sort
- 10.2.3. Tracing Challenge : Recursive Search and Sort
- 10.2.4. Summary
- 10.3.1. Concept Summary
- 10.3.2. Vocabulary Practice
- 10.3.3. Common Mistakes
- 10.4. Mixed Up Code Practice
- 10.5. More Mixed Up Code Practice
- 10.6. Toggle Mixed Up or Write Code Practice
- 10.7. Code Practice for Recursion
- 10.8.1. Base Case Practice
- 10.8.2. Easier Multiple Choice Questions
- 10.8.3. Medium Multiple Choice Questions
- 10.8.4. Hard Multiple Choice Questions
- 11.1. Post Test
- 11.2. Post-Survey
- 12.1. Preparing for the AP CSA Exam
- 12.2. Exam 1 for the AP CSA Exam (not timed)
- 12.3. Exam 2 for the AP CSA Exam (not timed)
- 12.4. Exam 3 for the AP CSA Exam (not timed)
- 12.5. Exam 4 for the AP CSA Exam (not timed)
- 12.6. Exam 5 for the AP CSA Exam (not timed)
- 12.7. Exercises
- 13.1. Practice Exam 1 for the AP CSA Exam
- 13.2. Practice Exam 2 for the AP CSA Exam
- 13.3. Practice Exam 3 for the AP CSA Exam
- 13.4. Practice Exam X
- 13.5. AP Bowl 2021 - Part A
- 14.1.1. Mixed Up Code Practice
- 14.1.2. More Mixed Up Code Practice
- 14.2.1. Try and Solve It - Again
- 14.3. RandomStringChooser - Part B Parsons
- 14.4.1. Try and Solve It - Again
- 14.5. Exercises
- 15.1.1. Try and Solve It
- 15.2.1. Try and Solve It
- 15.3.1. Try and Solve It
- 15.4.1. Try and Solve It
- 15.5.1. Try and Solve It
- 15.6.1. Try and Solve It
- 15.7.1. Try and Solve It
- 15.8.1. Try and Solve It
- 15.9.1. Try and Solve It
- 15.10.1. Try and Solve It
- 15.11.1. Try and Solve It
- 15.12.1. Try and Solve It
- 15.13.1. Try and Solve It
- 15.14. Exercises
- 16.1.1. Impostor Syndrome
- 16.1.2. A Lack of Diversity
- 16.2.1. Anaya Taylor
- 16.2.2. Bryan Hickerson
- 16.2.3. Briceida Mariscal
- 16.2.4. Carla De Lira
- 16.2.5. Camille Mbayo
- 16.2.6. Destini Deinde-Smith
- 16.2.7. Eric Espinoza
- 16.2.8. Dr. Gloria Opoku-Boateng
- 16.2.9. Dr. Juan Gilbert
- 16.2.10. Luisa Morales
- 16.2.11. Lucas Vocos
- 16.2.12. Lien Diaz
- 16.2.13. Milly Rodriguez
- 16.2.14. Dr. Nettrice Gaskins
- 17. Hidden Items
Search Page
If you see errors or bugs, please report them with this errors form . If you are a teacher who is interested in CSAwesome PDs or community, please fill out this PD interest form and join the teaching CSAwesome group which will give you access to lesson plans at csawesome.org .
(last revised 7/2024)
© Copyright 2014-2024 Barb Ericson, Univ. Michigan; 2019-2024 Beryl Hoffman, Elms College; 2023-2024 Peter Seibel, Berkeley High School. All rights reserved.
Created using Runestone .

IMAGES
COMMENTS
Study with Quizlet and memorize flashcards containing terms like compound assignment operator, compound assignment operator ex., int varName = 5; varName %= 2; System.out.print(varName); and more.
Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand. The following are all possible assignment operator in java: 1. += (compound addition assignment operator) 2. -= (compound subtraction assignment operator) 3. *= (compound ...
1.4 - Compound Assignment Operators. Get a hint. The statement n = n + 100; is the same as... Click the card to flip 👆. n +=100; Click the card to flip 👆. 1 / 4.
You asked for it, so here it is: more operators! Wait...you didn't ask for more operators? Well, what am I supposed to do with them all?00:00 - Intro00:13 - ...
1.4. Expressions and Assignment Statements ¶ In this lesson, you will learn about assignment statements and expressions that contain math operators and variables.
The "* = 2" is an example of a compound assignment operator, which multiplies the current value of integerOne by 2 and sets that as the new value of integerOne. Other arithmetic operators also have compound assignment operators as well, with addition, subtraction, division, and modulo having +=, -=, /=, and %=, respectively.
Study with Quizlet and memorize flashcards containing terms like Concatenate, Concatenation Operator, Compound Assignment Operator and more.
1.4.1. Assignment Statements 1.4.2. Adding 1 to a Variable 1.4.3. Input with Variables 1.4.4. Operators 1.4.5. The Remainder Operator 1.4.6. Programming Challenge : Dog Years 1.4.7. Summary 1.4.8. AP Practice 1.5. Compound Assignment Operators
In this lesson, you will learn about assignment statements and expressions that contain math operators and variables. 1.4.1. Assignment Statements ¶. Assignment statements initialize or change the value stored in a variable using the assignment operator =. An assignment statement always has a single variable on the left hand side.
1.4: Compound Assignment Operators! AP Computer Science AIn this video, we're going to evaluate what is stored in a variable as a result of an expression wit...
Compound-assignment operators provide a shorter syntax for assigning the result of an arithmetic or bitwise operator. They perform the operation on the two operands before assigning the result to the first operand.
Compound Assignment Operators We often apply an operator to an object and then assign the result to that same object. As an example, consider the sum program from § 1.4.2 … - Selection from C++ Primer, Fifth Edition [Book]
compound operatorsshortcutsincrement ++decrement --https://repl.it/@bzurla/14-Compound-Assignment-Operators
The compound assignment operators consist of a binary operator and the simple assignment operator. They perform the operation of the binary operator on both operands and store the result of that operation into the left operand, which must be a modifiable lvalue.
The compound-assignment operators combine the simple-assignment operator with another binary operator. Compound-assignment operators perform the operation specified by the additional operator, then assign the result to the left operand. For example, a compound-assignment expression such as. expression1 += expression2. can be understood as.
If the operator is a compound-assignment operator ( §15.26.2 ), then evaluation of the left-hand operand includes both remembering the variable that the left-hand operand denotes and fetching and saving that variable's value for use in the implied binary operation.
The expression is usually the right-hand-side (RHS) of the statement, but compound assignment operators are a special shorthand than includes the compounded operation with the left-hand-side (LHS). A simple example is gamma += 1;, which is shorthand for gamma = gamma + 1; In the code given above, the RHS expression is delta / alpha + beta % alpha.
Example 1.3.3 Calculator Example 1.3.4 Temperature Conversion Example 1.3.5 Tricky Java Exercise 1.3.6 Weight of a Pyramid Exercise 1.3.7 Add Fractions Exercise 1.3.8 Freely Falling Bodies 1.4 Compound Assignment Operators Video 1.4.1 Compound Assignment Operators Check for Understanding 1.4.2 Compound Assignment Operators
A special case scenario for all the compound assigned operators. i= 2 ; i+= 2 * 2 ; #equals to, i = i+(2*2); In all the compound assignment operators, the expression on the right side of = is always calculated first and then the compound assignment operator will start its functioning. Hence in the last code, statement i+=2*2; is equal to i=i+ ...
1.5. Compound Assignment Operators. Compound assignment operators are shortcuts that do a math operation and assignment in one step. For example, x += 1 adds 1 to the current value of x and assigns the result back to x. It is the same as x = x + 1 . This pattern is possible with any operator put in front of the = sign, as seen below.
1.13.2 SG1: Decide order of operations. The expression is usually the right-hand-side (RHS) of the statement, but compound assignment operators are a special shorthand than includes the compounded operation with the left-hand-side (LHS). A much simpler example could be gamma += 1, which would be a shorthand for gamma = gamma +1.
1.3.5. Debugging Challenge : Weather Report 1.3.6. Summary 1.3.7. AP Practice 1.4. Expressions and Assignment Statements 1.4.1. Assignment Statements 1.4.2. Adding 1 to a Variable 1.4.3. Input with Variables 1.4.4. Operators 1.4.5. The Remainder Operator 1.4.6. Programming Challenge : Dog Years 1.4.7. Summary 1.4.8. AP Practice 1.5. Compound ...